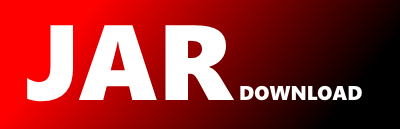
com.netflix.spinnaker.cats.test.ManualRunnableScheduler.groovy Maven / Gradle / Ivy
/*
* Copyright 2014 Netflix, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.netflix.spinnaker.cats.test
import java.util.concurrent.*
class ManualRunnableScheduler implements ScheduledExecutorService {
private final Collection> callables = new LinkedList<>()
private static class RunnableWrapper implements Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy