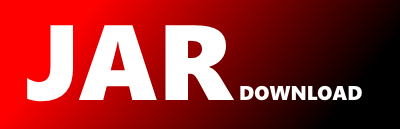
io.sphere.sdk.client.Tokens Maven / Gradle / Ivy
package io.sphere.sdk.client;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.type.TypeReference;
import io.sphere.sdk.models.Base;
import io.sphere.sdk.models.SphereException;
import java.time.ZonedDateTime;
import java.util.Optional;
import static org.apache.commons.lang3.StringUtils.isEmpty;
/** OAuth tokens returned by the authorization server. */
final class Tokens extends Base {
@JsonProperty("access_token")
private final String accessToken;
@JsonProperty("refresh_token")
private final String refreshToken;
@JsonProperty("expires_in")
private final Optional expiresIn;
@JsonIgnore
private final Optional expiresInZonedDateTime;
@JsonCreator
private Tokens(String accessToken, String refreshToken, Optional expiresIn) {
if (isEmpty(accessToken))
throw new SphereException("OAuth response must contain an access_token. Was empty.");
this.accessToken = accessToken;
this.refreshToken = refreshToken;
this.expiresIn = expiresIn;
expiresInZonedDateTime = expiresIn.map(seconds -> ZonedDateTime.now().plusSeconds(seconds));
}
public String getAccessToken() {
return accessToken;
}
public String getRefreshToken() {
return refreshToken;
}
public Optional getExpiresIn() {
return expiresIn;
}
Optional getExpiresZonedDateTime() {
return expiresInZonedDateTime;
}
public static TypeReference typeReference() {
return new TypeReference() {
@Override
public String toString() {
return "TypeReference";
}
};
}
@JsonIgnore
public static Tokens of(String accessToken, String refreshToken, Optional expiresIn) {
return new Tokens(accessToken, refreshToken, expiresIn);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy