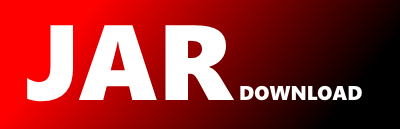
io.sphere.sdk.queries.TimestampSortingModelImpl Maven / Gradle / Ivy
package io.sphere.sdk.queries;
import io.sphere.sdk.utils.IterableUtils;
import javax.annotation.Nullable;
import java.time.ZoneOffset;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
import java.util.List;
import java.util.stream.Collectors;
import static java.util.stream.Collectors.toList;
final class TimestampSortingModelImpl extends QueryModelImpl implements TimestampSortingModel {
public TimestampSortingModelImpl(@Nullable final QueryModel parent, @Nullable final String pathSegment) {
super(parent, pathSegment);
}
@Deprecated
@Override
public QuerySort sort(final QuerySortDirection sortDirection) {
return new SphereQuerySort<>(this, sortDirection);
}
@Override
public DirectionlessQuerySort sort() {
return new DirectionlessQuerySort<>(this);
}
@Override
public QueryPredicate is(final ZonedDateTime value) {
return isPredicate(normalize(value));
}
@Override
public QueryPredicate isGreaterThan(final ZonedDateTime value) {
return ComparisonQueryPredicate.ofIsGreaterThan(this, quotify(value));
}
@Override
public QueryPredicate isGreaterThanOrEqualTo(final ZonedDateTime value) {
return ComparisonQueryPredicate.ofGreaterThanOrEqualTo(this, quotify(value));
}
@Override
public QueryPredicate isLessThan(final ZonedDateTime value) {
return ComparisonQueryPredicate.ofIsLessThan(this, quotify(value));
}
@Override
public QueryPredicate isLessThanOrEqualTo(final ZonedDateTime value) {
return ComparisonQueryPredicate.ofIsLessThanOrEqualTo(this, quotify(value));
}
@Override
public QueryPredicate isIn(final Iterable args) {
return new IsInQueryPredicate<>(this, quotify(args));
}
@Override
public QueryPredicate isNotIn(final Iterable args) {
return new IsNotInQueryPredicate<>(this, quotify(args));
}
private List quotify(final Iterable values) {
return IterableUtils.toStream(values).map(this::quotify).collect(Collectors.toList());
}
@Override
public QueryPredicate isNot(final ZonedDateTime value) {
return isNotPredicate(normalize(value));
}
private String normalize(final ZonedDateTime value) {
return DateTimeFormatter.ISO_INSTANT.format(value.withZoneSameInstant(ZoneOffset.UTC));
}
private String quotify(final ZonedDateTime value) {
return quotify(normalize(value));
}
private String quotify(final String value) {
return StringQuerySortingModel.normalize(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy