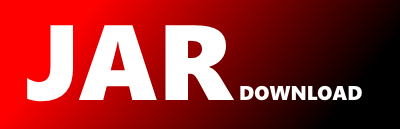
io.sphere.internal.CategoryCache Maven / Gradle / Ivy
package io.sphere.internal;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import io.sphere.client.shop.model.Category;
import java.util.*;
public class CategoryCache {
private ImmutableList roots;
private ImmutableList all;
private ImmutableMap byIdMap;
private ImmutableMap bySlugMap;
private CategoryCache(
ImmutableList roots,
ImmutableList all,
ImmutableMap categoriesById,
ImmutableMap categoriesBySlug) {
this.roots = roots;
this.byIdMap = categoriesById;
this.bySlugMap = categoriesBySlug;
this.all = all;
}
/** Caches category tree in multiple different ways for fast lookup. */
public static CategoryCache create(Iterable roots, Locale locale) {
List all = getAllRecursive(roots);
return new CategoryCache(ImmutableList.copyOf(roots), sortByName(all, locale), buildByIdMap(all), buildBySlugMap(all, locale));
}
public List getRoots() { return roots; }
public Category getById(String id) { return byIdMap.get(id); }
public Category getBySlug(String slug) { return bySlugMap.get(slug); }
public List getAsFlatList() { return all; }
// --------------------------------------------------
// Helpers for create()
// --------------------------------------------------
private static List getAllRecursive(Iterable categories) {
List result = new ArrayList();
for (Category c: categories) {
result.add(c);
for (Category child: getAllRecursive(c.getChildren())) {
result.add(child);
}
}
return result;
}
private static ImmutableMap buildByIdMap(Collection categories) {
Map map = new HashMap(categories.size());
for (Category c: categories) {
map.put(c.getId(), c);
}
return ImmutableMap.copyOf(map);
}
private static ImmutableMap buildBySlugMap(Collection categories, Locale locale) {
Map map = new HashMap(categories.size());
for (Category category: categories) {
map.put(category.getSlug(locale), category);
}
return ImmutableMap.copyOf(map);
}
private static ImmutableList sortByName(Collection categories, Locale locale) {
List categoriesCopy = new ArrayList(categories);
Collections.sort(categoriesCopy, new ByNameComparator(locale));
return ImmutableList.copyOf(categoriesCopy);
}
private static class ByNameComparator implements Comparator {
private final Locale locale;
private ByNameComparator(Locale locale) { this.locale = locale; }
@Override public int compare(Category category, Category other) {
if (category == null && other == null) return 0;
if (category == null && other != null) return -1;
if (category != null && other == null) return 1;
return category.getName(locale).compareTo(other.getName(locale));
}
/** Indicates whether some other object is equal to this comparator. */
@Override public boolean equals(Object other) {
return this == other;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy