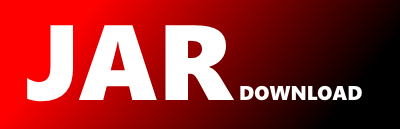
io.sphere.sdk.shippingmethods.queries.GetShippingMethodsByLocation Maven / Gradle / Ivy
package io.sphere.sdk.shippingmethods.queries;
import com.fasterxml.jackson.core.type.TypeReference;
import com.neovisionaries.i18n.CountryCode;
import io.sphere.sdk.client.HttpRequestIntent;
import io.sphere.sdk.client.SphereRequest;
import io.sphere.sdk.client.SphereRequestBase;
import io.sphere.sdk.http.HttpResponse;
import io.sphere.sdk.shippingmethods.ShippingMethod;
import io.sphere.sdk.http.UrlQueryBuilder;
import javax.money.CurrencyUnit;
import java.util.List;
import java.util.Optional;
import java.util.function.Function;
import static io.sphere.sdk.http.HttpMethod.GET;
/**
* Retrieves all the shipping methods that can ship to the given location.
* If the currency parameter is given, then the shipping methods must also have a rate defined in the specified currency.
*
* {@include.example io.sphere.sdk.shippingmethods.queries.GetShippingMethodsByLocationTest#execution()}
*/
public class GetShippingMethodsByLocation extends SphereRequestBase implements SphereRequest> {
private final CountryCode country;
private final Optional state;
private final Optional currency;
private GetShippingMethodsByLocation(final CountryCode country, final Optional state, final Optional currency) {
this.country = country;
this.state = state;
this.currency = currency;
}
@Override
public Function> resultMapper() {
return resultMapperOf(new TypeReference>() {
@Override
public String toString() {
return "TypeReference>";
}
});
}
@Override
public HttpRequestIntent httpRequestIntent() {
return HttpRequestIntent.of(GET, "/shipping-methods" + queryParameters());
}
public static GetShippingMethodsByLocation of(final CountryCode countryCode) {
return new GetShippingMethodsByLocation(countryCode, Optional.empty(), Optional.empty());
}
public GetShippingMethodsByLocation withState(final String state) {
return new GetShippingMethodsByLocation(country, Optional.of(state), currency);
}
public GetShippingMethodsByLocation withCurrency(final CurrencyUnit currency) {
return new GetShippingMethodsByLocation(country, state, Optional.of(currency));
}
private String queryParameters() {
final UrlQueryBuilder urlQueryBuilder = UrlQueryBuilder.of();
urlQueryBuilder.add("country", country.getAlpha2());
state.ifPresent(x -> urlQueryBuilder.add("state", x));
currency.ifPresent(x -> urlQueryBuilder.add("currency", x.getCurrencyCode()));
return urlQueryBuilder.toStringWithOptionalQuestionMark();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy