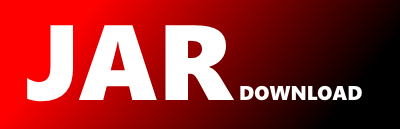
io.sprucehill.telize.model.GeoIPInformation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of telize-geoip-java-api-wrapper Show documentation
Show all versions of telize-geoip-java-api-wrapper Show documentation
A Java API wrapper for the Telize REST API
The newest version!
/*
Copyright 2015 SpruceHill.io GmbH
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.sprucehill.telize.model;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* A model object for the GeoIP query response
*
* @author Michael Duergner
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class GeoIPInformation {
@JsonProperty(value = "ip")
String ip;
@JsonProperty(value = "country_code")
String countryCode;
@JsonProperty(value = "country_code3")
String countryCode3;
@JsonProperty(value = "country")
String country;
@JsonProperty(value = "region_code")
String regionCode;
@JsonProperty(value = "region")
String region;
@JsonProperty(value = "city")
String city;
@JsonProperty(value = "postal_code")
String postalCode;
@JsonProperty(value = "continent_code")
String continentCode;
@JsonProperty(value = "latitude")
Double latitude;
@JsonProperty(value = "longitude")
Double longitude;
@JsonProperty(value = "dma_code")
String dmaCode;
@JsonProperty(value = "area_code")
String areaCode;
@JsonProperty(value = "asn")
String asn;
@JsonProperty(value = "isp")
String isp;
@JsonProperty(value = "timezone")
String timezone;
/**
* Get the IP address queried
*
* @return A string being the representation of the IP
*/
public String getIp() {
return ip;
}
/**
* Get the country code (ISO 3166-1 alpha-2) for the IP address queried
*
* @return A string being the country code for the IP or null if not available
*/
public String getCountryCode() {
return countryCode;
}
/**
* Get the country code (ISO 3166-1 alpha-3) for the IP address queried
*
* @return A string being the country code for the IP or null if not available
*/
public String getCountryCode3() {
return countryCode3;
}
/**
* Get the name of the country for the IP address queried
*
* @return A string being the name of the country for the IP or null if not available
*/
public String getCountry() {
return country;
}
/**
* Get the region code (ISO-3166-2) for the IP address queried
*
* @return A string being the region code for the IP or null if not available
*/
public String getRegionCode() {
return regionCode;
}
/**
* Get the region name for the IP address queried
*
* @return A string being the region name for the IP or null if not available
*/
public String getRegion() {
return region;
}
/**
* Get the city for the IP address queried
*
* @return A string being the city for the IP or null if not available
*/
public String getCity() {
return city;
}
/**
* Get the postal code for the IP address queried
*
* @return A string being the postal code for the IP or null if not available
*/
public String getPostalCode() {
return postalCode;
}
/**
* Get the continent code for the IP address queried
*
* @return A string being the continent code for the IP or null if not available
*/
public String getContinentCode() {
return continentCode;
}
/**
* Get the latitude for the IP address queried
*
* @return A double being the latitude for the IP or null if not available
*/
public Double getLatitude() {
return latitude;
}
/**
* Get the longitude for the IP address queried
*
* @return A double being the longitude for the IP or null if not available
*/
public Double getLongitude() {
return longitude;
}
/**
* Get the DMA code for the IP address queried
*
* @return A string being the DMA code for the IP or null if not available
*/
public String getDmaCode() {
return dmaCode;
}
/**
* Get the area code for the IP address queried
*
* @return A string being the area code for the IP or null if not available
*/
public String getAreaCode() {
return areaCode;
}
/**
* Get the ASN for the IP address queried
*
* @return A string being the ASN for the IP or null if not available
*/
public String getAsn() {
return asn;
}
/**
* Get the ISP for the IP address queried
*
* @return A string being the ISP for the IP or null if not available
*/
public String getIsp() {
return isp;
}
/**
* Get the timezone for the IP addres queried
*
* @return A string being the timezone for the IP or null if not available
*/
public String getTimezone() {
return timezone;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("GeoIPInformation{");
sb.append("ip='").append(ip).append('\'');
sb.append(", countryCode='").append(countryCode).append('\'');
sb.append(", countryCode3='").append(countryCode3).append('\'');
sb.append(", country='").append(country).append('\'');
sb.append(", regionCode='").append(regionCode).append('\'');
sb.append(", region='").append(region).append('\'');
sb.append(", city='").append(city).append('\'');
sb.append(", postalCode='").append(postalCode).append('\'');
sb.append(", continentCode='").append(continentCode).append('\'');
sb.append(", latitude=").append(latitude);
sb.append(", longitude=").append(longitude);
sb.append(", dmaCode='").append(dmaCode).append('\'');
sb.append(", areaCode='").append(areaCode).append('\'');
sb.append(", asn='").append(asn).append('\'');
sb.append(", isp='").append(isp).append('\'');
sb.append(", timezone='").append(timezone).append('\'');
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy