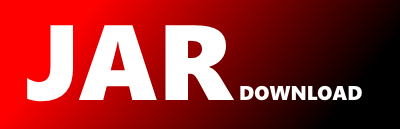
io.sphere.sdk.meta.ConstructionDocumentation Maven / Gradle / Ivy
package io.sphere.sdk.meta;
import io.sphere.sdk.models.LocalizedString;
/**
* This documentation is about creating objects.
*
* SPHERE.IO saves e-commerce data in the cloud. The JVM SDK is one way to read and write the data.
* There can be other tools like the Merchant Center or product sync tools which can also change data.
* As a result the data loaded with the JVM SDK is a record of the present or the
* past since another tool might have changed the data just after loading the data .
* Therefore, data containers are immutable and contain no setters.
*
* Static 'of' method
* To achieve flexibility in the implementation the SDK mostly doesn't support object instantiation by the {@code new} operator.
*
* Simple objects with few optional parameters typically have a static method called {@code of} to create an object.
*
* For example use {@link LocalizedString#of(java.util.Locale, String, java.util.Locale, String)}
* to create a translation for different locales:
*
* {@include.example io.sphere.sdk.meta.ConstructionDocumentationTest#ofMethodExample()}
*
* The {@code of} method is inspired by {@link java.util.Optional#of(Object)} and the Java date and time classes such as {@link java.time.format.DateTimeFormatter#ofPattern(String)}.
*
*
* Builders
*
* For objects with a lot of optional parameters the SDK provides builders. The builder is initialized with a
* static {@code of} method and the mandatory parameters for creating the target object. The builder provides methods
* to fill each target object's optional field.
* To create the target object a method called {@link io.sphere.sdk.models.Builder#build()} has to be executed.
*
* The {@link io.sphere.sdk.models.Address} object with the {@link io.sphere.sdk.models.AddressBuilder}
* is a good example since it contains only the country as mandatory parameter and a lot of optional fields:
*
* {@include.example io.sphere.sdk.meta.ConstructionDocumentationTest#builderExample()}
*
* The invariants of builders in the SDK:
* {@include.example io.sphere.sdk.meta.ConstructionDocumentationTest#builderIsMutableExample()}
*
* Some builders support a model as prototype:
* {@include.example io.sphere.sdk.meta.ConstructionDocumentationTest#builderWithTemplateInput()}
*
* Copy methods
*
* Sometimes you need to clone objects and to update one field since most objects are immutable;
* you can create updated copies with a method starting with "with":
*
* {@include.example io.sphere.sdk.meta.ConstructionDocumentationTest#possibilities1()}
*
* The 'with copy'-methods are inspired by Scala and the Java date and time API: {@link java.time.OffsetDateTime#withMinute(int)}.
*
* Persistent objects vs. local test doubles
*
*
* For persistent changes you need to use a SPHERE.IO
* client and to execute a {@link io.sphere.sdk.commands.Command}. Available commands for the SPHERE.IO resources
* are listed in {@link io.sphere.sdk.meta.SphereResources}
*
* Typically there are three kinds of commands:
*
*
* - {@link io.sphere.sdk.commands.CreateCommand}: command to create a resource
* - {@link io.sphere.sdk.commands.UpdateCommand}: command to update an existing resource
* - {@link io.sphere.sdk.commands.DeleteCommand}: command to delete resources
*
*/
public class ConstructionDocumentation {
private ConstructionDocumentation() {
}
}