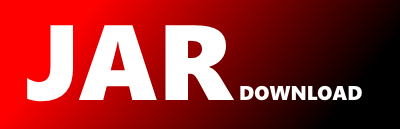
io.split.qos.server.failcondition.SimpleFailCondition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qosrunner Show documentation
Show all versions of qosrunner Show documentation
Framework for running JUnit Tests as a Continous Service (QoS)
package io.split.qos.server.failcondition;
import com.google.common.base.Preconditions;
import com.google.common.collect.ConcurrentHashMultiset;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.common.collect.Multiset;
import com.google.inject.Inject;
import com.google.inject.Singleton;
import com.google.inject.name.Named;
import io.split.qos.server.modules.QOSPropertiesModule;
import io.split.qos.server.util.TestId;
import java.util.Map;
import java.util.concurrent.TimeUnit;
/**
* Simple implementation of a failcondition.
*
* It takes into account two things, how many consecutive failures have to happen before broadcasting the failure.
* And how much time until the same failure gets broadcasted.
*/
@Singleton
public class SimpleFailCondition implements FailCondition {
//Each Tests starts its own injector, so each test brings up its own Broadcaster.
//Could inject this in QOSCommonModule instead so there is only one Broadcaster, haven't tried
//For now these has to be static.
private static final Multiset FAILURES = ConcurrentHashMultiset.create();
private static final Map FIRST_FAILURE_TIME = Maps.newConcurrentMap();
private static final Map FAILURE_MULTIPLIER = Maps.newConcurrentMap();
private final int consecutiveFailures;
private final Integer reBroadcastFailureInMinutes;
@Inject
public SimpleFailCondition(
@Named(QOSPropertiesModule.CONSECUTIVE_FAILURES) String consecutiveFailures,
@Named(QOSPropertiesModule.RE_BROADCAST_FAILURE_IN_MINUTES) String reBroadastFailureInMinutes) {
this.consecutiveFailures = Integer.valueOf(Preconditions.checkNotNull(consecutiveFailures));
this.reBroadcastFailureInMinutes = Integer.valueOf(Preconditions.checkNotNull(reBroadastFailureInMinutes));
}
@Override
public Broadcast failed(TestId testId) {
Preconditions.checkNotNull(testId);
FAILURES.add(testId);
if (FAILURES.count(testId) == consecutiveFailures) {
FIRST_FAILURE_TIME.put(testId, System.currentTimeMillis());
return Broadcast.FIRST;
}
Long when = FIRST_FAILURE_TIME.get(testId);
int mulitiplier = FAILURE_MULTIPLIER.getOrDefault(testId, 1);
if (when != null && (when + mulitiplier * TimeUnit.MINUTES.toMillis(reBroadcastFailureInMinutes)) < System.currentTimeMillis()) {
mulitiplier++;
FAILURE_MULTIPLIER.put(testId, mulitiplier);
return Broadcast.REBROADCAST;
}
return Broadcast.NO;
}
@Override
public Broadcast success(TestId testId) {
Preconditions.checkNotNull(testId);
int count = FAILURES.count(testId);
FIRST_FAILURE_TIME.remove(testId);
FAILURE_MULTIPLIER.put(testId, 1);
FAILURES.removeAll(Lists.newArrayList(testId));
if (count >= consecutiveFailures) {
return Broadcast.RECOVERY;
} else {
return Broadcast.NO;
}
}
@Override
public Long firstFailure(TestId testId) {
return FIRST_FAILURE_TIME.get(testId);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy