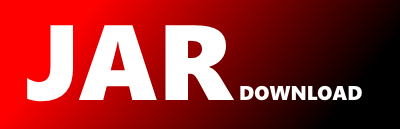
io.sphere.internal.OrderServiceImpl Maven / Gradle / Ivy
package io.sphere.internal;
import com.google.common.base.Function;
import io.sphere.client.*;
import io.sphere.client.exceptions.OutOfStockException;
import io.sphere.client.exceptions.PriceChangedException;
import io.sphere.client.exceptions.SphereBackendException;
import io.sphere.client.exceptions.SphereException;
import io.sphere.client.model.QueryResult;
import io.sphere.client.model.VersionedId;
import io.sphere.client.shop.ApiMode;
import io.sphere.client.shop.OrderService;
import io.sphere.client.shop.model.Order;
import io.sphere.client.shop.model.PaymentState;
import io.sphere.client.shop.model.ShipmentState;
import io.sphere.internal.command.CartCommands;
import io.sphere.internal.command.Command;
import io.sphere.internal.command.OrderCommands;
import io.sphere.internal.request.RequestFactory;
import com.google.common.base.Optional;
import org.codehaus.jackson.type.TypeReference;
import static io.sphere.internal.util.Util.*;
import javax.annotation.Nullable;
public class OrderServiceImpl implements OrderService {
private ProjectEndpoints endpoints;
private RequestFactory requestFactory;
public OrderServiceImpl(RequestFactory requestFactory, ProjectEndpoints endpoints) {
this.requestFactory = requestFactory;
this.endpoints = endpoints;
}
@Override public FetchRequest byId(String id) {
return requestFactory.createFetchRequest(
endpoints.orders.byId(id),
Optional.absent(),
new TypeReference() {});
}
@Override public QueryRequest all() {
return requestFactory.createQueryRequest(
endpoints.orders.root(),
Optional.absent(),
new TypeReference>() {});
}
@Override public QueryRequest forCustomer(String customerId) {
return requestFactory.createQueryRequest(
endpoints.orders.queryByCustomerId(customerId),
Optional.absent(),
new TypeReference>() {});
}
@Override public CommandRequest updatePaymentState(VersionedId orderId, PaymentState paymentState) {
return createCommandRequest(
endpoints.orders.updatePaymentState(),
new OrderCommands.UpdatePaymentState(orderId.getId(), orderId.getVersion(), paymentState));
}
@Override public CommandRequest updateShipmentState(VersionedId orderId, ShipmentState shipmentState) {
return createCommandRequest(
endpoints.orders.updateShipmentState(),
new OrderCommands.UpdateShipmentState(orderId.getId(), orderId.getVersion(), shipmentState));
}
@Override public CommandRequest createOrder(VersionedId cartId, PaymentState paymentState) {
return requestFactory.createCommandRequest(
endpoints.orders.root(),
new CartCommands.OrderCart(cartId.getId(), cartId.getVersion(), paymentState),
new TypeReference() {}).
withErrorHandling(new Function() {
public SphereException apply(@Nullable SphereBackendException e) {
SphereError.OutOfStock outOfStockError = getError(e, SphereError.OutOfStock.class);
if (outOfStockError != null)
return new OutOfStockException(outOfStockError.getLineItemIds());
SphereError.PriceChanged priceChangedError = getError(e, SphereError.PriceChanged.class);
if (priceChangedError != null)
return new PriceChangedException(priceChangedError.getLineItemIds());
return null;
}
});
}
@Override public CommandRequest createOrder(VersionedId cartId) {
return createOrder(cartId, null);
}
/** Helper to save some repetitive code. */
private CommandRequest createCommandRequest(String url, Command command) {
return requestFactory.createCommandRequest(url, command, new TypeReference() {});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy