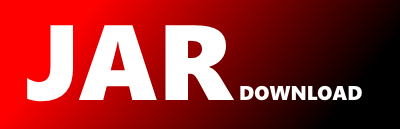
io.soffa.foundation.commons.ObjectUtil Maven / Gradle / Ivy
package io.soffa.foundation.commons;
import com.jsoniter.JsonIterator;
import com.jsoniter.output.JsonStream;
import lombok.SneakyThrows;
import java.nio.charset.StandardCharsets;
import java.util.Optional;
public final class ObjectUtil {
private ObjectUtil() {
}
@SneakyThrows
public static byte[] serialize(Object input) {
if (input == null) {
return null;
}
if (input instanceof byte[]) {
return (byte[]) input;
}
if (input instanceof Optional) {
Optional> opt = (Optional>) input;
return opt.map(ObjectUtil::serialize).orElse(null);
}
return JsonStream.serialize(input).getBytes(StandardCharsets.UTF_8);
}
@SneakyThrows
public static T deserialize(byte[] input, Class type) {
if (input == null || input.length == 0) {
return null;
}
return JsonIterator.deserialize(input, type);
}
@SuppressWarnings("unchecked")
public static T clone(T input) {
return (T) deserialize(serialize(input), input.getClass());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy