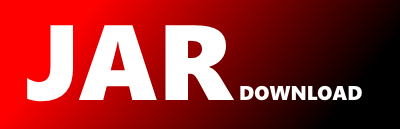
io.sphere.sdk.meta.GettingStarted Maven / Gradle / Ivy
package io.sphere.sdk.meta;
import io.sphere.sdk.models.Base;
/**
About the clients
The client communicates asynchronously with the SPHERE.IO backend via HTTPS.
The client has a method {@code execute} which takes a request as parameter and returns a future of the response type.
There are different clients for different future interfaces:
Clients and future implementations
Client Future implementation
{@link io.sphere.sdk.client.JavaClient} {@code java.util.concurrent.CompletableFuture}
{@link io.sphere.sdk.client.ScalaClient} {@code scala.concurrent.Future}
{@link io.sphere.sdk.client.PlayJavaClient} {@code play.libs.F.Promise}
Preparation
You need to create a project in SPHERE.IO.
Then you need to go to the "Developers" section and open the tab "API clients".
There you find the credentials to access the project with the client.
This is an example for the client credentials page (client secret has been modified):
For this example the contents of an application.conf file could be:
sphere.project="jvm-sdk-dev-1"
sphere.clientId="ELqF0rykXD2fyS8s-IhIPKfQ"
sphere.clientSecret="222222222222222222222222222222226"
Instantiation
Java 8 client
{@include.example example.JavaClientInstantiationExample}
You can use Typesafe Config
for easier configuration management, put "sphere.project", "sphere.clientId", "sphere.clientSecret" into application.conf and the code amount reduces to:
{@include.example example.JavaClientInstantiationExampleWithTypesafeConfig}
Client for Play Framework with Java
{@include.example example.PlayJavaClientInstantiationExample}
For integration tests you can also use directly Typesafe Config to create a client:
{@include.example example.PlayJavaClientIntegrationTestInstantiationExample}
Perform requests
A client works on the abstraction level of one HTTP request for one project.
With one client you can start multiple requests in parallel, it is thread-safe.
The clients have a method {@link io.sphere.sdk.client.PlayJavaClient#execute(io.sphere.sdk.http.ClientRequest)}, which takes a {@link io.sphere.sdk.http.ClientRequest} as parameter.
You can create {@link io.sphere.sdk.http.ClientRequest} yourself or use the given ones.
To find the given ones navigate to {@link io.sphere.sdk.models.DefaultModel} and look for all known subinterfaces,
these should include {@link io.sphere.sdk.products.Product}, {@link io.sphere.sdk.categories.Category},
{@link io.sphere.sdk.taxcategories.TaxCategory} and some more.
The package of these models contain subpackages {@code queries} and {@code commands} which include typical requests like {@link io.sphere.sdk.taxcategories.queries.TaxCategoryQuery}. Example:
{@include.example example.TaxCategoryQueryExample#exampleQuery()}
How to work with asynchronous code
For Play Framework {@code F.Promise}s will be mapped into a {@code Result} or other types as described in the
Play Framework documentation:
{@include.example io.sphere.sdk.queries.QueryDemo#clientShowAsyncProcessing()}
Using design patterns to add functionality to the clients
The clients are interfaces which have a default implementation (add "Impl" to the interface name).
This enables you to use the decorator pattern to configure the cross concern behaviour of the client:
- setup recover mechanisms like returning empty lists or retry the request
- log events
- set timeouts (depending on the future implementation)
- return fake answers for tests
- configure throttling.
The following listing shows a pimped client which updates metrics on responses, retries commands and sets default values:
{@include.example io.sphere.sdk.client.WrappedClientDemo}
Client test doubles for unit tests
Since the clients are interfaces you can implement them to provide test doubles.
Here are some example to provide fake client responses in tests:
{@include.example io.sphere.sdk.client.TestsDemo#withInstanceResults()}
{@include.example io.sphere.sdk.client.TestsDemo#modelInstanceFromJson()}
{@include.example io.sphere.sdk.client.TestsDemo#withJson()}
Conventions
Builders are mutable and use setters like {@link io.sphere.sdk.models.AddressBuilder#streetName(String)} and by calling
them it changes the internal state of the builders.
Methods starting with the prefix {@code with} such as {@link io.sphere.sdk.models.Address#withEmail(String)} will return a new instance which has the same values of the original object (here address) but it has a modified value, in this case another email address.
*/
public final class GettingStarted extends Base {
private GettingStarted() {
}
}