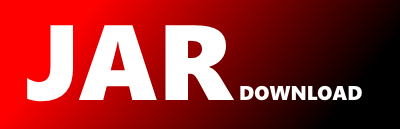
io.sphere.sdk.search.SearchModelExpression Maven / Gradle / Ivy
package io.sphere.sdk.search;
import io.sphere.sdk.models.Base;
import javax.annotation.Nullable;
import java.util.Optional;
import java.util.function.Function;
import static io.sphere.sdk.utils.IterableUtils.toStream;
import static java.util.stream.Collectors.joining;
abstract class SearchModelExpression extends Base {
private final SearchModel searchModel;
private final TypeSerializer typeSerializer;
@Nullable
protected final String alias;
protected SearchModelExpression(final SearchModel searchModel, final TypeSerializer typeSerializer, @Nullable final String alias) {
this.searchModel = searchModel;
this.typeSerializer = typeSerializer;
this.alias = alias;
}
public final String serializeValue(final V value) {
return typeSerializer.getSerializer().apply(value);
}
public final V deserializeValue(final String valueAsString) {
return typeSerializer.getDeserializer().apply(valueAsString);
}
public final String toSearchExpression() {
return attributePath() + serializedValue() + serializedAlias();
}
public final String attributePath() {
return toStream(searchModel.buildPath()).collect(joining("."));
}
public final String resultPath() {
return Optional.ofNullable(alias).orElse(attributePath());
}
protected final String serializedAlias() {
return Optional.ofNullable(alias).map(a -> " as " + a).orElse("");
}
protected abstract String serializedValue();
protected Function serializer() {
return typeSerializer.getSerializer();
}
@Override
public String toString() {
return toSearchExpression();
}
@Override
public final int hashCode() {
return toSearchExpression().hashCode();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy