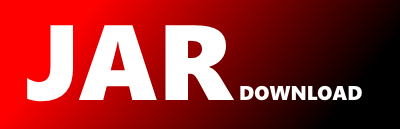
io.sphere.sdk.meta.ProductAttributeDocumentation Maven / Gradle / Ivy
package io.sphere.sdk.meta;
import io.sphere.sdk.products.attributes.AttributeAccess;
import io.sphere.sdk.models.LocalizedString;
import io.sphere.sdk.products.ProductVariantDraftBuilder;
/**
Introduction
ProductType Creation
A {@link io.sphere.sdk.producttypes.ProductType} is like a schema that defines how the product attributes are structured.
{@link io.sphere.sdk.producttypes.ProductType}s contain a list of {@link io.sphere.sdk.products.attributes.AttributeDefinition}s which corresponds to the name and type of each attribute, along with some additional information".
Each name/type pair must be unique across a SPHERE.IO project, so if you create an attribute "foo" of type String, you cannot create
another {@link io.sphere.sdk.producttypes.ProductType} where "foo" has another type (e.g. {@link LocalizedString}). If you do it anyway you get an error message like:
"The attribute with name 'foo' has a different type on product type 'exampleproducttype'."
In this scenario we provide two {@link io.sphere.sdk.producttypes.ProductType}s book and tshirt.
The book product type contains the following attributes:
- {@code isbn} as {@link String}, International Standard Book Number
The tshirt product type contains the following attributes:
- {@code color} as {@link io.sphere.sdk.models.LocalizedEnumValue} with the colors green and red and their translations in German and English
- {@code size} as {@link io.sphere.sdk.models.EnumValue} with S, M and X
- {@code laundrySymbols} as set of {@link io.sphere.sdk.models.LocalizedEnumValue} with temperature and tumble drying
- {@code matchingProducts} as set of product {@link io.sphere.sdk.models.Reference}s, which can point to products that are similar to the current product
- {@code rrp} as {@link javax.money.MonetaryAmount} containing the recommended retail price
- {@code availableSince} as {@link java.time.LocalDateTime} which contains the date since when the product is available for the customer in the shop
All available attribute types you can find here: {@link io.sphere.sdk.products.attributes.AttributeType} in "All Known Implementing Classes".
The code for the creation of the book {@link io.sphere.sdk.producttypes.ProductType}:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#createBookProductType()}
The code for the creation of the tshirt {@link io.sphere.sdk.producttypes.ProductType}:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#createProductType()}
{@link io.sphere.sdk.producttypes.ProductType}s have a name (String)
which can be used as key to logically identify {@link io.sphere.sdk.producttypes.ProductType}s. Beware that the name has no unique constraint,
so it is possible to create multiple {@link io.sphere.sdk.producttypes.ProductType}s with the same name which will cause confusion.
Check if product type with the same name exists:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#demoCheckingIfProductTypeExist()}
Product Creation
To create a product you need to reference the product type. Since the {@link io.sphere.sdk.producttypes.ProductType}
ID of the development system will not be the ID of the production system it is necessary to find the product type by name:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#fetchProductTypeByName()}
The simplest way of adding attributes to a {@link io.sphere.sdk.products.ProductVariant} is to use
{@link ProductVariantDraftBuilder#plusAttribute(java.lang.String, java.lang.Object)} which enables you to directly
put the value of the attribute to the draft. But it cannot check if you put the right objects and types in it.
A book example:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#createBookProduct()}
A tshirt example:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#createProduct()}
A wrong value for a field or an invalid type will cause an {@link io.sphere.sdk.client.ErrorResponseException}
with an error code of {@value io.sphere.sdk.models.errors.InvalidField#CODE}.
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#invalidTypeCausesException()}
As alternative you could declare your attributes at the same place and use these to read and write
attribute values:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#productCreationWithGetterSetter()}
Reading Attributes
To get a value out of an attribute you need an instance of {@link AttributeAccess}
which keeps the type info to deserialize the attribute.
You can reuse the {@link io.sphere.sdk.products.attributes.NamedAttributeAccess} declaration if you want to:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#readAttributeWithoutProductTypeWithNamedAccess()}
Or you can access it on the fly:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#readAttributeWithoutProductTypeWithName()}
Or you can access it as {@link com.fasterxml.jackson.databind.JsonNode}, for example if you don't know the type or the SDK does not support it yet:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#readAttributeWithoutProductTypeWithJson()}
If the attribute is not present in the {@link io.sphere.sdk.products.AttributeContainer} then the {@link java.util.Optional} will be empty:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#notPresentAttributeRead()}
If you provide a wrong type, the code will throw a {@link io.sphere.sdk.json.JsonException}:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#readAttributeWithoutProductTypeWithNamedAccessWithWrongType()}
Creating a table of attributes
With the help of the product type, you can display a table with attributes. Such as:
color | green
----------------------------------------------------------------
size | S
----------------------------------------------------------------
matching products | referenceable product
----------------------------------------------------------------
washing labels | tumble drying, Wash at or below 30°C
----------------------------------------------------------------
recommended retail price | EUR300.00
----------------------------------------------------------------
available since | 2015-02-02
----------------------------------------------------------------
In this example the left column is the label of
the attribute from the product type and the right column is the formatted value from the product:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#showProductAttributeTable()}
Update attribute values of a product
Setting attribute values is like a a product creation:
Example for books:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#updateAttributesBooks()}
Example for tshirts:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#updateAttributes()}
A wrong value for a field or an invalid type (like flat value instead of set) will cause an {@link io.sphere.sdk.client.ErrorResponseException}
with an error code of {@value io.sphere.sdk.models.errors.InvalidField#CODE}.
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#updateWithWrongType()}
Create attribute stubs for unit tests
For unit tests you can create an {@link io.sphere.sdk.products.attributes.Attribute} with a
static factory method such as {@link io.sphere.sdk.products.attributes.Attribute#of(String, AttributeAccess, Object)}:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#attributesForUnitTests()}
Create attributes for importing orders
Importing attribute values for orders works different from updating products.
In orders you provide the full value for enum-like types instead of just the key as done for all other types.
This makes it possible to create a new enum value on the fly. The other attributes behave as expected.
Example:
{@include.example io.sphere.sdk.attributestutorial.ProductTypeCreationDemoTest#orderImportExample()}
Semantics of the Attribute classes
attribute properties
{@link io.sphere.sdk.products.attributes.Attribute} {@link io.sphere.sdk.products.attributes.AttributeDraft} {@link io.sphere.sdk.products.attributes.AttributeImportDraft}
purpose read access write product, create product order import
reference expansion keeps expanded references no expansion no expansion
enum shape full enum enum key (String) full enum
value constraints value in product type value in product type free, create on the fly
Nested attributes (experimental)
This feature is experimental.
An example:
{@include.example io.sphere.sdk.producttypes.NestedAttributeIntegrationTest}
A general explanation can be found in the HTTP API tutorial.
*/
public class ProductAttributeDocumentation {
private ProductAttributeDocumentation() {
}
}