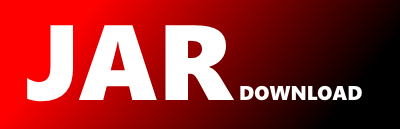
io.sphere.sdk.carts.CartImpl Maven / Gradle / Ivy
package io.sphere.sdk.carts;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.neovisionaries.i18n.CountryCode;
import io.sphere.sdk.customergroups.CustomerGroup;
import io.sphere.sdk.discountcodes.DiscountCodeInfo;
import io.sphere.sdk.models.Address;
import io.sphere.sdk.models.ResourceImpl;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.types.CustomFields;
import javax.annotation.Nullable;
import javax.money.MonetaryAmount;
import java.time.ZonedDateTime;
import java.util.List;
class CartImpl extends ResourceImpl implements Cart {
@Nullable
private final String customerId;
@Nullable
private final String customerEmail;
private final List lineItems;
private final List customLineItems;
private final MonetaryAmount totalPrice;
@Nullable
private final TaxedPrice taxedPrice;
private final CartState cartState;
@Nullable
private final Address shippingAddress;
@Nullable
private final Address billingAddress;
private final InventoryMode inventoryMode;
@Nullable
private final Reference customerGroup;
@Nullable
private final CountryCode country;
@Nullable
private final CartShippingInfo shippingInfo;
private final List discountCodes;
@Nullable
private final CustomFields custom;
@Nullable
private final PaymentInfo paymentInfo;
@JsonCreator
CartImpl(final String id, final Long version, final ZonedDateTime createdAt,
final ZonedDateTime lastModifiedAt, final String customerId,
final String customerEmail, final List lineItems,
final List customLineItems, final MonetaryAmount totalPrice,
final TaxedPrice taxedPrice, final CartState cartState,
final Address shippingAddress, final Address billingAddress,
final InventoryMode inventoryMode, final Reference customerGroup,
final CountryCode country, final CartShippingInfo shippingInfo,
final List discountCodes, final CustomFields custom, final PaymentInfo paymentInfo) {
super(id, version, createdAt, lastModifiedAt);
this.customerId = customerId;
this.customerEmail = customerEmail;
this.lineItems = lineItems;
this.customLineItems = customLineItems;
this.totalPrice = totalPrice;
this.taxedPrice = taxedPrice;
this.cartState = cartState;
this.shippingAddress = shippingAddress;
this.billingAddress = billingAddress;
this.inventoryMode = inventoryMode;
this.customerGroup = customerGroup;
this.country = country;
this.shippingInfo = shippingInfo;
this.discountCodes = discountCodes;
this.custom = custom;
this.paymentInfo = paymentInfo;
}
@Override
@Nullable
public String getCustomerId() {
return customerId;
}
@Override
@Nullable
public String getCustomerEmail() {
return customerEmail;
}
@Override
public List getLineItems() {
return lineItems;
}
@Override
public List getCustomLineItems() {
return customLineItems;
}
@Override
public MonetaryAmount getTotalPrice() {
return totalPrice;
}
@Override
@Nullable
public TaxedPrice getTaxedPrice() {
return taxedPrice;
}
@Override
public CartState getCartState() {
return cartState;
}
@Override
@Nullable
public Address getShippingAddress() {
return shippingAddress;
}
@Override
@Nullable
public Address getBillingAddress() {
return billingAddress;
}
@Override
public InventoryMode getInventoryMode() {
return inventoryMode;
}
@Override
@Nullable
public Reference getCustomerGroup() {
return customerGroup;
}
@Override
@Nullable
public CountryCode getCountry() {
return country;
}
@Override
@Nullable
public CartShippingInfo getShippingInfo() {
return shippingInfo;
}
@Override
public List getDiscountCodes() {
return discountCodes;
}
@Override
@Nullable
public CustomFields getCustom() {
return custom;
}
@Override
@Nullable
public PaymentInfo getPaymentInfo() {
return paymentInfo;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy