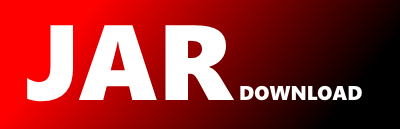
io.stargate.graphql.schema.cqlfirst.dml.fetchers.DmlFetcher Maven / Gradle / Ivy
package io.stargate.graphql.schema.cqlfirst.dml.fetchers;
import static io.stargate.graphql.schema.SchemaConstants.ASYNC_DIRECTIVE;
import com.datastax.oss.driver.shaded.guava.common.collect.ImmutableList;
import com.datastax.oss.driver.shaded.guava.common.collect.ImmutableMap;
import graphql.language.OperationDefinition;
import graphql.schema.DataFetchingEnvironment;
import io.stargate.db.ImmutableParameters;
import io.stargate.db.Parameters;
import io.stargate.db.datastore.ResultSet;
import io.stargate.db.datastore.Row;
import io.stargate.db.query.BoundQuery;
import io.stargate.db.query.Predicate;
import io.stargate.db.query.builder.BuiltCondition;
import io.stargate.db.schema.Column;
import io.stargate.db.schema.Column.ColumnType;
import io.stargate.db.schema.Table;
import io.stargate.graphql.schema.CassandraFetcher;
import io.stargate.graphql.schema.cqlfirst.dml.NameMapping;
import io.stargate.graphql.web.StargateGraphqlContext;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.Base64;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.function.UnaryOperator;
import java.util.stream.Collectors;
import org.apache.cassandra.stargate.db.ConsistencyLevel;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public abstract class DmlFetcher extends CassandraFetcher {
private static final Logger log = LoggerFactory.getLogger(DmlFetcher.class);
protected final Table table;
protected final NameMapping nameMapping;
protected final DbColumnGetter dbColumnGetter;
protected DmlFetcher(Table table, NameMapping nameMapping) {
this.table = table;
this.nameMapping = nameMapping;
this.dbColumnGetter = new DbColumnGetter(nameMapping);
}
protected Parameters buildParameters(DataFetchingEnvironment environment) {
Map options = environment.getArgument("options");
if (options == null) {
return DEFAULT_PARAMETERS;
}
ImmutableParameters.Builder builder = DEFAULT_PARAMETERS.toBuilder();
Object consistency = options.get("consistency");
if (consistency != null) {
builder.consistencyLevel(ConsistencyLevel.valueOf((String) consistency));
}
Object serialConsistency = options.get("serialConsistency");
if (serialConsistency != null) {
builder.serialConsistencyLevel(ConsistencyLevel.valueOf((String) serialConsistency));
}
Object pageSize = options.get("pageSize");
if (pageSize != null) {
builder.pageSize((Integer) pageSize);
}
Object pageState = options.get("pageState");
if (pageState != null) {
builder.pagingState(ByteBuffer.wrap(Base64.getDecoder().decode((String) pageState)));
}
return builder.build();
}
protected List buildConditions(
Table table, Map> columnList) {
if (columnList == null) {
return ImmutableList.of();
}
List where = new ArrayList<>();
for (Map.Entry> clauseEntry : columnList.entrySet()) {
Column column = dbColumnGetter.getColumn(table, clauseEntry.getKey());
for (Map.Entry condition : clauseEntry.getValue().entrySet()) {
FilterOperator operator = FilterOperator.fromFieldName(condition.getKey());
where.add(operator.buildCondition(column, condition.getValue(), nameMapping));
}
}
return where;
}
protected List buildClause(Table table, DataFetchingEnvironment environment) {
if (environment.containsArgument("filter")) {
Map> columnList = environment.getArgument("filter");
return buildConditions(table, columnList);
} else {
Map value = environment.getArgument("value");
List relations = new ArrayList<>();
if (value == null) return ImmutableList.of();
for (Map.Entry entry : value.entrySet()) {
Column column = dbColumnGetter.getColumn(table, entry.getKey());
Object whereValue = toDBValue(column.type(), entry.getValue());
relations.add(BuiltCondition.of(column.name(), Predicate.EQ, whereValue));
}
return relations;
}
}
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy