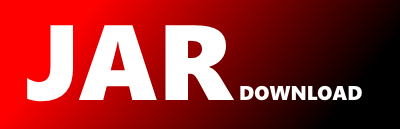
io.sphere.sdk.utils.ListUtils Maven / Gradle / Ivy
package io.sphere.sdk.utils;
import java.util.Optional;
import com.google.common.base.Predicate;
import org.apache.commons.lang3.tuple.Pair;
import java.util.List;
import static com.google.common.collect.Lists.newArrayList;
public final class ListUtils {
private ListUtils() {
}
/**
* Partitions list
in two lists according to predicate
.
* @param list the list which should be divided
* @param predicate returns true if the element of list
should belong to the first result list
* @param generic type of the list
* @return the first list satisfies predicate
, the second one not.
*/
public static Pair, List> partition(final List list, final Predicate predicate) {
final List matchingPredicate = newArrayList();
final List notMatchingPredicate = newArrayList();
for (final T element : list) {
if (predicate.apply(element)) {
matchingPredicate.add(element);
} else {
notMatchingPredicate.add(element);
}
}
return Pair.of(matchingPredicate, notMatchingPredicate);
}
public static Optional headOption(final List list) {
return IterableUtils.headOption(list);
}
}