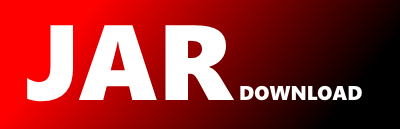
io.sphere.sdk.meta.ReleaseNotes Maven / Gradle / Ivy
package io.sphere.sdk.meta;
import io.sphere.sdk.carts.LineItem;
import io.sphere.sdk.carts.queries.CartByIdGet;
import io.sphere.sdk.categories.Category;
import io.sphere.sdk.channels.queries.ChannelByIdGet;
import io.sphere.sdk.client.SphereRequest;
import io.sphere.sdk.commands.UpdateActionImpl;
import io.sphere.sdk.expansion.ExpansionPath;
import io.sphere.sdk.http.ApacheHttpClientAdapter;
import io.sphere.sdk.http.HttpResponse;
import io.sphere.sdk.http.AsyncHttpClientAdapter;
import io.sphere.sdk.models.Identifiable;
import io.sphere.sdk.models.LocalizedString;
import io.sphere.sdk.orders.Order;
import io.sphere.sdk.productdiscounts.queries.ProductDiscountByIdGet;
import io.sphere.sdk.products.ProductProjectionType;
import io.sphere.sdk.products.ProductVariant;
import io.sphere.sdk.products.commands.updateactions.SetMetaDescription;
import io.sphere.sdk.products.commands.updateactions.SetMetaKeywords;
import io.sphere.sdk.products.commands.updateactions.SetMetaTitle;
import io.sphere.sdk.products.queries.ProductByIdGet;
import io.sphere.sdk.products.queries.ProductProjectionByIdGet;
import io.sphere.sdk.products.queries.ProductProjectionQuery;
import io.sphere.sdk.queries.Get;
import io.sphere.sdk.queries.QueryPredicate;
import javax.money.CurrencyUnit;
import java.util.concurrent.CompletionStage;
import java.util.function.BiFunction;
import java.util.function.Function;
/**
Legend
- removed functionality
- added functionality
- breaking change
- bugfix, can include a breaking change
1.0.0-M16
This release is intended to be the last release before 1.0.0-RC1.
- {@link io.sphere.sdk.productdiscounts.commands.ProductDiscountCreateCommand},
{@link io.sphere.sdk.productdiscounts.commands.ProductDiscountUpdateCommand},
{@link io.sphere.sdk.productdiscounts.commands.ProductDiscountDeleteCommand},
{@link ProductDiscountByIdGet},
{@link io.sphere.sdk.productdiscounts.queries.ProductDiscountQuery}
- More reference expansion for product fields.
- Added a {@link ProductAttributeDocumentation product attribute tutorial}.
- Added a {@link CategoryDocumentation category tutorial}.
- Added the {@link io.sphere.sdk.messages.queries.MessageQuery message endpoint}.
- Added query and expansion meta models for carts.
- {@link io.sphere.sdk.utils.MoneyImpl#ofCents(long, CurrencyUnit)} can be used to create monetary values
- Customer can be created with attributes dateOfBirth, companyName, vatId, isEmailVerified, customerGroup and addresses.
- External images can be included in the product creation.
- Added {@link io.sphere.sdk.inventory.commands.updateactions.ChangeQuantity} to update {@link io.sphere.sdk.inventory.InventoryEntry}s.
- Added {@link io.sphere.sdk.channels.ChannelRole#PRODUCT_DISTRIBUTION}.
- Added {@link LineItem#getDistributionChannel()}.
- Meta models can be used in the product search, too. See {@link io.sphere.sdk.products.search.ProductProjectionSearch#plusFacetFilters(Function)}.
- Added documentation how to query for large offsets in {@link QueryDocumentation}.
- Added hints how to format date and monetary data in {@link FormattingDocumentation}.
- Added {@link io.sphere.sdk.producttypes.commands.ProductTypeUpdateCommand}.
- To imrove the query speed the calculation of the total amount of items in commercetools platform can be deactivated with {@link io.sphere.sdk.products.queries.ProductQuery#withFetchTotal(boolean)}.
- {@link QueryPredicate}s can be negated with {@link QueryPredicate#negate()}
- Added {@link io.sphere.sdk.carts.CartState#ORDERED} and {@link Order#getCart()}.
- Added {@link io.sphere.sdk.products.ProductProjection#findVariantBySky(String)}.
- Reference expansion for the product projection search.
- The default behaviour of queries changed, things are not sorted by ID by default for performance reasons. The impact is that performing the exact query again may yields different results.
- {@link java.util.Optional} as return type for optional values has been removed, now the annotation {@link javax.annotation.Nullable} is used to mark objects as not mandatory. This change was necessary to provide a stable API, to provide later serializable Objects and sparse representations for objects.
- For API read objects primitives have been replaced by wrapper classed to implement later sparse representations. This may affect type conversions which won't work anymore.
- The instantiation of the {@link io.sphere.sdk.client.SphereClient} has been changed, see {@link GettingStarted} and {@link io.sphere.sdk.client.SphereClientFactory}.
- Product type creation has been refactored. Have a look at {@link ProductAttributeDocumentation product attribute tutorial}.
- Product prices have an ID and price updates need to be performed via the price ID: {@link io.sphere.sdk.products.commands.updateactions.ChangePrice}, {@link io.sphere.sdk.products.commands.updateactions.RemovePrice}. Keep in mind that {@link io.sphere.sdk.products.Price#equals(Object)} includes the ID.
- The naming conventions in the commercetools platform have been changed:
- PlainEnumValue is now EnumValue
- LocalizedStrings is now LocalizedString
- LocalizedStringsEntry is now LocalizedStringEntry
- Fetch is now Get (e.g. {@code ProductProjectionByIdFetch} is now {@link ProductProjectionByIdGet}
- {@code JsonUtils} have been renamed to {@link io.sphere.sdk.json.SphereJsonUtils}, this is an internal utility class working for the commercetools platform context, it is not intended to build apps or libs on it.
- {@code ChannelRoles} have been renamed to {@link io.sphere.sdk.channels.ChannelRole}.
- {@code SearchText} is now {@link io.sphere.sdk.models.LocalizedStringEntry}
- Removed {@code ProductUpdateScope}, so all product update actions update only staged.
- Builders for resources (read objects) have been removed. See {@link TestingDocumentation} to build the objects anyway.
- QueryToFetch adapter has been removed.
- {@link io.sphere.sdk.queries.StringQueryModel#isGreaterThan(Object)} and other methods do not quote strings correctly. See 558.
- Product variant expansion does not work. See 631.
1.0.0-M15
Expand all
- {@code io.sphere.sdk.products.search.ExperimentalProductProjectionSearchModel#productType()} enables you to build search expressions with the Product Type reference of products
- {@link ProductVariant#getIdentifier()} enables to get the product id and the variant id from the variant. This is nice, since this data is often needed and on the product variant level the product ID is not available.
- Error reporting has been improved. Especially if JSON mappings do not fit.
- {@link LineItem#getProductSlug()}
- Send User-Agent head for oauth requests.
- A lot of more reference expansions.
- Reference expansion for single elements of a list, example: {@link io.sphere.sdk.categories.expansion.CategoryExpansionModel#ancestors(int)}.
- Reference expansion for fetch endpoints, e.g., {@link ProductProjectionByIdGet#withExpansionPaths(ExpansionPath)}.
- Set the reference expansion, query predicate and sort expression more convenient (less imports, less class search) with lambdas.
Migration pains: {@code QueryDsl
} will be {@code CategoryQuery}
Benefits of the new API:
{@include.example example.QueryMetaDslDemo#demo1()}
instead of
{@include.example example.QueryMetaDslDemo#demo2()}
In Scala (with add-ons) it will look like val query = ProductProjectionQuery.ofCurrent()
.withPredicateScala(_.productType.id.is("product-type-id-1"))
.withSortScala(_.name.lang(ENGLISH).sort.asc)
.withExpansionPathsScala(_.productType)
-
DSL for expansion of references in a product attribute.
{@include.example io.sphere.sdk.products.expansion.ProductVariantExpansionModelTest#productAttributeReferenceExpansion()}
- {@link io.sphere.sdk.reviews.commands.ReviewDeleteCommand}
- {@link io.sphere.sdk.client.SphereClientConfig#ofEnvironmentVariables(String)} to get the
- The product attributes have been refactored, look at the {@link ProductAttributeDocumentation} how it works now.
- {@link io.sphere.sdk.client.SphereClient} implements {@link AutoCloseable} instead of {@link java.io.Closeable}.
- For timestamps we moved from {@link java.time.Instant} to {@link java.time.ZonedDateTime} since the latter also contains a timezone which better reflects SPHERE.IOs date time data.
- Getting the child categories of a category is not in category anymore but in {@link io.sphere.sdk.categories.CategoryTree#findChildren(Identifiable)}.
- Sphere client does not shutdown actors properly. See #491.
- {@code Category#getPathInTree()}
- {@code ExperimentalProductImageUploadCommand}, but you can find a similar command here: https://github.com/sphereio/sphere-jvm-sdk-experimental-java-add-ons
1.0.0-M14
- New fields in {@link io.sphere.sdk.products.Price}: {@link io.sphere.sdk.products.Price#validFrom} and {@link io.sphere.sdk.products.Price#validUntil}.
- Use {@link io.sphere.sdk.products.queries.ProductProjectionQueryModel#allVariants()} to formulate a predicate for all variants. In SPHERE.IO the json fields masterVariant (object) and variants (array of objects) together contain all variants.
- Using {@link ProductProjectionQuery#ofCurrent()} and {@link ProductProjectionQuery#ofStaged()} saves you the import of {@link ProductProjectionType}.
- {@link CompletionStage} does not support by default timeouts which are quite important in a reactive application so you can decorate the {@link io.sphere.sdk.client.SphereClient} with {@link io.sphere.sdk.client.TimeoutSphereClientDecorator} to get a {@link java.util.concurrent.TimeoutException} after a certain amount of time. But this does NOT cancel the request to SPHERE.IO.
- The {@link io.sphere.sdk.reviews.Review} endpoints and models are implemented, but we suggest to not use it, since {@link io.sphere.sdk.reviews.Review}s cannot be deleted or marked as hidden.
- New endpoint: Use {@link io.sphere.sdk.projects.queries.ProjectGet} to get the currencies, countries and languages of the SPHERE.IO project.
- Categories with SEO meta attributes {@link Category#getMetaTitle()}, {@link Category#getMetaDescription()} and {@link Category#getMetaKeywords()} and
update actions {@link io.sphere.sdk.categories.commands.updateactions.SetMetaTitle}, {@link io.sphere.sdk.categories.commands.updateactions.SetMetaDescription} and {@link io.sphere.sdk.categories.commands.updateactions.SetMetaKeywords}.
- Cart discounts: {@link io.sphere.sdk.cartdiscounts.commands.CartDiscountCreateCommand}.
- Discount codes: {@link io.sphere.sdk.discountcodes.commands.DiscountCodeCreateCommand}.
- {@link io.sphere.sdk.client.SphereApiConfig}, {@link io.sphere.sdk.client.SphereAuthConfig}, {@link io.sphere.sdk.client.SphereClientConfig} validates the input, so for example you cannot enter null or whitespace for the project key.
- Date time attributes in {@code ProductProjectionSearchModel} are using ZonedDateTime instead of LocalDateTime.
- The {@code ProductProjectionSearchModel} has been improved with better naming and better documentation.
- Sort related classes for the Query API have been renamed with a "Query" prefix, to distinguish them from the Search API sort classes.
- {@code io.sphere.sdk.queries.Predicate} has been renamed to {@link io.sphere.sdk.queries.QueryPredicate}.
- The JVM SDK itself uses for tests the assertj assertion methods instead of fest assertions.
- {@code io.sphere.sdk.products.commands.updateactions.SetMetaAttributes} has been removed since it is deprecated in SPHERE.IO.
Use {@link SetMetaTitle},
{@link SetMetaDescription},
{@link SetMetaKeywords} or {@link io.sphere.sdk.products.commands.updateactions.MetaAttributesUpdateActions} for all together.
- Update of the Java Money library to version 1.0 which has some breaking changes in comparison to the release candidates.
- Some predicate methods have been renamed: "isOneOf" to "isIn", "isAnyOf" to "isIn", "isLessThanOrEquals" to "isLessThanOrEqualTo" (analog for greaterThan).
- The exception for a failing {@link io.sphere.sdk.customers.commands.CustomerSignInCommand} shows more problem details. See #397.
- There has been a thread leak if {@code io.sphere.sdk.client.SphereClientFactory#createClient(io.sphere.sdk.client.SphereClientConfig)} was used.
It created twice a http client instance and closed just one.
1.0.0-M13
- {@link LocalizedString#mapValue(BiFunction)} and {@link LocalizedString#stream()}
can be used transform {@link LocalizedString}, for example for creating slugs or formatting.
- Experimental {@link io.sphere.sdk.utils.CompletableFutureUtils} to work with Java 8 Futures.
- {@link AsyncDocumentation} documents how to work with {@link java.util.concurrent.CompletableFuture} and {@link java.util.concurrent.CompletionStage}.
- {@link io.sphere.sdk.models.SphereException}s may give hint to developers how to recover from errors. For example on typical elasticsearch related problems it suggests to reindex the product index.
- The JVM SDK is available for Ning Async HTTP Client 1.8 and 1.9 (incompatible to 1.8).
- State update actions and {@code io.sphere.sdk.states.StateBuilder} contributed by Ansgar Brauner
- Embedded predicates (as used in 'where'-clauses) now support lambda syntax: {@link io.sphere.sdk.products.queries.ProductVariantQueryModel#where(java.util.function.Function)}.
- {@link io.sphere.sdk.orders.queries.OrderQuery#byCustomerId(java.lang.String)} and {@link io.sphere.sdk.orders.queries.OrderQuery#byCustomerEmail(java.lang.String)}
- {@link io.sphere.sdk.channels.commands.ChannelUpdateCommand}
- {@link ChannelByIdGet}
- {@link io.sphere.sdk.customers.queries.CustomerQuery#byEmail(java.lang.String)}
- {@link io.sphere.sdk.client.SphereRequest} has been refactored:
- {@code Function
resultMapper()} is now {@link io.sphere.sdk.client.SphereRequest#deserialize(HttpResponse)}
- {@code boolean canHandleResponse(final HttpResponse response)} is now {@link io.sphere.sdk.client.SphereRequest#canDeserialize(HttpResponse)}
- Artifact IDs start now with "sphere-".
- {@link java.util.concurrent.CompletableFuture} has been replaced with {@link java.util.concurrent.CompletionStage}.
You can convert from {@link java.util.concurrent.CompletionStage} to {@link java.util.concurrent.CompletableFuture} with {@link CompletionStage#toCompletableFuture()}.
The opposite direction can be achieved with assigning {@link java.util.concurrent.CompletableFuture} (implementation) to {@link java.util.concurrent.CompletionStage} (interface).
- Refactoring of {@link io.sphere.sdk.client.SphereClient}, {@code
CompletionStage execute(final SphereRequest sphereRequest)}
is now {@link io.sphere.sdk.client.SphereClient#execute(SphereRequest)} which returns {@link java.util.concurrent.CompletionStage} instead of {@link java.util.concurrent.CompletableFuture}.
- {@link io.sphere.sdk.carts.LineItem#getTaxRate()} is optional.
- {@link io.sphere.sdk.carts.LineItem#getState()} now returns a set instead of a list.
- {@link io.sphere.sdk.products.ProductProjection#getCategories()} now returns a set instead of a list.
- URL encoding of parameters. See #240.
1.0.0-M12
- Added the {@link io.sphere.sdk.orders.commands.OrderImportCommand}.
- Added the nested attributes: {@code io.sphere.sdk.attributes.AttributeAccess#ofNested()} + {@code io.sphere.sdk.attributes.AttributeAccess#ofNestedSet()}.
- The error JSON body from SPHERE.IO responses can be directly extracted as JSON with {@link io.sphere.sdk.client.SphereServiceException#getJsonBody()}.
- {@link io.sphere.sdk.http.HttpResponse} also contains {@link io.sphere.sdk.http.HttpHeaders}.
- Experimental search filter/facet/sort expression model {@code ProductProjectionSearchModel}. See also {@link io.sphere.sdk.meta.ProductSearchDocumentation}.
- The {@link io.sphere.sdk.producttypes.ProductType} creation has been simplified (TextAttributeDefinition, LocalizedStringsAttributeDefinition, ... are just AttributeDefinition), see {@link io.sphere.sdk.producttypes.commands.ProductTypeCreateCommand} how to create them.
- {@link io.sphere.sdk.search.TermFacetResult} and
{@link io.sphere.sdk.search.RangeFacetResult} are using generics.
{@link io.sphere.sdk.search.TermFacetResult} uses long instead of int for some methods like {@link io.sphere.sdk.search.TermFacetResult#getMissing()}.
- Methods in {@link io.sphere.sdk.search.SearchDsl} have been renamed.
- {@code io.sphere.sdk.search.RangeStats#getMean()} now returns a double.
- {@link io.sphere.sdk.http.HttpHeaders} allows reoccurring headers.
- Use of a new toString style, from
io.sphere.sdk.client.HttpRequestIntent@7308a939[httpMethod=POST,path=/categories,headers={},body=Optional[io.sphere.sdk.http.StringHttpRequestBody@216ec9be[body={invalidJson :)]]]
to HttpRequestIntent[httpMethod=POST,path=/categories,headers={},body=Optional[StringHttpRequestBody[body={invalidJson :)]]]
- {@link io.sphere.sdk.client.ErrorResponseException#getMessage()} now returns also the project debug info.
- Incompatibility with Jackson 2.5.1 has been fixed. A cause message was "No suitable constructor found for type [simple type, class io.sphere.sdk.models.ImageImpl]: can not instantiate from JSON object (missing default constructor or creator, or perhaps need to add/enable type information?)"
1.0.0-M11
Overall
- Code examples contain the links to the GitHub source code.
- The {@link io.sphere.sdk.client.SphereClient} architecture has been refactored, so it is now possible to inject access token suppliers and custom HTTP clients.
- {@link AsyncHttpClientAdapter} enables to use a custom underlying Ning HTTP client for settings like proxies or max connections per host.
- The new module {@code java-client-apache-async} contains an {@link ApacheHttpClientAdapter adapter} to use the Apache HTTP client instead of the current default client Ning.
- The {@link io.sphere.sdk.client.QueueSphereClientDecorator} enables to limit the amount of concurrent requests to SPHERE.IO with a task queue.
- {@link io.sphere.sdk.client.SphereAccessTokenSupplierFactory} is a starting point to create custom access token suppliers for one token (either fetched from SPHERE.IO or as String) or auto refreshing for online shops.
- Added {@link io.sphere.sdk.client.SphereRequestDecorator} to decorate {@link io.sphere.sdk.client.SphereRequest}s.
- {@link io.sphere.sdk.meta.ExceptionDocumentation Exception hierarchy}, relocated some exceptions and deleted some.
- Removed SphereBackendException, SphereClientException, JavaConcurrentUtils, Requestable
- Removed ReferenceExistsException, usage as {@code SphereError} from a {@link io.sphere.sdk.client.ErrorResponseException}
- JsonParseException is now {@link io.sphere.sdk.json.JsonException}.
- InvalidQueryOffsetException is replaced with {@link java.lang.IllegalArgumentException}.
- For SDK devs: {@link io.sphere.sdk.http.HttpRequest} has changed tasks and structure, now it contains the full information for a HTTP request whereas now {@link io.sphere.sdk.client.HttpRequestIntent} is an element to describe an endpoint of sphere project independent.
- For SDK devs: {@link io.sphere.sdk.client.JsonEndpoint} moved to the client package
- {@link Get} class names end now with Fetch for consistency, so for example {@code CartFetchById} is now {@link CartByIdGet}.
- {@link io.sphere.sdk.commands.DeleteCommand} implementations don't have {@code ById} or {@code ByKey} in the class name and the {@code of} factory method returns the interface, not the implementation, example {@link io.sphere.sdk.categories.commands.CategoryDeleteCommand#of(io.sphere.sdk.models.Versioned)}.
- LocalizedText* classes have been renamed to LocalizedString.
- Fixed: UnknownCurrencyException #264.
States
- {@link io.sphere.sdk.states.State} models, creation and deletion contributed by Ansgar Brauner
Products
- Added update actions: {@link io.sphere.sdk.products.commands.updateactions.AddVariant}, {@link io.sphere.sdk.products.commands.updateactions.RemoveFromCategory}, {@link io.sphere.sdk.products.commands.updateactions.RemoveVariant}, {@link io.sphere.sdk.products.commands.updateactions.RevertStagedChanges}, {@link io.sphere.sdk.products.commands.updateactions.SetAttribute}, {@link io.sphere.sdk.products.commands.updateactions.SetAttributeInAllVariants}, {@link io.sphere.sdk.products.commands.updateactions.SetSearchKeywords}
1.0.0-M10
- Added {@link io.sphere.sdk.customobjects.CustomObject} models and endpoints. There is also a {@link io.sphere.sdk.meta.CustomObjectDocumentation tutorial for custom objects}.
- Added the {@link io.sphere.sdk.zones.Zone} models and endpoints.
- Added the {@link io.sphere.sdk.shippingmethods.ShippingMethod} models and endpoints.
- Added Typesafe Activator files, so you can edit the SDK on UNIX with {@code ./activator ui} or on Windows with {@code activator ui}.
- Added io.sphere.sdk.client.ConcurrentModificationException which is thrown when an {@link io.sphere.sdk.commands.UpdateCommand} fails because of concurrent usage.
- Added io.sphere.sdk.client.ReferenceExistsException which is thrown when executing a {@link io.sphere.sdk.commands.DeleteCommand} and the resource is referenced by another resource and cannot be deleted before deleting the other resource.
- Added io.sphere.sdk.queries.InvalidQueryOffsetException which is thrown if offset is
not between {@value io.sphere.sdk.queries.Query#MIN_OFFSET} and {@value io.sphere.sdk.queries.Query#MAX_OFFSET}..
- Improved on different location the structure of interfaces so important methods are highlighted bold in the IDE.
- JSON mapping errors are better logged.
- Added update actions for cart: {@link io.sphere.sdk.carts.commands.updateactions.SetShippingMethod} and {@link io.sphere.sdk.carts.commands.updateactions.SetCustomerId}.
- Added update actions for customer: {@link io.sphere.sdk.carts.commands.updateactions.SetCustomerId}.
- Added {@link io.sphere.sdk.models.Referenceable#hasSameIdAs(io.sphere.sdk.models.Referenceable)} to check if a similar object has the same ID.
- Added {@code AttributeAccess#ofName(String)} as alias to {@code io.sphere.sdk.attributes.AttributeAccess#getterSetter(String)}.
- Update action list in update commands do not have the type {@literal List
>} {@literal List extends UpdateAction>}, so you can pass a list of a subclass of {@link UpdateActionImpl}.
Example: {@literal List} can be assigned where {@literal ChangeName} extends {@link UpdateActionImpl}.
- Added {@link io.sphere.sdk.models.Address#of(com.neovisionaries.i18n.CountryCode)}.
- Added {@code io.sphere.sdk.carts.Cart#getLineItem(String)} and {@code io.sphere.sdk.carts.Cart#getCustomLineItem(String)} to find items in a cart.
- Added {@link io.sphere.sdk.products.ProductProjection#getAllVariants()} to receive master variant and all other variants in one call. {@link io.sphere.sdk.products.ProductProjection#getVariants()} just returns all variants except the master variant.
- Added {@link io.sphere.sdk.products.ProductProjection#getVariant(int)} and {@link io.sphere.sdk.products.ProductProjection#getVariantOrMaster(int)} to find a product variant by id.
- Added {@link io.sphere.sdk.products.VariantIdentifier} to have a container to address product variants which needs a product ID and a variant ID.
- added {@link io.sphere.sdk.customers.commands.CustomerDeleteCommand} to delete customers.
- Added {@link io.sphere.sdk.products.commands.updateactions.AddExternalImage} to connect products with images not hosted by SPHERE.IO.
- Added {@link io.sphere.sdk.products.commands.updateactions.RemoveImage} to disconnect images from a product (external images and SPHERE.IO hosted).
- Added {@link io.sphere.sdk.client.SphereAccessTokenSupplier} as authentication method in the {@link io.sphere.sdk.client.SphereClient}.
It is possible to automatically refresh a token or just pass a token to the client, see {@link io.sphere.sdk.client.SphereClientFactory#createClient(io.sphere.sdk.client.SphereApiConfig, io.sphere.sdk.client.SphereAccessTokenSupplier)} and {@link io.sphere.sdk.client.SphereAccessTokenSupplier#ofConstantToken(String)}.
- Product variants are all of type int, was int and long before.
- {@link io.sphere.sdk.models.Reference} is not instantiated with new.
- {@link io.sphere.sdk.http.UrlQueryBuilder} is not instantiated with new.
- io.sphere.sdk.client.SphereErrorResponse is not instantiated with new.
- {@code ClientRequest} has been renamed to {@link io.sphere.sdk.client.SphereRequest} and therefore {@code ClientRequestBase} to {@link io.sphere.sdk.client.SphereRequestBase}.
- {@code ClientRequest} has been renamed to {@link io.sphere.sdk.client.SphereRequest} and therefore {@code ClientRequestBase} to {@link io.sphere.sdk.client.SphereRequestBase}.
- {@code JavaClient} has been renamed to {@link io.sphere.sdk.client.SphereClient} and uses the {@link io.sphere.sdk.client.SphereClientFactory} to initialized a client, {@code JavaClientIml} has been removed, see {@link io.sphere.sdk.meta.GettingStarted}.
The typesafe config library is not used anymore. The class {@code HttpClientTestDouble} has been removed, use {@link io.sphere.sdk.client.SphereClientFactory#createHttpTestDouble(java.util.function.Function)} instead.
{@code SphereRequestExecutor} and {@code SphereRequestExecutorTestDouble} have been removed, use {@link io.sphere.sdk.client.SphereClientFactory#createObjectTestDouble(java.util.function.Function)} instead.
- Money portions in the taxed price is not null. The method name is now {@link io.sphere.sdk.carts.TaxPortion#getAmount()} instead of {@code getMoney()}.
- Fixed JSON serialization and deserialization of {@code ImageDimensions} which caused adding external images to a product to fail.
1.0.0-M9
- Added Known Issues page.
- Added experimental support for uploading product images in variants. See {@code io.sphere.sdk.products.commands.ExperimentalProductImageUploadCommand}.
- Added factory methods for {@code Image}.
- {@code Image} contains directly getters for width {@code Image#getWidth()}
and height {@code Image#getHeight()}.
- {@link io.sphere.sdk.queries.PagedQueryResult} is constructable for empty results. Before this, the SDK throwed an Exception.
- Fields called {@code quantity} are now of type long instead of int.
- Added a documentation page {@link io.sphere.sdk.meta.ConstructionDocumentation how to construct objects}.
1.0.0-M8
- Query models contain id, createdAt and lastModifiedAt for predicates and sorting.
- Introduced endpoints and models for {@link io.sphere.sdk.carts.Cart}s, {@link io.sphere.sdk.customers.Customer}s, {@link io.sphere.sdk.customergroups.CustomerGroup}s and {@link io.sphere.sdk.orders.Order}s.
- Quantity fields are now of type long.
- Classes like {@link ProductByIdGet} take now a string parameter for the ID and not an {@link io.sphere.sdk.models.Identifiable}.
- Queries, Fetches, Commands and Searches are only instantiable with an static of method like {@link io.sphere.sdk.categories.commands.CategoryCreateCommand#of(io.sphere.sdk.categories.CategoryDraft)}. The instantiation by constructor is not supported anymore.
- Enum constant names are only in upper case.
1.0.0-M7
- Incompatible change: Classes to create templates for new entries in SPHERE.IO like {@code NewCategory} have been renamed to {@link io.sphere.sdk.categories.CategoryDraft}.
- Incompatible change: {@link io.sphere.sdk.producttypes.ProductTypeDraft} has now only
factory methods with an explicit parameter for the attribute declarations to prevent to use
the getter {@link io.sphere.sdk.producttypes.ProductTypeDraft#getAttributes()} and list add operations.
- Incompatible change: {@code LocalizedString} has been renamed to {@link LocalizedString}, since it is not a container for one string and a locale, but for multiple strings of different locals. It is like a map.
- Incompatible change: The {@link Get} classes have been renamed. From FetchRESOURCEByWhatever to RESOURCEFetchByWhatever
- Moved Scala and Play clients out of the Git repository to https://github.com/sphereio/sphere-jvm-sdk-scala-add-ons. The artifact ID changed.
- {@link io.sphere.sdk.meta.SphereResources} contains now also a listing of queries and commands for the resources.
- Added {@link io.sphere.sdk.products.search.ProductProjectionSearch} for full-text, filtered and faceted search.
- Incompatible change: {@code io.sphere.sdk.products.ProductUpdateScope} makes it more visible that product update operations can be for only staged or for current and staged. The product update actions will be affected by that.
- Implemented anonymous carts.
1.0.0-M6
- Usage of Java money instead of custom implementation.
- Introduce {@link io.sphere.sdk.products.queries.ProductProjectionQuery}.
- Introduce {@link io.sphere.sdk.meta.QueryDocumentation} to document the query API.
1.0.0-M5
- Fixed client shutdown problem.
- Put {@link io.sphere.sdk.models.MetaAttributes MetaAttributes} in common module and make it an interface.
- Add {@link io.sphere.sdk.products.ProductProjection#isPublished()}.
- Add {@link io.sphere.sdk.productdiscounts.ProductDiscount ProductDiscount} models.
- Add {@link io.sphere.sdk.categories.Category#getExternalId() external id fields and methods} for categories.
- Make {@link io.sphere.sdk.products.ProductCatalogData#getCurrent()} return an optional {@link io.sphere.sdk.products.ProductData}, since current should not be accessible if {@link io.sphere.sdk.products.ProductCatalogData#isPublished()} returns false.
- Make masterVariant in {@link io.sphere.sdk.products.ProductDraftBuilder} mandatory.
1.0.0-M4
- Replacing joda time library with Java 8 DateTime API.
- Removing dependency to Google Guava.
- Rename artifact organization to {@code io.sphere.sdk.jvm}.
- Rename {@code JsonUtils.readObjectFromJsonFileInClasspath} to {@code JsonUtils.readObjectFromResource}.
- Reduced the number of SBT modules to speed up travis builds since the resolving of artifacts for every module is slow. In addition fewer JARs needs to be downloaded.
- Introduced {@link io.sphere.sdk.products.ProductProjection}s.
- Javadoc does contain a table of content box for h3 headings.
1.0.0-M3
- The query model can now be accessed by it's Query class, e.g., {@code io.sphere.sdk.categories.queries.CategoryQuery#model()}.
- Added a {@link io.sphere.sdk.meta.GettingStarted Getting Started} page.
- Added a {@link io.sphere.sdk.meta.JvmSdkFeatures Features of the SDK} page.
- Addad a legacy Play Java client for Play Framework 2.2.x.
- Added {@link io.sphere.sdk.products.PriceBuilder}.
- Further null checks.
- Add a lot of a Javadoc, in general for the packages.
- {@link io.sphere.sdk.categories.CategoryTree#of(java.util.List)} instead of CategoryTreeFactory is to be used for creating a category tree.
- Move {@link io.sphere.sdk.models.AddressBuilder} out of the {@link io.sphere.sdk.models.Address} class.
- Performed a lot of renamings like the {@code requests} package to {@code http}
- Moved commands and queries to own packages for easier discovery.
- Introduced new predicates for inequality like {@link io.sphere.sdk.queries.StringQueryModel#isGreaterThanOrEqualTo(String)},
{@link io.sphere.sdk.queries.StringQueryModel#isNot(String)},
{@code io.sphere.sdk.queries.StringQueryModel#isNotIn(String, String...)} or {@link io.sphere.sdk.queries.StringQueryModel#isNotPresent()}.
- Introduced an unsafe way to create predicates from strings with {@link QueryPredicate#of(String)}.
1.0.0-M2
- With the new reference expansion dsl it is possible to discover and create reference expansion paths for a query.
- All artifacts have the ivy organization {@code io.sphere.jvmsdk}.
- Migration from Google Guavas com.google.common.util.concurrent.ListenableFuture to Java 8 java.util.concurrent.CompletableFuture.
- Removed all Google Guava classes from the public API (internally still used).
- The logger is more fine granular controllable, for example the logger {@code sphere.products.responses.queries} logs only the responses of the queries for products. The trace level logs the JSON of responses and requests as pretty printed JSON.
- Introduced the class {@code io.sphere.sdk.models.Referenceable} which enables to use a model or a reference to a model as parameter, so no direct call of {@code io.sphere.sdk.models.DefaultModel#toReference()} is needed anymore for model classes.
- It is possible to overwrite the error messages of {@code io.sphere.sdk.test.DefaultModelAssert}, {@code io.sphere.sdk.test.LocalizedStringAssert} and {@code io.sphere.sdk.test.ReferenceAssert}.
- {@link io.sphere.sdk.models.Versioned} contains a type parameter to prevent copy and paste errors.
- Sorting query model methods have better support in the IDE, important methods are bold.
- Queries and commands for models are in there own package now and less coupled to the model.
- The query classes have been refactored.
*/
public final class ReleaseNotes {
private ReleaseNotes() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy