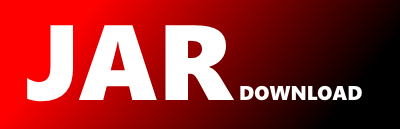
io.sphere.internal.command.ChannelCommands Maven / Gradle / Ivy
package io.sphere.internal.command;
import com.google.common.collect.Sets;
import io.sphere.client.shop.model.ChannelRoles;
import org.codehaus.jackson.map.annotate.JsonSerialize;
import java.util.Set;
public class ChannelCommands {
public static abstract class ChannelUpdateAction extends UpdateAction {
public ChannelUpdateAction(final String action) {
super(action);
}
}
public static class ChangeKey extends ChannelUpdateAction {
private final String key;
public ChangeKey(final String key) {
super("changeKey");
this.key = key;
}
public String getKey() {
return key;
}
}
public static class AddRoles extends ChannelUpdateAction {
private final Set roles;
public AddRoles(final Set roles) {
super("addRoles");
this.roles = roles;
}
public Set getRoles() {
return roles;
}
}
public static class RemoveRoles extends ChannelUpdateAction {
private final Set roles;
public RemoveRoles(final Set roles) {
super("removeRoles");
this.roles = roles;
}
public Set getRoles() {
return roles;
}
}
public static class SetRoles extends ChannelUpdateAction {
private final Set roles;
public SetRoles(final Set roles) {
super("setRoles");
this.roles = roles;
}
public Set getRoles() {
return roles;
}
}
public static class CreateChannel implements Command {
private final String key;
@JsonSerialize(include = JsonSerialize.Inclusion.NON_EMPTY)
private final Set roles;
public CreateChannel(final String key, final Set roles) {
this.key = key;
this.roles = roles;
}
public CreateChannel(final String key) {
this(key, Sets.newHashSet());
}
public String getKey() {
return key;
}
public Set getRoles() {
return roles;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy