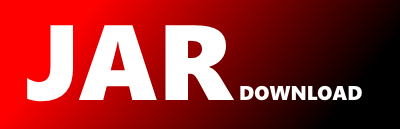
io.sphere.sdk.queries.PagedQueryResult Maven / Gradle / Ivy
package io.sphere.sdk.queries;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
/**
* A container for query responses which contains a subset of the matching values.
* @param the type of the underlying model, like category or product.
*/
public class PagedQueryResult extends PagedResult {
@JsonCreator
PagedQueryResult(final Long offset, final Long total, final List results) {
super(offset, total, results);
}
/**
* Creates a {@code PagedQueryResult} for queries with no matching values.
* @param the type of the underlying model
* @return an empty {@code PagedQueryResult}
*/
public static PagedQueryResultDsl empty() {
return new PagedQueryResultDsl<>(0L, 0L, Collections.emptyList());
}
public static PagedQueryResultDsl of(final Long offset, final Long total, final List results) {
return new PagedQueryResultDsl<>(offset, total, results);
}
public static PagedQueryResultDsl of(final List results) {
return of(0L, Long.valueOf(results.size()), results);
}
@JsonIgnore
public static PagedQueryResultDsl of(final T singleResult) {
return of(Collections.singletonList(singleResult));
}
@Override
public Long getOffset() {
return super.getOffset();
}
@Override
public List getResults() {
return super.getResults();
}
/**
* The total number of results matching the query.
* This field is returned by default.
* For improved performance, calculating this field can be deactivated by using {@link QueryDsl#withFetchTotal(boolean)} and {@code false}.
*
* {@include.example io.sphere.sdk.categories.queries.CategoryQueryTest#withFetchTotalFalseRemovesTotalFromOutput()}
*
* @return total or null
*/
@Override
public Long getTotal() {
return super.getTotal();
}
@Override
public Optional head() {
return super.head();
}
@Override
public boolean isFirst() {
return super.isFirst();
}
@Override
public boolean isLast() {
return super.isLast();
}
@Override
public Long size() {
return super.size();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy