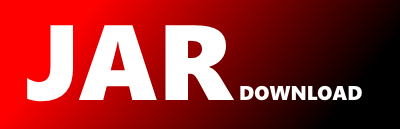
io.stargate.db.query.Query Maven / Gradle / Ivy
package io.stargate.db.query;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import org.apache.cassandra.stargate.exceptions.InvalidRequestException;
import org.apache.cassandra.stargate.utils.MD5Digest;
/**
* A CQL query.
*
* Implementations of this class make no strong guarantees on the validity of the query they
* represent; executing them may raise an {@link InvalidRequestException}.
*/
public interface Query {
TypedValue.Codec valueCodec();
/** The (potentially empty) list of bind markers that remains to be bound in this query. */
List bindMarkers();
/** If this is a prepared query, it's prepared ID. */
Optional preparedId();
/** Creates a prepared copy of this query, using the provided prepared ID. */
Query withPreparedId(MD5Digest preparedId);
/**
* The query string that must be used to prepare this query.
*
* Please note that the query string returned by this method may or may not be equal to the one
* of {@link #toString()} (in particular, the query is allowed to prepare some of values that are
* not part of {@link #bindMarkers()}, to optimize prepared statement caching for instance).
*/
String queryStringForPreparation();
B bindValues(List values);
default B bind(Object... values) {
return bind(Arrays.asList(values));
}
default B bind(List