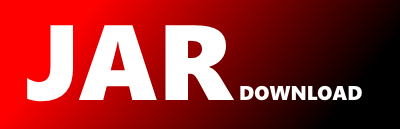
io.sphere.sdk.search.SearchDsl Maven / Gradle / Ivy
package io.sphere.sdk.search;
import io.sphere.sdk.models.LocalizedStringEntry;
import java.util.List;
import java.util.Locale;
public interface SearchDsl> extends EntitySearch {
/**
* Returns an EntitySearch with the new text as search text.
* @param text the new search text
* @return an EntitySearch with text
*/
C withText(final LocalizedStringEntry text);
/**
* Returns an EntitySearch with mofified fuzzyParameter.
* @param fuzzy a flag to indicate if fuzzy search is enabled (true) or not (false)
* @return an EntitySearch with the new fuzzy flag setting
*/
C withFuzzy(final Boolean fuzzy);
C withText(final Locale locale, final String text);
/**
* Returns an EntitySearch with the new facet list as facets.
* @param facets the new facet list
* @return an EntitySearch with facets
*/
C withFacets(final List> facets);
C withFacets(final FacetExpression facet);
/**
* Returns an EntitySearch with the new facet list appended to the existing facets.
* @param facets the new facet list
* @return an EntitySearch with the existing facets plus the new facet list.
*/
C plusFacets(final List> facets);
C plusFacets(final FacetExpression facet);
/**
* Returns an EntitySearch with the new result filter list as result filter.
* @param resultFilters the new result filter list
* @return an EntitySearch with resultFilters
*/
C withResultFilters(final List> resultFilters);
C withResultFilters(final FilterExpression resultFilter);
/**
* Returns an EntitySearch with the new result filter list appended to the existing result filters.
* @param resultFilters the new result filter list
* @return an EntitySearch with the existing result filter plus the new result filter list.
*/
C plusResultFilters(final List> resultFilters);
C plusResultFilters(final FilterExpression resultFilter);
/**
* Returns an EntitySearch with the new query filter list as query filters.
* @param queryFilters the new query filter list
* @return an EntitySearch with queryFilters
*/
C withQueryFilters(final List> queryFilters);
C withQueryFilters(final FilterExpression queryFilter);
/**
* Returns an EntitySearch with the new query filter list appended to the existing query filters.
* @param queryFilters the new query filter list
* @return an EntitySearch with the existing query filters plus the new query filter list.
*/
C plusQueryFilters(final List> queryFilters);
C plusQueryFilters(final FilterExpression queryFilter);
/**
* Returns an EntitySearch with the new facet filter list as facet filter.
* @param facetFilters the new facet filter list
* @return an EntitySearch with facetFilters
*/
C withFacetFilters(final List> facetFilters);
C withFacetFilters(final FilterExpression facetFilter);
/**
* Returns an EntitySearch with the new facet filter list appended to the existing facet filters.
* @param facetFilters the new facet filter list
* @return an EntitySearch with the existing facet filters plus the new facet filter list.
*/
C plusFacetFilters(final List> facetFilters);
C plusFacetFilters(final FilterExpression facetFilter);
/**
* Returns an EntityQuery with the new sort as sort.
* @param sort how the results of the search should be sorted
* @return EntityQuery with sort
*/
C withSort(final SearchSort sort);
//not yet implemented in the SPHERE.IO backend
//
// /**
// * Returns an EntityQuery with the new sort as sort.
// * @param sort list of sorts how the results of the search should be sorted
// * @return EntityQuery with sort
// */
// C withSort(final List> sort);
C withLimit(final long limit);
C withOffset(final long offset);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy