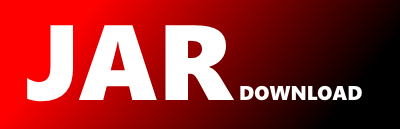
io.sphere.sdk.orders.OrderImpl Maven / Gradle / Ivy
package io.sphere.sdk.orders;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.neovisionaries.i18n.CountryCode;
import io.sphere.sdk.carts.*;
import io.sphere.sdk.customergroups.CustomerGroup;
import io.sphere.sdk.discountcodes.DiscountCodeInfo;
import io.sphere.sdk.models.Address;
import io.sphere.sdk.models.ResourceImpl;
import io.sphere.sdk.models.Reference;
import io.sphere.sdk.states.State;
import io.sphere.sdk.types.CustomFields;
import javax.annotation.Nullable;
import javax.money.MonetaryAmount;
import java.time.ZonedDateTime;
import java.util.List;
import java.util.Set;
final class OrderImpl extends ResourceImpl implements Order {
@Nullable
private final Address billingAddress;
@Nullable
private final CountryCode country;
@Nullable
private final String customerEmail;
@Nullable
private final Reference customerGroup;
@Nullable
private final String customerId;
private final List customLineItems;
private final InventoryMode inventoryMode;
private final Long lastMessageSequenceNumber;
private final List lineItems;
@Nullable
private final String orderNumber;
private final OrderState orderState;
private final List returnInfo;
@Nullable
private final ShipmentState shipmentState;
@Nullable
private final Address shippingAddress;
private final OrderShippingInfo shippingInfo;
private final Set syncInfo;
@Nullable
private final TaxedPrice taxedPrice;
private final MonetaryAmount totalPrice;
@Nullable
private final PaymentState paymentState;
@Nullable
private final ZonedDateTime completedAt;
private final List discountCodes;
@Nullable
private final Reference cart;
@Nullable
private final CustomFields custom;
@Nullable
private final Reference state;
@Nullable
private final PaymentInfo paymentInfo;
@JsonCreator
protected OrderImpl(final String id, final Long version, final ZonedDateTime createdAt, final ZonedDateTime lastModifiedAt, final Address billingAddress, final CountryCode country, final String customerEmail, final Reference customerGroup, final String customerId, final List customLineItems, final InventoryMode inventoryMode, final Long lastMessageSequenceNumber, final List lineItems, @Nullable final String orderNumber, final OrderState orderState, final List returnInfo, @Nullable final ShipmentState shipmentState, final Address shippingAddress, @Nullable final OrderShippingInfo shippingInfo, final Set syncInfo, final TaxedPrice taxedPrice, final MonetaryAmount totalPrice, @Nullable final PaymentState paymentState, @Nullable final ZonedDateTime completedAt, final List discountCodes, @Nullable final Reference cart, final CustomFields custom, final Reference state, @Nullable final PaymentInfo paymentInfo) {
super(id, version, createdAt, lastModifiedAt);
this.billingAddress = billingAddress;
this.country = country;
this.customerEmail = customerEmail;
this.customerGroup = customerGroup;
this.customerId = customerId;
this.customLineItems = customLineItems;
this.inventoryMode = inventoryMode;
this.lastMessageSequenceNumber = lastMessageSequenceNumber;
this.lineItems = lineItems;
this.orderNumber = orderNumber;
this.orderState = orderState;
this.returnInfo = returnInfo;
this.shipmentState = shipmentState;
this.shippingAddress = shippingAddress;
this.shippingInfo = shippingInfo;
this.syncInfo = syncInfo;
this.taxedPrice = taxedPrice;
this.totalPrice = totalPrice;
this.paymentState = paymentState;
this.completedAt = completedAt;
this.discountCodes = discountCodes;
this.cart = cart;
this.custom = custom;
this.state = state;
this.paymentInfo = paymentInfo;
}
@Override
@Nullable
public Address getBillingAddress() {
return billingAddress;
}
@Override
@Nullable
public CountryCode getCountry() {
return country;
}
@Override
@Nullable
public String getCustomerEmail() {
return customerEmail;
}
@Override
@Nullable
public Reference getCustomerGroup() {
return customerGroup;
}
@Override
@Nullable
public String getCustomerId() {
return customerId;
}
@Override
public List getCustomLineItems() {
return customLineItems;
}
@Override
public InventoryMode getInventoryMode() {
return inventoryMode;
}
@Override
public Long getLastMessageSequenceNumber() {
return lastMessageSequenceNumber;
}
@Override
public List getLineItems() {
return lineItems;
}
@Override
@Nullable
public String getOrderNumber() {
return orderNumber;
}
@Override
public OrderState getOrderState() {
return orderState;
}
@Override
public List getReturnInfo() {
return returnInfo;
}
@Override
@Nullable
public ShipmentState getShipmentState() {
return shipmentState;
}
@Override
@Nullable
public Address getShippingAddress() {
return shippingAddress;
}
@Override
public OrderShippingInfo getShippingInfo() {
return shippingInfo;
}
@Override
public Set getSyncInfo() {
return syncInfo;
}
@Override
@Nullable
public TaxedPrice getTaxedPrice() {
return taxedPrice;
}
@Override
public MonetaryAmount getTotalPrice() {
return totalPrice;
}
@Override
@Nullable
public PaymentState getPaymentState() {
return paymentState;
}
@Override
@Nullable
public ZonedDateTime getCompletedAt() {
return completedAt;
}
@Override
public List getDiscountCodes() {
return discountCodes;
}
@Nullable
@Override
public Reference getCart() {
return cart;
}
@Override
@Nullable
public CustomFields getCustom() {
return custom;
}
@Override
@Nullable
public Reference getState() {
return state;
}
@Override
@Nullable
public PaymentInfo getPaymentInfo() {
return paymentInfo;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy