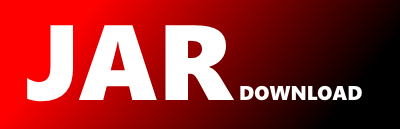
io.sphere.sdk.utils.MapUtils Maven / Gradle / Ivy
package io.sphere.sdk.utils;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.function.Supplier;
import static java.lang.String.format;
public final class MapUtils {
private MapUtils() {
}
public static V getOrThrow(final Map map, final K key, Supplier exceptionSupplier) throws E {
if (map.containsKey(key)) {
return map.get(key);
} else {
throw exceptionSupplier.get();
}
}
public static Map immutableCopyOf(final Map map) {
return Collections.unmodifiableMap(copyOf(map));
}
public static Map copyOf(final Map map) {
final Map copy = new HashMap<>();
copy.putAll(map);
return copy;
}
public static Map mapOf(final K key, final V value) {
final Map result = new HashMap<>();
result.put(key, value);
return result;
}
public static Map mapOf(final K key1, final V value1, final K key2, final V value2) {
if (key1.equals(key2)) {
throw new IllegalArgumentException(format("Duplicate keys (%s) for map creation.", key1));
}
final Map result = new HashMap<>();
result.put(key1, value1);
result.put(key2, value2);
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy