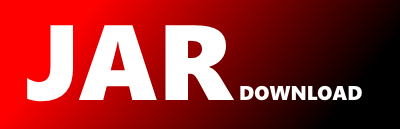
io.sphere.sdk.queries.QueryModelImpl Maven / Gradle / Ivy
package io.sphere.sdk.queries;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.SphereEnumeration;
import javax.annotation.Nullable;
import static io.sphere.sdk.queries.StringQuerySortingModel.normalize;
public class QueryModelImpl extends Base implements QueryModel {
@Nullable
private final QueryModel parent;
@Nullable
private final String pathSegment;
protected QueryModelImpl(final QueryModel parent, final String pathSegment) {
this.parent = parent;
this.pathSegment = pathSegment;
}
@Override
@Nullable
public String getPathSegment() {
return pathSegment;
}
@Nullable
@Override
public QueryModel getParent() {
return parent;
}
protected CurrencyCodeQueryModel currencyCodeModel(final String pathSegment) {
return new CurrencyCodeQueryModelImpl<>(this, pathSegment);
}
protected MoneyQueryModel moneyModel(final String pathSegment) {
return new MoneyQueryModelImpl<>(this, pathSegment);
}
protected AnyReferenceQueryModel anyReferenceModel(final String pathSegment) {
return new AnyReferenceQueryModelImpl <>(this, pathSegment);
}
protected ReferenceQueryModel referenceModel(final String pathSegment) {
return new ReferenceQueryModelImpl<>(this, pathSegment);
}
protected ReferenceOptionalQueryModel referenceOptionalModel(final String pathSegment) {
return new ReferenceOptionalQueryModelImpl<>(this, pathSegment);
}
protected ReferenceCollectionQueryModel referenceCollectionModel(final String pathSegment) {
return new ReferenceCollectionQueryModelImpl<>(this, pathSegment);
}
protected SphereEnumerationQueryModel enumerationQueryModel(final String pathSegment) {
return new SphereEnumerationQueryModelImpl<>(this, pathSegment);
}
protected StringQuerySortingModel stringModel(final String pathSegment) {
return new StringQuerySortingModelImpl<>(this, pathSegment);
}
protected BooleanQueryModel booleanModel(final String pathSegment) {
return new BooleanQueryModelImpl<>(this, pathSegment);
}
protected CountryQueryModel countryQueryModel(final String pathSegment) {
return new CountryQueryModelImpl<>(this, pathSegment);
}
protected LongQuerySortingModel longModel(final String pathSegment) {
return new LongQuerySortingModelImpl<>(this, pathSegment);
}
protected IntegerQuerySortingModel integerModel(final String pathSegment) {
return new IntegerQuerySortingModelImpl<>(this, pathSegment);
}
protected LocalizedStringQuerySortingModel localizedStringQuerySortingModel(final String pathSegment) {
return new LocalizedStringQuerySortingModelImpl<>(this, pathSegment);
}
protected final TimestampSortingModel timestampSortingModel(final String pathSegment) {
return new TimestampSortingModelImpl<>(this, pathSegment);
}
@SuppressWarnings("unchecked")
protected QueryPredicate isPredicate(final V value) {
final V normalizedValue = value instanceof String ? (V) normalize((String) value) : value;
return ComparisonQueryPredicate.ofIsEqualTo(this, normalizedValue);
}
protected QueryPredicate isNotPredicate(final V value) {
return ComparisonQueryPredicate.ofIsNotEqualTo(this, value);
}
protected QueryPredicate isNotPredicate(final String value) {
return ComparisonQueryPredicate.ofIsNotEqualTo(this, normalize(value));
}
protected QueryPredicate isInPredicate(final Iterable args) {
return new IsInQueryPredicate<>(this, args);
}
protected QueryPredicate isNotInPredicate(final Iterable args) {
return new IsNotInQueryPredicate<>(this, args);
}
protected QueryPredicate isGreaterThanPredicate(final V value) {
return ComparisonQueryPredicate.ofIsGreaterThan(this, value);
}
protected QueryPredicate isLessThanPredicate(final V value) {
return ComparisonQueryPredicate.ofIsLessThan(this, value);
}
protected QueryPredicate isLessThanOrEqualToPredicate(final V value) {
return ComparisonQueryPredicate.ofIsLessThanOrEqualTo(this, value);
}
protected QueryPredicate isGreaterThanOrEqualToPredicate(final V value) {
return ComparisonQueryPredicate.ofGreaterThanOrEqualTo(this, value);
}
protected QueryPredicate isPresentPredicate() {
return new OptionalQueryPredicate<>(this, true);
}
protected QueryPredicate isNotPresentPredicate() {
return new OptionalQueryPredicate<>(this, false);
}
protected QueryPredicate embedPredicate(final QueryPredicate embeddedPredicate) {
return new EmbeddedQueryPredicate<>(this, embeddedPredicate);
}
protected QueryPredicate isEmptyCollectionQueryPredicate() {
return new QueryModelQueryPredicate(this){
@Override
protected String render() {
return " is empty";
}
};
}
protected QueryPredicate isNotEmptyCollectionQueryPredicate() {
return new QueryModelQueryPredicate(this){
@Override
protected String render() {
return " is not empty";
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy