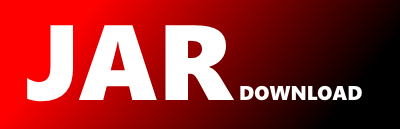
io.sphere.sdk.utils.SphereInternalLogger Maven / Gradle / Ivy
package io.sphere.sdk.utils;
import io.sphere.sdk.http.HttpMethod;
import io.sphere.sdk.http.HttpRequest;
import io.sphere.sdk.http.HttpResponse;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Optional;
import java.util.function.Supplier;
/** Internal logging used by the sphere Java client itself.
*
* Uses slf4j logger named 'sphere' and does not depend on Play logger
* (which also uses slf4j loggers, named 'play' and 'application').
*
* The logger can be configured per Play application using 'logger.sphere' in 'application.conf'.
*
* {@include.example example.LoggingExample}
*
* Logger hierarchy
*
* The loggers form a hierarchy separated by a dot. The root logger is {@code "sphere"} which is implicit set for
* {@link SphereInternalLogger#getLogger(java.lang.String)}, so you never include "sphere" in the logger name.
* The child loggers of sphere are the endpoints, so for example {@code "sphere.categories"} for categories and
* {@code "sphere.product-types"} for product types.
* The grandchild loggers refer to the action. {@code "sphere.categories.requests"} refers to
* performing requests per HTTPS to SPHERE.IO for categories, {@code "sphere.categories.responses"} refers to the responses of SPHERE.IO.
* {@code "sphere.categories.objects"} is for non HTTP API stuff like local object creation.
*
*
* The logger makes use of different log levels, so for example {@code "sphere.categories.responses"} logs on debug level the
* http response from SPHERE.io (abbreviated example):
*
* 10:59:18.623 [ForkJoinPool.commonPool-worker-3] DEBUG sphere.categories.responses - io.sphere.sdk.requests.HttpResponse@39984ae7[statusCode=200,responseBody={"offset":0,"count":4,"total":4,"results":[{"id":"2c41b33e-2d8e-415c-a4b7-f62628fa06e3","version":1,"name":{"en":"Hats"}, [...]
*
* {@code "sphere.categories.responses"} logs on trace level additional the formatted http response from SPHERE.io (abbreviated example):
*
* 10:59:18.657 [ForkJoinPool.commonPool-worker-3] TRACE sphere.categories.responses - 200
{
"offset" : 0,
"count" : 4,
"total" : 4,
"results" : [ {
"id" : "2c41b33e-2d8e-415c-a4b7-f62628fa06e3",
"version" : 1,
"name" : {
"en" : "Hats"
},
"slug" : {
"en" : "hats"
},
"ancestors" : [ ],
"orderHint" : "0.000013999891309781676091866",
"createdAt" : "2014-05-13T13:52:10.978Z",
"lastModifiedAt" : "2014-05-13T13:52:10.978Z"
},
[...]
{@code sphere.products.responses.queries} logs only HTTP GET requests and {@code sphere.products.responses.commands}
logs only HTTP POST/DELETE requests.
Remove the chatty output of the Ning HTTP client
The current Java client uses internally ning and it logs by default a lot, so you need to set the loglevel in your log configuration.
For logback with {@code logback.xml} or {@code logback-test.xml} the setting is {@code }.
*/
public final class SphereInternalLogger {
private final Logger underlyingLogger;
private SphereInternalLogger(final Logger underlyingLogger) {
this.underlyingLogger = underlyingLogger;
}
public SphereInternalLogger debug(final Supplier