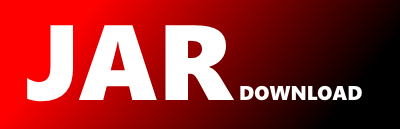
io.stargate.db.schema.ImmutableMaterializedView Maven / Gradle / Ivy
package io.stargate.db.schema;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.io.ObjectStreamException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link MaterializedView}.
*
* Use the builder to create immutable instances:
* {@code ImmutableMaterializedView.builder()}.
*/
@Generated(from = "MaterializedView", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ImmutableMaterializedView extends MaterializedView {
private final String name;
private final String keyspace;
private final List columns;
private final int hashCode;
private ImmutableMaterializedView(
String name,
String keyspace,
List columns) {
this.name = name;
this.keyspace = keyspace;
this.columns = columns;
this.hashCode = computeHashCode();
}
/**
* @return The value of the {@code name} attribute
*/
@Override
public String name() {
return name;
}
/**
* @return The value of the {@code keyspace} attribute
*/
@Override
public String keyspace() {
return keyspace;
}
/**
* @return The value of the {@code columns} attribute
*/
@Override
public List columns() {
return columns;
}
/**
* Copy the current immutable object by setting a value for the {@link MaterializedView#name() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableMaterializedView withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableMaterializedView(newValue, this.keyspace, this.columns);
}
/**
* Copy the current immutable object by setting a value for the {@link MaterializedView#keyspace() keyspace} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for keyspace
* @return A modified copy of the {@code this} object
*/
public final ImmutableMaterializedView withKeyspace(String value) {
String newValue = Objects.requireNonNull(value, "keyspace");
if (this.keyspace.equals(newValue)) return this;
return new ImmutableMaterializedView(this.name, newValue, this.columns);
}
/**
* Copy the current immutable object with elements that replace the content of {@link MaterializedView#columns() columns}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableMaterializedView withColumns(Column... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableMaterializedView(this.name, this.keyspace, newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link MaterializedView#columns() columns}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of columns elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableMaterializedView withColumns(Iterable extends Column> elements) {
if (this.columns == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableMaterializedView(this.name, this.keyspace, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableMaterializedView} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableMaterializedView
&& equalTo((ImmutableMaterializedView) another);
}
private boolean equalTo(ImmutableMaterializedView another) {
if (hashCode != another.hashCode) return false;
return name.equals(another.name)
&& keyspace.equals(another.keyspace)
&& columns.equals(another.columns);
}
/**
* Returns a precomputed-on-construction hash code from attributes: {@code name}, {@code keyspace}, {@code columns}.
* @return hashCode value
*/
@Override
public int hashCode() {
return hashCode;
}
private int computeHashCode() {
int h = 5381;
h += (h << 5) + name.hashCode();
h += (h << 5) + keyspace.hashCode();
h += (h << 5) + columns.hashCode();
return h;
}
/**
* Prints the immutable value {@code MaterializedView} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "MaterializedView{"
+ "name=" + name
+ ", keyspace=" + keyspace
+ ", columns=" + columns
+ "}";
}
private transient volatile long lazyInitBitmap;
private static final long CQL_NAME_LAZY_INIT_BIT = 0x1L;
private transient String cqlName;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#cqlName() cqlName} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code cqlName} attribute
*/
@Override
public String cqlName() {
if ((lazyInitBitmap & CQL_NAME_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & CQL_NAME_LAZY_INIT_BIT) == 0) {
this.cqlName = Objects.requireNonNull(super.cqlName(), "cqlName");
lazyInitBitmap |= CQL_NAME_LAZY_INIT_BIT;
}
}
}
return cqlName;
}
private static final long CQL_KEYSPACE_LAZY_INIT_BIT = 0x2L;
private transient String cqlKeyspace;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#cqlKeyspace() cqlKeyspace} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code cqlKeyspace} attribute
*/
@Override
public String cqlKeyspace() {
if ((lazyInitBitmap & CQL_KEYSPACE_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & CQL_KEYSPACE_LAZY_INIT_BIT) == 0) {
this.cqlKeyspace = Objects.requireNonNull(super.cqlKeyspace(), "cqlKeyspace");
lazyInitBitmap |= CQL_KEYSPACE_LAZY_INIT_BIT;
}
}
}
return cqlKeyspace;
}
private static final long CQL_QUALIFIED_NAME_LAZY_INIT_BIT = 0x4L;
private transient String cqlQualifiedName;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#cqlQualifiedName() cqlQualifiedName} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code cqlQualifiedName} attribute
*/
@Override
public String cqlQualifiedName() {
if ((lazyInitBitmap & CQL_QUALIFIED_NAME_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & CQL_QUALIFIED_NAME_LAZY_INIT_BIT) == 0) {
this.cqlQualifiedName = Objects.requireNonNull(super.cqlQualifiedName(), "cqlQualifiedName");
lazyInitBitmap |= CQL_QUALIFIED_NAME_LAZY_INIT_BIT;
}
}
}
return cqlQualifiedName;
}
private static final long COLUMN_MAP_LAZY_INIT_BIT = 0x8L;
private transient Map columnMap;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#columnMap() columnMap} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code columnMap} attribute
*/
@Override
Map columnMap() {
if ((lazyInitBitmap & COLUMN_MAP_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & COLUMN_MAP_LAZY_INIT_BIT) == 0) {
this.columnMap = Objects.requireNonNull(super.columnMap(), "columnMap");
lazyInitBitmap |= COLUMN_MAP_LAZY_INIT_BIT;
}
}
}
return columnMap;
}
private static final long PARTITION_KEY_COLUMNS_LAZY_INIT_BIT = 0x10L;
private transient List partitionKeyColumns;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#partitionKeyColumns() partitionKeyColumns} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code partitionKeyColumns} attribute
*/
@Override
public List partitionKeyColumns() {
if ((lazyInitBitmap & PARTITION_KEY_COLUMNS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & PARTITION_KEY_COLUMNS_LAZY_INIT_BIT) == 0) {
this.partitionKeyColumns = Objects.requireNonNull(super.partitionKeyColumns(), "partitionKeyColumns");
lazyInitBitmap |= PARTITION_KEY_COLUMNS_LAZY_INIT_BIT;
}
}
}
return partitionKeyColumns;
}
private static final long CLUSTERING_KEY_COLUMNS_LAZY_INIT_BIT = 0x20L;
private transient List clusteringKeyColumns;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#clusteringKeyColumns() clusteringKeyColumns} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code clusteringKeyColumns} attribute
*/
@Override
public List clusteringKeyColumns() {
if ((lazyInitBitmap & CLUSTERING_KEY_COLUMNS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & CLUSTERING_KEY_COLUMNS_LAZY_INIT_BIT) == 0) {
this.clusteringKeyColumns = Objects.requireNonNull(super.clusteringKeyColumns(), "clusteringKeyColumns");
lazyInitBitmap |= CLUSTERING_KEY_COLUMNS_LAZY_INIT_BIT;
}
}
}
return clusteringKeyColumns;
}
private static final long PRIMARY_KEY_COLUMNS_LAZY_INIT_BIT = 0x40L;
private transient List primaryKeyColumns;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#primaryKeyColumns() primaryKeyColumns} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code primaryKeyColumns} attribute
*/
@Override
public List primaryKeyColumns() {
if ((lazyInitBitmap & PRIMARY_KEY_COLUMNS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & PRIMARY_KEY_COLUMNS_LAZY_INIT_BIT) == 0) {
this.primaryKeyColumns = Objects.requireNonNull(super.primaryKeyColumns(), "primaryKeyColumns");
lazyInitBitmap |= PRIMARY_KEY_COLUMNS_LAZY_INIT_BIT;
}
}
}
return primaryKeyColumns;
}
private static final long REGULAR_AND_STATIC_COLUMNS_LAZY_INIT_BIT = 0x80L;
private transient List regularAndStaticColumns;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#regularAndStaticColumns() regularAndStaticColumns} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code regularAndStaticColumns} attribute
*/
@Override
public List regularAndStaticColumns() {
if ((lazyInitBitmap & REGULAR_AND_STATIC_COLUMNS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & REGULAR_AND_STATIC_COLUMNS_LAZY_INIT_BIT) == 0) {
this.regularAndStaticColumns = Objects.requireNonNull(super.regularAndStaticColumns(), "regularAndStaticColumns");
lazyInitBitmap |= REGULAR_AND_STATIC_COLUMNS_LAZY_INIT_BIT;
}
}
}
return regularAndStaticColumns;
}
private static final long REQUIRED_INDEX_COLUMNS_LAZY_INIT_BIT = 0x100L;
private transient Set requiredIndexColumns;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#getRequiredIndexColumns() requiredIndexColumns} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code requiredIndexColumns} attribute
*/
@Override
public Set getRequiredIndexColumns() {
if ((lazyInitBitmap & REQUIRED_INDEX_COLUMNS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & REQUIRED_INDEX_COLUMNS_LAZY_INIT_BIT) == 0) {
this.requiredIndexColumns = Objects.requireNonNull(super.getRequiredIndexColumns(), "requiredIndexColumns");
lazyInitBitmap |= REQUIRED_INDEX_COLUMNS_LAZY_INIT_BIT;
}
}
}
return requiredIndexColumns;
}
private static final long OPTIONAL_INDEX_COLUMNS_LAZY_INIT_BIT = 0x200L;
private transient Set optionalIndexColumns;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link MaterializedView#getOptionalIndexColumns() optionalIndexColumns} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* In case of any exception or error thrown by the lazy value initializer,
* the result will not be memoised (i.e. remembered) and on next call computation
* will be attempted again.
* @return A lazily initialized value of the {@code optionalIndexColumns} attribute
*/
@Override
public Set getOptionalIndexColumns() {
if ((lazyInitBitmap & OPTIONAL_INDEX_COLUMNS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & OPTIONAL_INDEX_COLUMNS_LAZY_INIT_BIT) == 0) {
this.optionalIndexColumns = Objects.requireNonNull(super.getOptionalIndexColumns(), "optionalIndexColumns");
lazyInitBitmap |= OPTIONAL_INDEX_COLUMNS_LAZY_INIT_BIT;
}
}
}
return optionalIndexColumns;
}
/**
* Creates an immutable copy of a {@link MaterializedView} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable MaterializedView instance
*/
public static ImmutableMaterializedView copyOf(MaterializedView instance) {
if (instance instanceof ImmutableMaterializedView) {
return (ImmutableMaterializedView) instance;
}
return ImmutableMaterializedView.builder()
.from(instance)
.build();
}
private static final long serialVersionUID = -2999120284516448661L;
private Object readResolve() throws ObjectStreamException {
return new ImmutableMaterializedView(this.name, this.keyspace, this.columns);
}
/**
* Creates a builder for {@link ImmutableMaterializedView ImmutableMaterializedView}.
*
* ImmutableMaterializedView.builder()
* .name(String) // required {@link MaterializedView#name() name}
* .keyspace(String) // required {@link MaterializedView#keyspace() keyspace}
* .addColumns|addAllColumns(io.stargate.db.schema.Column) // {@link MaterializedView#columns() columns} elements
* .build();
*
* @return A new ImmutableMaterializedView builder
*/
public static ImmutableMaterializedView.Builder builder() {
return new ImmutableMaterializedView.Builder();
}
/**
* Builds instances of type {@link ImmutableMaterializedView ImmutableMaterializedView}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "MaterializedView", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long INIT_BIT_KEYSPACE = 0x2L;
private long initBits = 0x3L;
private @Nullable String name;
private @Nullable String keyspace;
private List columns = new ArrayList();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code io.stargate.db.schema.SchemaEntity} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SchemaEntity instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.stargate.db.schema.QualifiedSchemaEntity} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(QualifiedSchemaEntity instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.stargate.db.schema.AbstractTable} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AbstractTable instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code io.stargate.db.schema.MaterializedView} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(MaterializedView instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof SchemaEntity) {
SchemaEntity instance = (SchemaEntity) object;
name(instance.name());
}
if (object instanceof QualifiedSchemaEntity) {
QualifiedSchemaEntity instance = (QualifiedSchemaEntity) object;
keyspace(instance.keyspace());
}
if (object instanceof AbstractTable) {
AbstractTable instance = (AbstractTable) object;
addAllColumns(instance.columns());
}
}
/**
* Initializes the value for the {@link MaterializedView#name() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link MaterializedView#keyspace() keyspace} attribute.
* @param keyspace The value for keyspace
* @return {@code this} builder for use in a chained invocation
*/
public final Builder keyspace(String keyspace) {
this.keyspace = Objects.requireNonNull(keyspace, "keyspace");
initBits &= ~INIT_BIT_KEYSPACE;
return this;
}
/**
* Adds one element to {@link MaterializedView#columns() columns} list.
* @param element A columns element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addColumns(Column element) {
this.columns.add(Objects.requireNonNull(element, "columns element"));
return this;
}
/**
* Adds elements to {@link MaterializedView#columns() columns} list.
* @param elements An array of columns elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addColumns(Column... elements) {
for (Column element : elements) {
this.columns.add(Objects.requireNonNull(element, "columns element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link MaterializedView#columns() columns} list.
* @param elements An iterable of columns elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder columns(Iterable extends Column> elements) {
this.columns.clear();
return addAllColumns(elements);
}
/**
* Adds elements to {@link MaterializedView#columns() columns} list.
* @param elements An iterable of columns elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllColumns(Iterable extends Column> elements) {
for (Column element : elements) {
this.columns.add(Objects.requireNonNull(element, "columns element"));
}
return this;
}
/**
* Builds a new {@link ImmutableMaterializedView ImmutableMaterializedView}.
* @return An immutable instance of MaterializedView
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableMaterializedView build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableMaterializedView(name, keyspace, createUnmodifiableList(true, columns));
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_KEYSPACE) != 0) attributes.add("keyspace");
return "Cannot build MaterializedView, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}