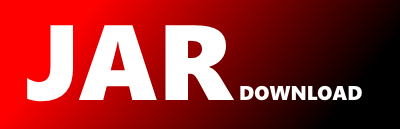
io.stargate.auth.ImmutableAuthenticationSubject Maven / Gradle / Ivy
package io.stargate.auth;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link AuthenticationSubject}.
*
* Use the builder to create immutable instances:
* {@code ImmutableAuthenticationSubject.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableAuthenticationSubject.of()}.
*/
@Generated(from = "AuthenticationSubject", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableAuthenticationSubject implements AuthenticationSubject {
private final @Nullable String token;
private final String roleName;
private final boolean isFromExternalAuth;
private final Map customProperties;
private ImmutableAuthenticationSubject(
@Nullable String token,
String roleName,
boolean isFromExternalAuth,
Map customProperties) {
this.token = token;
this.roleName = Objects.requireNonNull(roleName, "roleName");
this.isFromExternalAuth = isFromExternalAuth;
this.customProperties = Objects.requireNonNull(customProperties, "customProperties");
}
private ImmutableAuthenticationSubject(
ImmutableAuthenticationSubject original,
@Nullable String token,
String roleName,
boolean isFromExternalAuth,
Map customProperties) {
this.token = token;
this.roleName = roleName;
this.isFromExternalAuth = isFromExternalAuth;
this.customProperties = customProperties;
}
/**
* @return The value of the {@code token} attribute
*/
@Override
public @Nullable String token() {
return token;
}
/**
* @return The value of the {@code roleName} attribute
*/
@Override
public String roleName() {
return roleName;
}
/**
* @return The value of the {@code isFromExternalAuth} attribute
*/
@Override
public boolean isFromExternalAuth() {
return isFromExternalAuth;
}
/**
* @return The value of the {@code customProperties} attribute
*/
@Override
public Map customProperties() {
return customProperties;
}
/**
* Copy the current immutable object by setting a value for the {@link AuthenticationSubject#token() token} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for token (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAuthenticationSubject withToken(@Nullable String value) {
if (Objects.equals(this.token, value)) return this;
return new ImmutableAuthenticationSubject(this, value, this.roleName, this.isFromExternalAuth, this.customProperties);
}
/**
* Copy the current immutable object by setting a value for the {@link AuthenticationSubject#roleName() roleName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for roleName
* @return A modified copy of the {@code this} object
*/
public final ImmutableAuthenticationSubject withRoleName(String value) {
String newValue = Objects.requireNonNull(value, "roleName");
if (this.roleName.equals(newValue)) return this;
return new ImmutableAuthenticationSubject(this, this.token, newValue, this.isFromExternalAuth, this.customProperties);
}
/**
* Copy the current immutable object by setting a value for the {@link AuthenticationSubject#isFromExternalAuth() isFromExternalAuth} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isFromExternalAuth
* @return A modified copy of the {@code this} object
*/
public final ImmutableAuthenticationSubject withIsFromExternalAuth(boolean value) {
if (this.isFromExternalAuth == value) return this;
return new ImmutableAuthenticationSubject(this, this.token, this.roleName, value, this.customProperties);
}
/**
* Copy the current immutable object by setting a value for the {@link AuthenticationSubject#customProperties() customProperties} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for customProperties
* @return A modified copy of the {@code this} object
*/
public final ImmutableAuthenticationSubject withCustomProperties(Map value) {
if (this.customProperties == value) return this;
Map newValue = Objects.requireNonNull(value, "customProperties");
return new ImmutableAuthenticationSubject(this, this.token, this.roleName, this.isFromExternalAuth, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableAuthenticationSubject} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableAuthenticationSubject
&& equalTo((ImmutableAuthenticationSubject) another);
}
private boolean equalTo(ImmutableAuthenticationSubject another) {
return Objects.equals(token, another.token)
&& roleName.equals(another.roleName)
&& isFromExternalAuth == another.isFromExternalAuth
&& customProperties.equals(another.customProperties);
}
/**
* Computes a hash code from attributes: {@code token}, {@code roleName}, {@code isFromExternalAuth}, {@code customProperties}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(token);
h += (h << 5) + roleName.hashCode();
h += (h << 5) + Boolean.hashCode(isFromExternalAuth);
h += (h << 5) + customProperties.hashCode();
return h;
}
/**
* Prints the immutable value {@code AuthenticationSubject} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "AuthenticationSubject{"
+ "token=" + token
+ ", roleName=" + roleName
+ ", isFromExternalAuth=" + isFromExternalAuth
+ ", customProperties=" + customProperties
+ "}";
}
/**
* Construct a new immutable {@code AuthenticationSubject} instance.
* @param token The value for the {@code token} attribute
* @param roleName The value for the {@code roleName} attribute
* @param isFromExternalAuth The value for the {@code isFromExternalAuth} attribute
* @param customProperties The value for the {@code customProperties} attribute
* @return An immutable AuthenticationSubject instance
*/
public static ImmutableAuthenticationSubject of(@Nullable String token, String roleName, boolean isFromExternalAuth, Map customProperties) {
return new ImmutableAuthenticationSubject(token, roleName, isFromExternalAuth, customProperties);
}
/**
* Creates an immutable copy of a {@link AuthenticationSubject} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable AuthenticationSubject instance
*/
public static ImmutableAuthenticationSubject copyOf(AuthenticationSubject instance) {
if (instance instanceof ImmutableAuthenticationSubject) {
return (ImmutableAuthenticationSubject) instance;
}
return ImmutableAuthenticationSubject.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableAuthenticationSubject ImmutableAuthenticationSubject}.
*
* ImmutableAuthenticationSubject.builder()
* .token(String | null) // nullable {@link AuthenticationSubject#token() token}
* .roleName(String) // required {@link AuthenticationSubject#roleName() roleName}
* .isFromExternalAuth(boolean) // required {@link AuthenticationSubject#isFromExternalAuth() isFromExternalAuth}
* .customProperties(Map<String, String>) // required {@link AuthenticationSubject#customProperties() customProperties}
* .build();
*
* @return A new ImmutableAuthenticationSubject builder
*/
public static ImmutableAuthenticationSubject.Builder builder() {
return new ImmutableAuthenticationSubject.Builder();
}
/**
* Builds instances of type {@link ImmutableAuthenticationSubject ImmutableAuthenticationSubject}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "AuthenticationSubject", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_ROLE_NAME = 0x1L;
private static final long INIT_BIT_IS_FROM_EXTERNAL_AUTH = 0x2L;
private static final long INIT_BIT_CUSTOM_PROPERTIES = 0x4L;
private long initBits = 0x7L;
private @Nullable String token;
private @Nullable String roleName;
private boolean isFromExternalAuth;
private @Nullable Map customProperties;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code AuthenticationSubject} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AuthenticationSubject instance) {
Objects.requireNonNull(instance, "instance");
@Nullable String tokenValue = instance.token();
if (tokenValue != null) {
token(tokenValue);
}
roleName(instance.roleName());
isFromExternalAuth(instance.isFromExternalAuth());
customProperties(instance.customProperties());
return this;
}
/**
* Initializes the value for the {@link AuthenticationSubject#token() token} attribute.
* @param token The value for token (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder token(@Nullable String token) {
this.token = token;
return this;
}
/**
* Initializes the value for the {@link AuthenticationSubject#roleName() roleName} attribute.
* @param roleName The value for roleName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder roleName(String roleName) {
this.roleName = Objects.requireNonNull(roleName, "roleName");
initBits &= ~INIT_BIT_ROLE_NAME;
return this;
}
/**
* Initializes the value for the {@link AuthenticationSubject#isFromExternalAuth() isFromExternalAuth} attribute.
* @param isFromExternalAuth The value for isFromExternalAuth
* @return {@code this} builder for use in a chained invocation
*/
public final Builder isFromExternalAuth(boolean isFromExternalAuth) {
this.isFromExternalAuth = isFromExternalAuth;
initBits &= ~INIT_BIT_IS_FROM_EXTERNAL_AUTH;
return this;
}
/**
* Initializes the value for the {@link AuthenticationSubject#customProperties() customProperties} attribute.
* @param customProperties The value for customProperties
* @return {@code this} builder for use in a chained invocation
*/
public final Builder customProperties(Map customProperties) {
this.customProperties = Objects.requireNonNull(customProperties, "customProperties");
initBits &= ~INIT_BIT_CUSTOM_PROPERTIES;
return this;
}
/**
* Builds a new {@link ImmutableAuthenticationSubject ImmutableAuthenticationSubject}.
* @return An immutable instance of AuthenticationSubject
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableAuthenticationSubject build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableAuthenticationSubject(null, token, roleName, isFromExternalAuth, customProperties);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ROLE_NAME) != 0) attributes.add("roleName");
if ((initBits & INIT_BIT_IS_FROM_EXTERNAL_AUTH) != 0) attributes.add("isFromExternalAuth");
if ((initBits & INIT_BIT_CUSTOM_PROPERTIES) != 0) attributes.add("customProperties");
return "Cannot build AuthenticationSubject, some of required attributes are not set " + attributes;
}
}
}