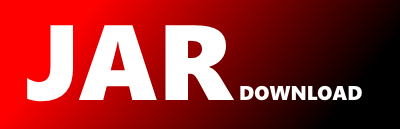
io.sphere.sdk.search.FacetSearchModel Maven / Gradle / Ivy
package io.sphere.sdk.search;
import javax.annotation.Nullable;
import static java.util.Collections.singletonList;
public class FacetSearchModel extends SearchModelImpl {
@Nullable
protected final String alias;
protected final TypeSerializer typeSerializer;
FacetSearchModel(@Nullable final SearchModel parent, final String pathSegment, final TypeSerializer typeSerializer, final String alias) {
super(parent, pathSegment);
this.alias = alias;
this.typeSerializer = typeSerializer;
}
FacetSearchModel(@Nullable final SearchModel parent, final String pathSegment, final TypeSerializer typeSerializer) {
super(parent, pathSegment);
this.alias = null;
this.typeSerializer = typeSerializer;
}
public FacetSearchModel withAlias(final String alias) {
return new FacetSearchModel<>(this, null, typeSerializer, alias);
}
/**
* Generates an expression to obtain the facets of the attribute for all values.
* For example: a possible faceted classification could be ["red": 4, "yellow": 2, "blue": 1].
* @return a facet expression for all values
*/
public TermFacetExpression byAllTerms() {
return new TermFacetExpression<>(this, typeSerializer, alias);
}
/**
* Generates an expression to obtain the facet of the attribute for only the given value.
* For example: a possible faceted classification for "red" could be ["red": 4].
* @param value the value from which to obtain the facet
* @return a facet expression for only the given value
*/
public FilteredFacetExpression byTerm(final V value) {
return byTerm(singletonList(value));
}
/**
* Generates an expression to obtain the facets of the attribute for only the given values.
* For example: a possible faceted classification for ["red", "blue"] could be ["red": 4, "blue": 1].
* @param values the values from which to obtain the facets
* @return a facet expression for only the given values
*/
public FilteredFacetExpression byTerm(final Iterable values) {
return new FilteredFacetExpression<>(this, typeSerializer, values, alias);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy