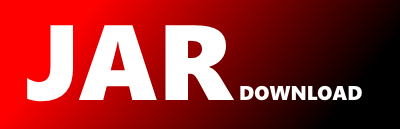
io.sphere.sdk.models.LocalizedString Maven / Gradle / Ivy
package io.sphere.sdk.models;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.google.common.base.Joiner;
import java.util.Optional;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Iterables;
import net.jcip.annotations.Immutable;
import javax.annotation.Nullable;
import java.util.*;
/**
* A wrapper around an attribute which can be translated into a number of locales.
* Note that even if your project only uses one language some attributes (name and description for example) will be
* always be LocalizedString.
*/
@Immutable
public class LocalizedString {
@JsonIgnore
private final Map translations;
@JsonCreator
public LocalizedString(final Map translations) {
this.translations = Optional.ofNullable(translations).orElse(new HashMap<>());
}
/**
* LocalizedString containing the given entry.
* @param locale the locale of the new entry
* @param value the value for the locale
*/
@JsonIgnore
public LocalizedString(final Locale locale, final String value) {
this(ImmutableMap.of(locale, value));
}
/**
* LocalizedString containing the 2 entries.
* @param locale1 the locale for the first entry
* @param value1 the value for the first entry
* @param locale2 the locale for the second entry
* @param value2 the value for the second entry
* @throws IllegalArgumentException if duplicate locales are provided
*/
@JsonIgnore
public LocalizedString(final Locale locale1, final String value1, final Locale locale2, final String value2) {
this(ImmutableMap.of(locale1, value1, locale2, value2));
}
@JsonIgnore
public static LocalizedString of(final Locale locale, final String value) {
return new LocalizedString(locale, value);
}
/**
* LocalizedString containing the given entries.
* @param locale the additional locale of the new entry
* @param value the value for the locale
* @return a LocalizedString containing this data and the from the parameters.
* @throws IllegalArgumentException if duplicate locales are provided
*/
public LocalizedString plus(final Locale locale, final String value) {
final Map newMap = new ImmutableMap.Builder().
putAll(translations).
put(locale, value).
build();
return new LocalizedString(newMap);
}
public Optional get(final Locale locale) {
return Optional.ofNullable(translations.get(locale));
}
public Optional get(final Iterable locales) {
final Locale firstAvailableLocale = Iterables.find(locales, translations::containsKey, null);
return get(firstAvailableLocale);
}
@JsonIgnore
public Set getLocales() {
return translations.keySet();
}
@JsonAnyGetter//@JsonUnwrap supports not maps, but this construct puts map content on top level
private Map getTranslations() {
return ImmutableMap.copyOf(translations);
}
@Override
public String toString() {
return "LocalizedString(" + Joiner.on(", ").withKeyValueSeparator(" -> ").join(translations) + ")";
}
@SuppressWarnings("unused")//used by Jackson JSON mapper
@JsonAnySetter
private void set(final String languageTag, String value) {
translations.put(Locale.forLanguageTag(languageTag), value);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
LocalizedString localized = (LocalizedString) o;
return this.translations.equals(localized.translations);
}
@Override
public int hashCode() {
return translations.hashCode();
}
}