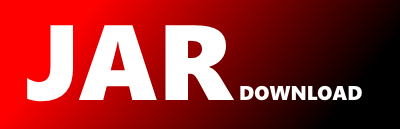
io.sphere.client.QueryRequest Maven / Gradle / Ivy
package io.sphere.client;
import com.google.common.util.concurrent.ListenableFuture;
import io.sphere.client.model.QueryResult;
/** Request that uses a Sphere query API to fetch objects satisfying some conditions. */
public interface QueryRequest {
/** Executes the request and returns the result. */
QueryResult fetch();
/** Executes the request asynchronously and returns a future providing the result. */
ListenableFuture> fetchAsync();
/**
* Sets the predicate used to filter the results for this query request.
* @param predicate The predicate which is used to filter the results.
* Example: "name(en=\"myName\")"
.
**/
QueryRequest where(String predicate);
/**
* Sets the sort expression for sorting the result.
* @param fieldName the attribute to sort with
* Example: "name.en"
.
* @param sortDirection The direction to sort with.
**/
QueryRequest sort(String fieldName, SortDirection sortDirection);
/** Sets the page number for paging through results. Page numbers start at zero. */
QueryRequest page(int page);
/** Sets the size of a page for paging through results. When page size is not set, the default of 10 is used. */
QueryRequest pageSize(int pageSize);
/** Requests {@linkplain io.sphere.client.model.Reference Reference fields} to be expanded in the returned objects.
* Expanded references contain full target objects they link to.
*
* As an illustration, here is how reference expansion looks at the underlying JSON transport level.
* Given a customer object:
*
{@code
*{
* "name": "John Doe"
* "customerGroup": {
* "typeId": "customer-group",
* "id": "7ba61480-6a72-4a2a-a72e-cd39f75a7ef2"
* }
*}}
*
* This is what the result looks like when the path 'customerGroup' has been expanded:
*
*{@code
*{
* "name": "John Doe"
* "customerGroup": {
* typeId: "customer-group",
* id: "7ba61480-6a72-4a2a-a72e-cd39f75a7ef2"
* obj: {
* "name": "Gold"
* }
* }
*}}
*
* @param paths The paths to be expanded, such as 'customerGroup'. */
QueryRequest expand(String... paths);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy