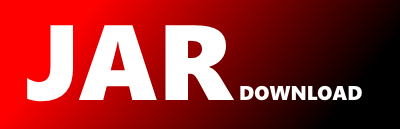
io.springlets.data.domain.jaxb.IterableAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of springlets-data-commons Show documentation
Show all versions of springlets-data-commons Show documentation
Springlets for Spring Data Commons
/*
* Copyright 2016 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.springlets.data.domain.jaxb;
import java.util.AbstractCollection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import javax.xml.bind.annotation.adapters.XmlAdapter;
/**
* JAXB {@link XmlAdapter} to convert {@link Iterable} instances
* into a {@link Collection}s and vice versa.
*
* @author Enrique Ruiz at http://www.disid.com[DISID Corporation S.L.]
*/
public class IterableAdapter extends XmlAdapter, Iterable> {
@Override
@SuppressWarnings({"unchecked", "rawtypes"})
public AbstractCollection marshal(Iterable source) throws Exception {
if (source == null) {
return (AbstractCollection) Collections.emptyList();
}
if (source instanceof AbstractCollection) {
return (AbstractCollection) source;
}
return makeCollection(source);
}
@Override
public Iterable unmarshal(AbstractCollection source) throws Exception {
if (source == null || source.isEmpty()) {
return Collections.emptyList();
}
return source;
}
/**
* Creates a mutable {@link ArrayList} instance containing the given elements.
*
* @param source Iterable
* @return Collection
*/
protected AbstractCollection makeCollection(Iterable source) {
AbstractCollection result = new ArrayList();
for (S element : source) {
try {
result.add(element);
}
catch (Exception o_O) {
throw new RuntimeException(o_O);
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy