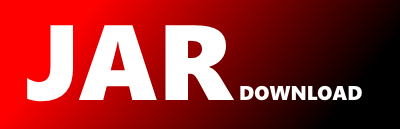
io.sphere.sdk.queries.MetaModelGetDslImpl Maven / Gradle / Ivy
package io.sphere.sdk.queries;
import com.fasterxml.jackson.core.type.TypeReference;
import io.sphere.sdk.client.HttpRequestIntent;
import io.sphere.sdk.client.JsonEndpoint;
import io.sphere.sdk.client.SphereRequestBase;
import io.sphere.sdk.expansion.ExpansionDslUtil;
import io.sphere.sdk.expansion.ExpansionPath;
import io.sphere.sdk.expansion.MetaModelExpansionDslExpansionModelRead;
import io.sphere.sdk.http.HttpMethod;
import io.sphere.sdk.http.HttpQueryParameter;
import io.sphere.sdk.http.HttpResponse;
import io.sphere.sdk.http.UrlQueryBuilder;
import javax.annotation.Nullable;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.function.Function;
import static io.sphere.sdk.http.HttpStatusCode.NOT_FOUND_404;
import static io.sphere.sdk.queries.QueryParameterKeys.EXPAND;
import static io.sphere.sdk.utils.ListUtils.listOf;
import static java.util.Objects.requireNonNull;
/**
* @param result type, maybe directly {@code T} or sth. like {@code List}
* @param type of the query result
* @param type of the class implementing this class
* @param type of the expansion model
*/
public class MetaModelGetDslImpl, E> extends SphereRequestBase implements MetaModelGetDsl, MetaModelExpansionDslExpansionModelRead {
final JsonEndpoint endpoint;
/**
for example an ID, a key, slug, token
*/
final String identifierToSearchFor;
final List> expansionPaths;
final List additionalParameters;
final E expansionModel;
final Function, C> builderFunction;
protected MetaModelGetDslImpl(final JsonEndpoint endpoint, final String identifierToSearchFor, final E expansionModel, final Function, C> builderFunction, final List additionalParameters) {
this(endpoint, identifierToSearchFor, Collections.emptyList(), expansionModel, builderFunction, additionalParameters);
}
protected MetaModelGetDslImpl(final String identifierToSearchFor, final JsonEndpoint endpoint, final E expansionModel, final Function, C> builderFunction) {
this(endpoint, identifierToSearchFor, Collections.emptyList(), expansionModel, builderFunction, Collections.emptyList());
}
protected MetaModelGetDslImpl(final JsonEndpoint endpoint, final String identifierToSearchFor, final E expansionModel, final Function, C> builderFunction) {
this(endpoint, identifierToSearchFor, Collections.emptyList(), expansionModel, builderFunction, Collections.emptyList());
}
protected MetaModelGetDslImpl(final MetaModelGetDslBuilder builder) {
this(builder.endpoint, builder.identifierToSearchFor, builder.expansionPaths, builder.expansionModel, builder.builderFunction, builder.additionalParameters);
}
protected MetaModelGetDslImpl(final JsonEndpoint endpoint, final String identifierToSearchFor, final List> expansionPaths, final E expansionModel, final Function, C> builderFunction, final List additionalParameters) {
this.endpoint = requireNonNull(endpoint);
this.identifierToSearchFor = requireNonNull(identifierToSearchFor);
this.expansionPaths = requireNonNull(expansionPaths);
this.expansionModel = requireNonNull(expansionModel);
this.builderFunction = requireNonNull(builderFunction);
this.additionalParameters = requireNonNull(additionalParameters);
}
@Nullable
@Override
public R deserialize(final HttpResponse httpResponse) {
return Optional.of(httpResponse)
.filter(r -> r.getStatusCode() != NOT_FOUND_404)
.map(r -> deserialize(r, typeReference()))
.orElse(null);
}
@Override
public HttpRequestIntent httpRequestIntent() {
if (!endpoint.endpoint().startsWith("/")) {
throw new RuntimeException("By convention the paths start with a slash, see baseEndpointWithoutId()");
}
final boolean urlEncoded = true;
final UrlQueryBuilder builder = UrlQueryBuilder.of();
expansionPaths().forEach(path -> builder.add(EXPAND, path.toSphereExpand(), urlEncoded));
additionalQueryParameters().forEach(parameter -> builder.add(parameter.getKey(), parameter.getValue(), urlEncoded));
final String queryParameters = builder.toStringWithOptionalQuestionMark();
final String path = endpoint.endpoint() + "/" + identifierToSearchFor + (queryParameters.length() > 1 ? queryParameters : "");
return HttpRequestIntent.of(HttpMethod.GET, path);
}
protected TypeReference typeReference() {
return endpoint.typeReference();
}
protected List additionalQueryParameters() {
return additionalParameters;
}
@Override
public boolean canDeserialize(final HttpResponse httpResponse) {
return httpResponse.hasSuccessResponseCode() || httpResponse.getStatusCode() == NOT_FOUND_404;
}
@Override
public List> expansionPaths() {
return expansionPaths;
}
@Override
public final C withExpansionPaths(final List> expansionPaths) {
return copyBuilder().expansionPaths(expansionPaths).build();
}
@Override
public C withExpansionPaths(final ExpansionPath expansionPath) {
return ExpansionDslUtil.withExpansionPaths(this, expansionPath);
}
@Override
public C withExpansionPaths(final Function> m) {
return ExpansionDslUtil.withExpansionPaths(this, m);
}
@Override
public C plusExpansionPaths(final List> expansionPaths) {
return withExpansionPaths(listOf(expansionPaths(), expansionPaths));
}
@Override
public C plusExpansionPaths(final ExpansionPath expansionPath) {
return ExpansionDslUtil.plusExpansionPaths(this, expansionPath);
}
@Override
public C plusExpansionPaths(final Function> m) {
return ExpansionDslUtil.plusExpansionPaths(this, m);
}
@Override
public E expansionModel() {
return expansionModel;
}
protected MetaModelGetDslBuilder copyBuilder() {
return new MetaModelGetDslBuilder<>(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy