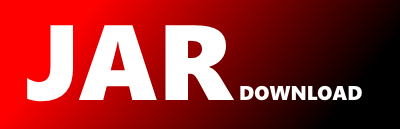
io.soffa.spring.config.SecurityConfig Maven / Gradle / Ivy
package io.soffa.spring.config;
import io.soffa.commons.jwt.JwtDecoder;
import io.soffa.spring.beans.RequestFilter;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter;
import org.springframework.web.cors.CorsConfiguration;
import org.springframework.web.cors.UrlBasedCorsConfigurationSource;
import org.springframework.web.filter.CorsFilter;
import javax.servlet.http.HttpServletResponse;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
private final JwtDecoder jwtDecoder;
public SecurityConfig(@Autowired(required = false) JwtDecoder jwtDecoder) {
this.jwtDecoder = jwtDecoder;
}
@Bean
public CorsFilter corsFilter() {
UrlBasedCorsConfigurationSource source =
new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true);
config.addAllowedOrigin("*");
config.addAllowedHeader("*");
config.addAllowedMethod("*");
source.registerCorsConfiguration("/**", config);
return new CorsFilter(source);
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http = http.cors().and().csrf().disable();
http = http
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and();
http = http
.exceptionHandling()
.authenticationEntryPoint((request, response, ex) -> response.sendError(HttpServletResponse.SC_UNAUTHORIZED, ex.getMessage()))
.and();
http.authorizeRequests().anyRequest().permitAll();
// http.addFilter(new RequestFilter(jwtDecoder));
// Add JWT token filter
http.addFilterBefore(
new RequestFilter(jwtDecoder),
UsernamePasswordAuthenticationFilter.class
);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy