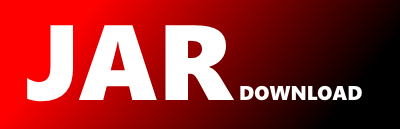
io.sphere.sdk.search.MetaModelSearchDslBuilder Maven / Gradle / Ivy
package io.sphere.sdk.search;
import io.sphere.sdk.expansion.ExpansionPath;
import io.sphere.sdk.http.HttpQueryParameter;
import io.sphere.sdk.http.HttpResponse;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.Builder;
import io.sphere.sdk.models.LocalizedStringEntry;
import javax.annotation.Nullable;
import java.util.List;
import java.util.function.Function;
import static java.util.Collections.emptyList;
/**
*
* @param type of the search result
* @param type of the class implementing this class
* @param type of the search model
* @param type of the expansion model
*/
public class MetaModelSearchDslBuilder, S, E> extends Base implements Builder {
@Nullable
protected LocalizedStringEntry text;
@Nullable
protected Boolean fuzzy;
protected List> facets = emptyList();
protected List> resultFilters = emptyList();
protected List> queryFilters = emptyList();
protected List> facetFilters = emptyList();
protected List> sort = emptyList();
@Nullable
protected Long limit;
@Nullable
protected Long offset;
protected List> expansionPaths = emptyList();
protected List additionalQueryParameters = emptyList();
protected final String endpoint;
protected final Function> resultMapper;
protected final S searchModel;
protected final E expansionModel;
protected final Function, C> searchDslBuilderFunction;
public MetaModelSearchDslBuilder(final String endpoint, final Function> resultMapper,
final S searchModel, final E expansionModel, final Function, C> searchDslBuilderFunction) {
this.endpoint = endpoint;
this.resultMapper = resultMapper;
this.searchModel = searchModel;
this.expansionModel = expansionModel;
this.searchDslBuilderFunction = searchDslBuilderFunction;
}
public MetaModelSearchDslBuilder(final MetaModelSearchDslImpl template) {
this(template.endpoint(), r -> template.deserialize(r), template.getSearchModel(), template.getExpansionModel(), template.searchDslBuilderFunction);
text = template.text();
facets = template.facets();
resultFilters = template.resultFilters();
queryFilters = template.queryFilters();
facetFilters = template.facetFilters();
sort = template.sort();
limit = template.limit();
offset = template.offset();
expansionPaths = template.expansionPaths();
additionalQueryParameters = template.additionalQueryParameters();
fuzzy = template.isFuzzy();
}
public MetaModelSearchDslBuilder text(@Nullable final LocalizedStringEntry text) {
this.text = text;
return this;
}
public MetaModelSearchDslBuilder facets(final List> facets) {
this.facets = facets;
return this;
}
public MetaModelSearchDslBuilder resultFilters(final List> resultFilters) {
this.resultFilters = resultFilters;
return this;
}
public MetaModelSearchDslBuilder queryFilters(final List> queryFilters) {
this.queryFilters = queryFilters;
return this;
}
public MetaModelSearchDslBuilder facetFilters(final List> facetFilters) {
this.facetFilters = facetFilters;
return this;
}
public MetaModelSearchDslBuilder sort(final List> sort) {
this.sort = sort;
return this;
}
public MetaModelSearchDslBuilder limit(@Nullable final Long limit) {
this.limit = limit;
return this;
}
public MetaModelSearchDslBuilder offset(@Nullable final Long offset) {
this.offset = offset;
return this;
}
public MetaModelSearchDslBuilder expansionPaths(final List> expansionPaths) {
this.expansionPaths = expansionPaths;
return this;
}
public MetaModelSearchDslBuilder fuzzy(final Boolean fuzzy) {
this.fuzzy = fuzzy;
return this;
}
@Override
public C build() {
return searchDslBuilderFunction.apply(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy