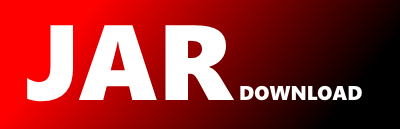
io.snappydata.thrift.ConnectionProperties Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package io.snappydata.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-08-09")
public class ConnectionProperties implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("ConnectionProperties");
private static final org.apache.thrift.protocol.TField CONN_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("connId", org.apache.thrift.protocol.TType.I64, (short)1);
private static final org.apache.thrift.protocol.TField CLIENT_HOST_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("clientHostName", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField CLIENT_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("clientID", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final org.apache.thrift.protocol.TField USER_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("userName", org.apache.thrift.protocol.TType.STRING, (short)4);
private static final org.apache.thrift.protocol.TField TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("token", org.apache.thrift.protocol.TType.STRING, (short)5);
private static final org.apache.thrift.protocol.TField DEFAULT_SCHEMA_FIELD_DESC = new org.apache.thrift.protocol.TField("defaultSchema", org.apache.thrift.protocol.TType.STRING, (short)6);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new ConnectionPropertiesStandardSchemeFactory());
schemes.put(TupleScheme.class, new ConnectionPropertiesTupleSchemeFactory());
}
public long connId; // required
public String clientHostName; // required
public String clientID; // required
public String userName; // optional
public ByteBuffer token; // optional
public String defaultSchema; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
CONN_ID((short)1, "connId"),
CLIENT_HOST_NAME((short)2, "clientHostName"),
CLIENT_ID((short)3, "clientID"),
USER_NAME((short)4, "userName"),
TOKEN((short)5, "token"),
DEFAULT_SCHEMA((short)6, "defaultSchema");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // CONN_ID
return CONN_ID;
case 2: // CLIENT_HOST_NAME
return CLIENT_HOST_NAME;
case 3: // CLIENT_ID
return CLIENT_ID;
case 4: // USER_NAME
return USER_NAME;
case 5: // TOKEN
return TOKEN;
case 6: // DEFAULT_SCHEMA
return DEFAULT_SCHEMA;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __CONNID_ISSET_ID = 0;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.USER_NAME,_Fields.TOKEN,_Fields.DEFAULT_SCHEMA};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.CONN_ID, new org.apache.thrift.meta_data.FieldMetaData("connId", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.CLIENT_HOST_NAME, new org.apache.thrift.meta_data.FieldMetaData("clientHostName", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.CLIENT_ID, new org.apache.thrift.meta_data.FieldMetaData("clientID", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.USER_NAME, new org.apache.thrift.meta_data.FieldMetaData("userName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.TOKEN, new org.apache.thrift.meta_data.FieldMetaData("token", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.DEFAULT_SCHEMA, new org.apache.thrift.meta_data.FieldMetaData("defaultSchema", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(ConnectionProperties.class, metaDataMap);
}
public ConnectionProperties() {
}
public ConnectionProperties(
long connId,
String clientHostName,
String clientID)
{
this();
this.connId = connId;
setConnIdIsSet(true);
this.clientHostName = clientHostName;
this.clientID = clientID;
}
/**
* Performs a deep copy on other.
*/
public ConnectionProperties(ConnectionProperties other) {
__isset_bitfield = other.__isset_bitfield;
this.connId = other.connId;
if (other.isSetClientHostName()) {
this.clientHostName = other.clientHostName;
}
if (other.isSetClientID()) {
this.clientID = other.clientID;
}
if (other.isSetUserName()) {
this.userName = other.userName;
}
if (other.isSetToken()) {
this.token = org.apache.thrift.TBaseHelper.copyBinary(other.token);
}
if (other.isSetDefaultSchema()) {
this.defaultSchema = other.defaultSchema;
}
}
public ConnectionProperties deepCopy() {
return new ConnectionProperties(this);
}
@Override
public void clear() {
setConnIdIsSet(false);
this.connId = 0;
this.clientHostName = null;
this.clientID = null;
this.userName = null;
this.token = null;
this.defaultSchema = null;
}
public long getConnId() {
return this.connId;
}
public ConnectionProperties setConnId(long connId) {
this.connId = connId;
setConnIdIsSet(true);
return this;
}
public void unsetConnId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __CONNID_ISSET_ID);
}
/** Returns true if field connId is set (has been assigned a value) and false otherwise */
public boolean isSetConnId() {
return EncodingUtils.testBit(__isset_bitfield, __CONNID_ISSET_ID);
}
public void setConnIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __CONNID_ISSET_ID, value);
}
public String getClientHostName() {
return this.clientHostName;
}
public ConnectionProperties setClientHostName(String clientHostName) {
this.clientHostName = clientHostName;
return this;
}
public void unsetClientHostName() {
this.clientHostName = null;
}
/** Returns true if field clientHostName is set (has been assigned a value) and false otherwise */
public boolean isSetClientHostName() {
return this.clientHostName != null;
}
public void setClientHostNameIsSet(boolean value) {
if (!value) {
this.clientHostName = null;
}
}
public String getClientID() {
return this.clientID;
}
public ConnectionProperties setClientID(String clientID) {
this.clientID = clientID;
return this;
}
public void unsetClientID() {
this.clientID = null;
}
/** Returns true if field clientID is set (has been assigned a value) and false otherwise */
public boolean isSetClientID() {
return this.clientID != null;
}
public void setClientIDIsSet(boolean value) {
if (!value) {
this.clientID = null;
}
}
public String getUserName() {
return this.userName;
}
public ConnectionProperties setUserName(String userName) {
this.userName = userName;
return this;
}
public void unsetUserName() {
this.userName = null;
}
/** Returns true if field userName is set (has been assigned a value) and false otherwise */
public boolean isSetUserName() {
return this.userName != null;
}
public void setUserNameIsSet(boolean value) {
if (!value) {
this.userName = null;
}
}
public byte[] getToken() {
setToken(org.apache.thrift.TBaseHelper.rightSize(token));
return token == null ? null : token.array();
}
public ByteBuffer bufferForToken() {
return org.apache.thrift.TBaseHelper.copyBinary(token);
}
public ConnectionProperties setToken(byte[] token) {
this.token = token == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(token, token.length));
return this;
}
public ConnectionProperties setToken(ByteBuffer token) {
this.token = org.apache.thrift.TBaseHelper.copyBinary(token);
return this;
}
public void unsetToken() {
this.token = null;
}
/** Returns true if field token is set (has been assigned a value) and false otherwise */
public boolean isSetToken() {
return this.token != null;
}
public void setTokenIsSet(boolean value) {
if (!value) {
this.token = null;
}
}
public String getDefaultSchema() {
return this.defaultSchema;
}
public ConnectionProperties setDefaultSchema(String defaultSchema) {
this.defaultSchema = defaultSchema;
return this;
}
public void unsetDefaultSchema() {
this.defaultSchema = null;
}
/** Returns true if field defaultSchema is set (has been assigned a value) and false otherwise */
public boolean isSetDefaultSchema() {
return this.defaultSchema != null;
}
public void setDefaultSchemaIsSet(boolean value) {
if (!value) {
this.defaultSchema = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case CONN_ID:
if (value == null) {
unsetConnId();
} else {
setConnId((Long)value);
}
break;
case CLIENT_HOST_NAME:
if (value == null) {
unsetClientHostName();
} else {
setClientHostName((String)value);
}
break;
case CLIENT_ID:
if (value == null) {
unsetClientID();
} else {
setClientID((String)value);
}
break;
case USER_NAME:
if (value == null) {
unsetUserName();
} else {
setUserName((String)value);
}
break;
case TOKEN:
if (value == null) {
unsetToken();
} else {
setToken((ByteBuffer)value);
}
break;
case DEFAULT_SCHEMA:
if (value == null) {
unsetDefaultSchema();
} else {
setDefaultSchema((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case CONN_ID:
return getConnId();
case CLIENT_HOST_NAME:
return getClientHostName();
case CLIENT_ID:
return getClientID();
case USER_NAME:
return getUserName();
case TOKEN:
return getToken();
case DEFAULT_SCHEMA:
return getDefaultSchema();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case CONN_ID:
return isSetConnId();
case CLIENT_HOST_NAME:
return isSetClientHostName();
case CLIENT_ID:
return isSetClientID();
case USER_NAME:
return isSetUserName();
case TOKEN:
return isSetToken();
case DEFAULT_SCHEMA:
return isSetDefaultSchema();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof ConnectionProperties)
return this.equals((ConnectionProperties)that);
return false;
}
public boolean equals(ConnectionProperties that) {
if (that == null)
return false;
boolean this_present_connId = true;
boolean that_present_connId = true;
if (this_present_connId || that_present_connId) {
if (!(this_present_connId && that_present_connId))
return false;
if (this.connId != that.connId)
return false;
}
boolean this_present_clientHostName = true && this.isSetClientHostName();
boolean that_present_clientHostName = true && that.isSetClientHostName();
if (this_present_clientHostName || that_present_clientHostName) {
if (!(this_present_clientHostName && that_present_clientHostName))
return false;
if (!this.clientHostName.equals(that.clientHostName))
return false;
}
boolean this_present_clientID = true && this.isSetClientID();
boolean that_present_clientID = true && that.isSetClientID();
if (this_present_clientID || that_present_clientID) {
if (!(this_present_clientID && that_present_clientID))
return false;
if (!this.clientID.equals(that.clientID))
return false;
}
boolean this_present_userName = true && this.isSetUserName();
boolean that_present_userName = true && that.isSetUserName();
if (this_present_userName || that_present_userName) {
if (!(this_present_userName && that_present_userName))
return false;
if (!this.userName.equals(that.userName))
return false;
}
boolean this_present_token = true && this.isSetToken();
boolean that_present_token = true && that.isSetToken();
if (this_present_token || that_present_token) {
if (!(this_present_token && that_present_token))
return false;
if (!this.token.equals(that.token))
return false;
}
boolean this_present_defaultSchema = true && this.isSetDefaultSchema();
boolean that_present_defaultSchema = true && that.isSetDefaultSchema();
if (this_present_defaultSchema || that_present_defaultSchema) {
if (!(this_present_defaultSchema && that_present_defaultSchema))
return false;
if (!this.defaultSchema.equals(that.defaultSchema))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy