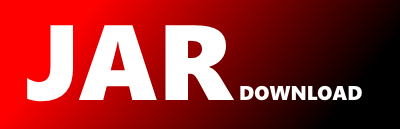
io.sphere.internal.util.ListUtil Maven / Gradle / Ivy
package io.sphere.internal.util;
import com.google.common.collect.FluentIterable;
import com.google.common.collect.ImmutableList;
import io.sphere.internal.util.SearchUtil;
import java.util.List;
import java.util.Random;
public class ListUtil {
/** Helper for vararg methods with at least one argument. */
public static ImmutableList list(T t, T... ts) {
ImmutableList.Builder builder = ImmutableList.builder();
if (t != null) builder = builder.add(t);
for (T elem: ts) {
if (elem != null) builder.add(elem);
}
return builder.build();
}
/** Creates an copy immutable copy of given collection with given element prepended. */
public static ImmutableList list(T t, Iterable elems) {
ImmutableList.Builder builder = ImmutableList.builder();
if (t != null) builder = builder.add(t);
for (T e: elems) {
if (e != null) builder.add(e);
}
return builder.build();
}
/** Creates an copy immutable copy of given collection with given element prepended. */
public static ImmutableList list(Iterable elems1, Iterable elems2) {
ImmutableList.Builder builder = ImmutableList.builder();
for (T e: elems1) {
if (e != null) builder.add(e);
}
for (T e: elems2) {
if (e != null) builder.add(e);
}
return builder.build();
}
/** Converts a Collection to a List. */
public static ImmutableList toList(Iterable elems) {
if (elems == null) {
return ImmutableList.of();
}
if (elems instanceof ImmutableList) {
return (ImmutableList)elems;
}
return ImmutableList.copyOf(FluentIterable.from(elems).filter(Util.isNotNull));
}
private static final ThreadLocal random = new ThreadLocal() {
@Override protected Random initialValue() {
return new Random();
}
};
/** Selects a random element from a list. */
public static T randomElement(List list) {
if (list.isEmpty()) throw new IllegalArgumentException("Can't select random element from an empty list.");
return list.get(random.get().nextInt(list.size()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy