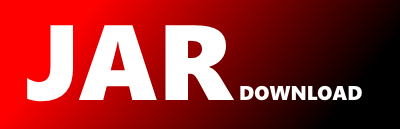
com.netflix.spinnaker.keel.verification.ImageFinder.kt Maven / Gradle / Ivy
package com.netflix.spinnaker.keel.verification
import com.netflix.spinnaker.keel.api.DeliveryConfig
import com.netflix.spinnaker.keel.api.Resource
import com.netflix.spinnaker.keel.api.ResourceSpec
import com.netflix.spinnaker.keel.api.plugins.BaseClusterHandler
import com.netflix.spinnaker.keel.api.plugins.CurrentImages
import kotlinx.coroutines.runBlocking
import org.slf4j.LoggerFactory
import org.springframework.stereotype.Component
@Component
class ImageFinder(
val clusterHandlers: List>
) {
private val log by lazy { LoggerFactory.getLogger(javaClass) }
/**
* Find images that are currently running in an environment and returns them
*/
fun getImages(deliveryConfig: DeliveryConfig, envName: String): List {
val env = checkNotNull(deliveryConfig.environments.find { it.name == envName }) {
"Failed to find environment $envName in deliveryConfig ${deliveryConfig.name}"
}
return env.resources.mapNotNull { resource ->
runBlocking { getImages(resource) }
}
}
private suspend fun getImages(resource: Resource<*>): CurrentImages? =
clusterHandlers
.find { it.supportedKind.kind == resource.kind }
?.getImages(resource)
@Suppress("UNCHECKED_CAST")
private suspend fun BaseClusterHandler.getImages(
resource: Resource<*>
): CurrentImages =
getImage(resource as Resource)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy