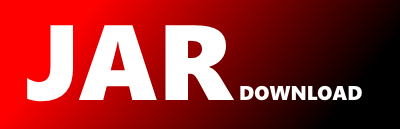
io.sphere.sdk.commands.UpdateCommandDslImpl Maven / Gradle / Ivy
package io.sphere.sdk.commands;
import com.fasterxml.jackson.core.type.TypeReference;
import io.sphere.sdk.client.HttpRequestIntent;
import io.sphere.sdk.client.JsonEndpoint;
import io.sphere.sdk.http.HttpMethod;
import io.sphere.sdk.models.ResourceView;
import io.sphere.sdk.models.Versioned;
import java.util.List;
import java.util.function.Function;
import static io.sphere.sdk.json.SphereJsonUtils.toJsonString;
import static java.util.Objects.requireNonNull;
/**
* Internal base class to implement commands that change one entity in SPHERE.IO.
*
* @param the type of the result of the command, most likely the updated entity without expanded references
*/
public abstract class UpdateCommandDslImpl, C extends UpdateCommandDsl> extends CommandImpl implements UpdateCommandDsl {
private final Versioned versioned;
private final List extends UpdateAction> updateActions;
private final TypeReference typeReference;
private final String baseEndpointWithoutId;
private final Function, C> creationFunction;
private UpdateCommandDslImpl(final Versioned versioned, final List extends UpdateAction> updateActions,
final TypeReference typeReference, final String baseEndpointWithoutId, final Function, C> creationFunction) {
this.creationFunction = requireNonNull(creationFunction);
this.versioned = requireNonNull(versioned);
this.updateActions = requireNonNull(updateActions);
this.typeReference = requireNonNull(typeReference);
this.baseEndpointWithoutId = requireNonNull(baseEndpointWithoutId);
}
protected UpdateCommandDslImpl(final Versioned versioned, final List extends UpdateAction> updateActions,
final JsonEndpoint endpoint, final Function, C> creationFunction) {
this(versioned, updateActions, endpoint.typeReference(), endpoint.endpoint(), creationFunction);
}
protected UpdateCommandDslImpl(final UpdateCommandDslBuilder builder) {
this(builder.getVersioned(), builder.getUpdateActions(), builder.getTypeReference(), builder.getBaseEndpointWithoutId(), builder.getCreationFunction());
}
@Override
protected TypeReference typeReference() {
return typeReference;
}
@Override
public HttpRequestIntent httpRequestIntent() {
if (!baseEndpointWithoutId.startsWith("/")) {
throw new RuntimeException("By convention the paths start with a slash, see baseEndpointWithoutId()");
}
final String path = baseEndpointWithoutId + "/" + getVersioned().getId();
return HttpRequestIntent.of(HttpMethod.POST, path, toJsonString(new UpdateCommandBody<>(getVersioned().getVersion(), getUpdateActions())));
}
@Override
public C withVersion(final Versioned newVersioned) {
return copyBuilder().versioned(newVersioned).build();
}
public Versioned getVersioned() {
return versioned;
}
public List extends UpdateAction> getUpdateActions() {
return updateActions;
}
protected UpdateCommandDslBuilder copyBuilder() {
return new UpdateCommandDslBuilder<>(this);
}
String getBaseEndpointWithoutId() {
return baseEndpointWithoutId;
}
Function, C> getCreationFunction() {
return creationFunction;
}
TypeReference getTypeReference() {
return typeReference;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy