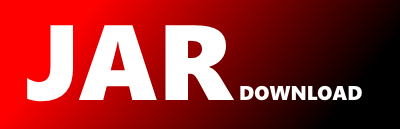
io.sphere.sdk.commands.MetaModelByIdDeleteCommandImpl Maven / Gradle / Ivy
package io.sphere.sdk.commands;
import com.fasterxml.jackson.core.type.TypeReference;
import io.sphere.sdk.client.HttpRequestIntent;
import io.sphere.sdk.client.JsonEndpoint;
import io.sphere.sdk.expansion.ExpansionDslUtil;
import io.sphere.sdk.expansion.ExpansionPath;
import io.sphere.sdk.expansion.MetaModelExpansionDslExpansionModelRead;
import io.sphere.sdk.http.HttpMethod;
import io.sphere.sdk.http.UrlQueryBuilder;
import io.sphere.sdk.models.ResourceView;
import io.sphere.sdk.models.Versioned;
import java.util.Collections;
import java.util.List;
import java.util.function.Function;
import static io.sphere.sdk.utils.ListUtils.listOf;
import static java.util.Objects.requireNonNull;
/**
* Internal base class to implement commands which deletes an entity by ID in SPHERE.IO.
*
* @param the type of the result of the command
*
*/
public abstract class MetaModelByIdDeleteCommandImpl, C, E> extends CommandImpl implements ByIdDeleteCommand, MetaModelExpansionDslExpansionModelRead {
final Versioned versioned;
final JsonEndpoint endpoint;
final E expansionModel;
final List> expansionPaths;
final Function, C> creationFunction;
protected MetaModelByIdDeleteCommandImpl(final Versioned versioned, final JsonEndpoint endpoint, final E expansionModel, final List> expansionPaths, final Function, C> creationFunction) {
this.creationFunction = requireNonNull(creationFunction);
this.expansionModel = requireNonNull(expansionModel);
this.expansionPaths = requireNonNull(expansionPaths);
this.versioned = requireNonNull(versioned);
this.endpoint = requireNonNull(endpoint);
}
protected MetaModelByIdDeleteCommandImpl(final Versioned versioned, final JsonEndpoint endpoint, final E expansionModel, final Function, C> creationFunction) {
this(versioned, endpoint, expansionModel, Collections.emptyList(), creationFunction);
}
protected MetaModelByIdDeleteCommandImpl(final MetaModelByIdDeleteCommandBuilder builder) {
this(builder.versioned, builder.endpoint, builder.expansionModel, builder.expansionPaths, builder.creationFunction);
}
@Override
public HttpRequestIntent httpRequestIntent() {
final String baseEndpointWithoutId = endpoint.endpoint();
if (!baseEndpointWithoutId.startsWith("/")) {
throw new RuntimeException("By convention the paths start with a slash");
}
final UrlQueryBuilder builder = UrlQueryBuilder.of();
expansionPaths().forEach(path -> builder.add("expand", path.toSphereExpand(), true));
final String expansionPathParameters = builder.build();
return HttpRequestIntent.of(HttpMethod.DELETE, baseEndpointWithoutId + "/" + versioned.getId() + "?version=" + versioned.getVersion() + (expansionPathParameters.isEmpty() ? "" : "&" + expansionPathParameters));
}
@Override
protected TypeReference typeReference() {
return endpoint.typeReference();
}
@Override
public List> expansionPaths() {
return expansionPaths;
}
@Override
public final C withExpansionPaths(final List> expansionPaths) {
return copyBuilder().expansionPaths(expansionPaths).build();
}
@Override
public C withExpansionPaths(final ExpansionPath expansionPath) {
return ExpansionDslUtil.withExpansionPaths(this, expansionPath);
}
@Override
public C withExpansionPaths(final Function> m) {
return ExpansionDslUtil.withExpansionPaths(this, m);
}
@Override
public C plusExpansionPaths(final List> expansionPaths) {
return withExpansionPaths(listOf(expansionPaths(), expansionPaths));
}
@Override
public C plusExpansionPaths(final ExpansionPath expansionPath) {
return ExpansionDslUtil.plusExpansionPaths(this, expansionPath);
}
@Override
public C plusExpansionPaths(final Function> m) {
return ExpansionDslUtil.plusExpansionPaths(this, m);
}
@Override
public E expansionModel() {
return expansionModel;
}
protected MetaModelByIdDeleteCommandBuilder copyBuilder() {
return new MetaModelByIdDeleteCommandBuilder<>(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy