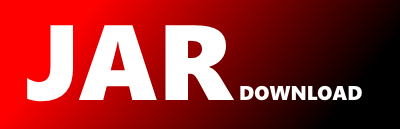
io.sphere.sdk.products.Product Maven / Gradle / Ivy
package io.sphere.sdk.products;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.util.Optional;
import io.sphere.sdk.models.DefaultModel;
import io.sphere.sdk.models.Reference;
/**
A sellable good.
id=operationsOperations:
- Create a product in SPHERE.IO with {@link io.sphere.sdk.products.commands.ProductCreateCommand}.
- Create a product test double with {@link io.sphere.sdk.products.ProductBuilder}.
- Query a product with {@link io.sphere.sdk.products.queries.ProductQuery}.
- Update a product with {@link io.sphere.sdk.products.commands.ProductUpdateCommand}.
- Delete a product with {@link io.sphere.sdk.products.commands.ProductDeleteByIdCommand}.
Consider to use {@link io.sphere.sdk.products.ProductProjection} for queries if you don't need the whole product data so you can safe traffic and memory.
@see io.sphere.sdk.products.ProductProjection
@see io.sphere.sdk.categories.Category
@see io.sphere.sdk.producttypes.ProductType
@see io.sphere.sdk.productdiscounts.ProductDiscount
*/
@JsonDeserialize(as=ProductImpl.class)
public interface Product extends ProductLike, DefaultModel {
ProductCatalogData getMasterData();
public static TypeReference typeReference(){
return new TypeReference() {
@Override
public String toString() {
return "TypeReference";
}
};
}
@Override
default Reference toReference() {
return reference(this);
}
public static String typeId(){
return "product";
}
public static Reference reference(final Product product) {
return Reference.of(typeId(), product.getId(), product);
}
public static Optional> reference(final Optional category) {
return category.map(Product::reference);
}
public static Reference reference(final String id) {
return Reference.of(typeId(), id);
}
default Optional toProjection(final ProductProjectionType productProjectionType) {
return getMasterData().get(productProjectionType)
.map(productData -> new ProductToProductProjectionWrapper(this, productProjectionType));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy