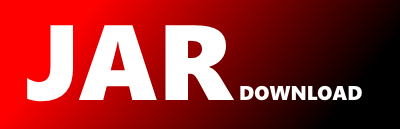
io.sprucehill.telize.service.TelizeGeoIPService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of telize-geoip-java-api-wrapper Show documentation
Show all versions of telize-geoip-java-api-wrapper Show documentation
A Java API wrapper for the Telize REST API
The newest version!
/*
Copyright 2015 SpruceHill.io GmbH
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package io.sprucehill.telize.service;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.sprucehill.telize.model.GeoIPInformation;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.HttpClientBuilder;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.PostConstruct;
import java.io.IOException;
import java.net.Inet4Address;
import java.net.Inet6Address;
/**
* Service implementation for the ITelizeGeoIPService interface
*
* @author Michael Duergner
*/
public class TelizeGeoIPService implements ITelizeGeoIPService {
protected Logger logger = LoggerFactory.getLogger(getClass());
protected HttpClient httpClient;
protected ObjectMapper objectMapper;
/**
* Set the HttpClient to use; if you don't set one, invoking the postConstruct() method will create a default
* implementation
*
* @param httpClient The HttpClient to use
*/
public void setHttpClient(HttpClient httpClient) {
this.httpClient = httpClient;
}
/**
* Set the ObjectMapper to use; if you don't set one, invoking the postConstruct() method will create one
*
* @param objectMapper The ObjectMapper to use
*/
public void setObjectMapper(ObjectMapper objectMapper) {
this.objectMapper = objectMapper;
}
/**
* Create a TelizeGeoIPService without submitting HttpClient or ObjectMapper; you can use setter injection or let
* the postConstruct() method create them with default implementations.
*/
public TelizeGeoIPService() {}
/**
* Create a TelizeGeoIPService with the supplied HttpClient; use setter injection for the ObjectMapper or let the
* postConstruct() method create it with a default implementation.
*
* @param httpClient The HttpClient to use
*/
public TelizeGeoIPService(HttpClient httpClient) {
this.httpClient = httpClient;
}
/**
* Create a TelizeGeoIPService with the supplied ObjectMapper; use setter injection for the HttpClient or let the
* postConstruct() method create it with a default implementation
*
* @param objectMapper The ObjectMapper to use
*/
public TelizeGeoIPService(ObjectMapper objectMapper) {
this.objectMapper = objectMapper;
}
/**
* Create a TelizeGeoIPService with the supplied HttpClient and ObjectMapper
*
* @param httpClient The HttpClient to use
* @param objectMapper The ObjectMapper to use
*/
public TelizeGeoIPService(HttpClient httpClient, ObjectMapper objectMapper) {
this.httpClient = httpClient;
this.objectMapper = objectMapper;
}
/**
* If you're not using this a part of a container implementation which will call @PostContruct annotated methods
* itself, make sure to call it after creating this bean to ensure it's fully initialized
*/
@PostConstruct
public void postConstruct() {
if (null == httpClient) {
httpClient = HttpClientBuilder.create().build();
}
if (null == objectMapper) {
objectMapper = new ObjectMapper();
}
}
@Override
public GeoIPInformation my() {
return query(null);
}
@Override
public GeoIPInformation ipv4(Inet4Address ipv4) {
return query(ipv4.getHostAddress());
}
@Override
public GeoIPInformation ipv6(Inet6Address ipv6) {
return query(ipv6.getHostAddress());
}
protected GeoIPInformation query(String ip) {
HttpGet request = null;
try {
String url = "http://www.telize.com/geoip" + (null != ip ? "/" + ip : "");
request = new HttpGet(url);
HttpResponse response = httpClient.execute(request);
if (200 == response.getStatusLine().getStatusCode()) {
return objectMapper.readValue(response.getEntity().getContent(),GeoIPInformation.class);
}
else if (400 == response.getStatusLine().getStatusCode()) {
logger.warn("Got an 'Invalid IP address' response from Telize - that should never happen");
throw new RuntimeException("Invalid IP address submitted");
}
else {
logger.warn("Got an unexpected status code '{}' from Telize",response.getStatusLine().getStatusCode());
throw new RuntimeException("We encountered and error while processing your request");
}
} catch (IOException e) {
logger.error(e.getMessage(),e);
throw new RuntimeException(e.getMessage(),e);
} finally {
if (null != request && !request.isAborted()) {
request.abort();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy