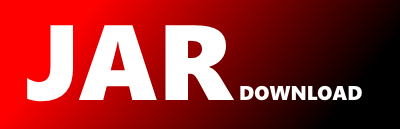
io.stargate.proto.StargateGrpc Maven / Gradle / Ivy
package io.stargate.proto;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* The gPRC service to interact with a Stargate coordinator.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.56.1)",
comments = "Source: stargate.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class StargateGrpc {
private StargateGrpc() {}
public static final String SERVICE_NAME = "stargate.Stargate";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getExecuteQueryMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteQuery",
requestType = io.stargate.proto.QueryOuterClass.Query.class,
responseType = io.stargate.proto.QueryOuterClass.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExecuteQueryMethod() {
io.grpc.MethodDescriptor getExecuteQueryMethod;
if ((getExecuteQueryMethod = StargateGrpc.getExecuteQueryMethod) == null) {
synchronized (StargateGrpc.class) {
if ((getExecuteQueryMethod = StargateGrpc.getExecuteQueryMethod) == null) {
StargateGrpc.getExecuteQueryMethod = getExecuteQueryMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteQuery"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.stargate.proto.QueryOuterClass.Query.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.stargate.proto.QueryOuterClass.Response.getDefaultInstance()))
.setSchemaDescriptor(new StargateMethodDescriptorSupplier("ExecuteQuery"))
.build();
}
}
}
return getExecuteQueryMethod;
}
private static volatile io.grpc.MethodDescriptor getExecuteQueryStreamMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteQueryStream",
requestType = io.stargate.proto.QueryOuterClass.Query.class,
responseType = io.stargate.proto.QueryOuterClass.StreamingResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
public static io.grpc.MethodDescriptor getExecuteQueryStreamMethod() {
io.grpc.MethodDescriptor getExecuteQueryStreamMethod;
if ((getExecuteQueryStreamMethod = StargateGrpc.getExecuteQueryStreamMethod) == null) {
synchronized (StargateGrpc.class) {
if ((getExecuteQueryStreamMethod = StargateGrpc.getExecuteQueryStreamMethod) == null) {
StargateGrpc.getExecuteQueryStreamMethod = getExecuteQueryStreamMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteQueryStream"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.stargate.proto.QueryOuterClass.Query.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.stargate.proto.QueryOuterClass.StreamingResponse.getDefaultInstance()))
.setSchemaDescriptor(new StargateMethodDescriptorSupplier("ExecuteQueryStream"))
.build();
}
}
}
return getExecuteQueryStreamMethod;
}
private static volatile io.grpc.MethodDescriptor getExecuteBatchMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteBatch",
requestType = io.stargate.proto.QueryOuterClass.Batch.class,
responseType = io.stargate.proto.QueryOuterClass.Response.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExecuteBatchMethod() {
io.grpc.MethodDescriptor getExecuteBatchMethod;
if ((getExecuteBatchMethod = StargateGrpc.getExecuteBatchMethod) == null) {
synchronized (StargateGrpc.class) {
if ((getExecuteBatchMethod = StargateGrpc.getExecuteBatchMethod) == null) {
StargateGrpc.getExecuteBatchMethod = getExecuteBatchMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteBatch"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.stargate.proto.QueryOuterClass.Batch.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.stargate.proto.QueryOuterClass.Response.getDefaultInstance()))
.setSchemaDescriptor(new StargateMethodDescriptorSupplier("ExecuteBatch"))
.build();
}
}
}
return getExecuteBatchMethod;
}
private static volatile io.grpc.MethodDescriptor getExecuteBatchStreamMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteBatchStream",
requestType = io.stargate.proto.QueryOuterClass.Batch.class,
responseType = io.stargate.proto.QueryOuterClass.StreamingResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
public static io.grpc.MethodDescriptor getExecuteBatchStreamMethod() {
io.grpc.MethodDescriptor getExecuteBatchStreamMethod;
if ((getExecuteBatchStreamMethod = StargateGrpc.getExecuteBatchStreamMethod) == null) {
synchronized (StargateGrpc.class) {
if ((getExecuteBatchStreamMethod = StargateGrpc.getExecuteBatchStreamMethod) == null) {
StargateGrpc.getExecuteBatchStreamMethod = getExecuteBatchStreamMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteBatchStream"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.stargate.proto.QueryOuterClass.Batch.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.stargate.proto.QueryOuterClass.StreamingResponse.getDefaultInstance()))
.setSchemaDescriptor(new StargateMethodDescriptorSupplier("ExecuteBatchStream"))
.build();
}
}
}
return getExecuteBatchStreamMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static StargateStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public StargateStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateStub(channel, callOptions);
}
};
return StargateStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static StargateBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public StargateBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateBlockingStub(channel, callOptions);
}
};
return StargateBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static StargateFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public StargateFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateFutureStub(channel, callOptions);
}
};
return StargateFutureStub.newStub(factory, channel);
}
/**
*
* The gPRC service to interact with a Stargate coordinator.
*
*/
public interface AsyncService {
/**
*
* Executes a single CQL query.
*
*/
default void executeQuery(io.stargate.proto.QueryOuterClass.Query request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteQueryMethod(), responseObserver);
}
/**
*
* Executes a bi-directional streaming for CQL queries.
*
*/
default io.grpc.stub.StreamObserver executeQueryStream(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall(getExecuteQueryStreamMethod(), responseObserver);
}
/**
*
* Executes a batch of CQL queries.
*
*/
default void executeBatch(io.stargate.proto.QueryOuterClass.Batch request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteBatchMethod(), responseObserver);
}
/**
*
* Executes a bi-directional streaming for batches of CQL queries.
*
*/
default io.grpc.stub.StreamObserver executeBatchStream(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall(getExecuteBatchStreamMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service Stargate.
*
* The gPRC service to interact with a Stargate coordinator.
*
*/
public static abstract class StargateImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return StargateGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service Stargate.
*
* The gPRC service to interact with a Stargate coordinator.
*
*/
public static final class StargateStub
extends io.grpc.stub.AbstractAsyncStub {
private StargateStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected StargateStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateStub(channel, callOptions);
}
/**
*
* Executes a single CQL query.
*
*/
public void executeQuery(io.stargate.proto.QueryOuterClass.Query request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteQueryMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Executes a bi-directional streaming for CQL queries.
*
*/
public io.grpc.stub.StreamObserver executeQueryStream(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ClientCalls.asyncBidiStreamingCall(
getChannel().newCall(getExecuteQueryStreamMethod(), getCallOptions()), responseObserver);
}
/**
*
* Executes a batch of CQL queries.
*
*/
public void executeBatch(io.stargate.proto.QueryOuterClass.Batch request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteBatchMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Executes a bi-directional streaming for batches of CQL queries.
*
*/
public io.grpc.stub.StreamObserver executeBatchStream(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ClientCalls.asyncBidiStreamingCall(
getChannel().newCall(getExecuteBatchStreamMethod(), getCallOptions()), responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service Stargate.
*
* The gPRC service to interact with a Stargate coordinator.
*
*/
public static final class StargateBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private StargateBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected StargateBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateBlockingStub(channel, callOptions);
}
/**
*
* Executes a single CQL query.
*
*/
public io.stargate.proto.QueryOuterClass.Response executeQuery(io.stargate.proto.QueryOuterClass.Query request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteQueryMethod(), getCallOptions(), request);
}
/**
*
* Executes a batch of CQL queries.
*
*/
public io.stargate.proto.QueryOuterClass.Response executeBatch(io.stargate.proto.QueryOuterClass.Batch request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteBatchMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service Stargate.
*
* The gPRC service to interact with a Stargate coordinator.
*
*/
public static final class StargateFutureStub
extends io.grpc.stub.AbstractFutureStub {
private StargateFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected StargateFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateFutureStub(channel, callOptions);
}
/**
*
* Executes a single CQL query.
*
*/
public com.google.common.util.concurrent.ListenableFuture executeQuery(
io.stargate.proto.QueryOuterClass.Query request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExecuteQueryMethod(), getCallOptions()), request);
}
/**
*
* Executes a batch of CQL queries.
*
*/
public com.google.common.util.concurrent.ListenableFuture executeBatch(
io.stargate.proto.QueryOuterClass.Batch request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExecuteBatchMethod(), getCallOptions()), request);
}
}
private static final int METHODID_EXECUTE_QUERY = 0;
private static final int METHODID_EXECUTE_BATCH = 1;
private static final int METHODID_EXECUTE_QUERY_STREAM = 2;
private static final int METHODID_EXECUTE_BATCH_STREAM = 3;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_EXECUTE_QUERY:
serviceImpl.executeQuery((io.stargate.proto.QueryOuterClass.Query) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_BATCH:
serviceImpl.executeBatch((io.stargate.proto.QueryOuterClass.Batch) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_EXECUTE_QUERY_STREAM:
return (io.grpc.stub.StreamObserver) serviceImpl.executeQueryStream(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_EXECUTE_BATCH_STREAM:
return (io.grpc.stub.StreamObserver) serviceImpl.executeBatchStream(
(io.grpc.stub.StreamObserver) responseObserver);
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getExecuteQueryMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
io.stargate.proto.QueryOuterClass.Query,
io.stargate.proto.QueryOuterClass.Response>(
service, METHODID_EXECUTE_QUERY)))
.addMethod(
getExecuteQueryStreamMethod(),
io.grpc.stub.ServerCalls.asyncBidiStreamingCall(
new MethodHandlers<
io.stargate.proto.QueryOuterClass.Query,
io.stargate.proto.QueryOuterClass.StreamingResponse>(
service, METHODID_EXECUTE_QUERY_STREAM)))
.addMethod(
getExecuteBatchMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
io.stargate.proto.QueryOuterClass.Batch,
io.stargate.proto.QueryOuterClass.Response>(
service, METHODID_EXECUTE_BATCH)))
.addMethod(
getExecuteBatchStreamMethod(),
io.grpc.stub.ServerCalls.asyncBidiStreamingCall(
new MethodHandlers<
io.stargate.proto.QueryOuterClass.Batch,
io.stargate.proto.QueryOuterClass.StreamingResponse>(
service, METHODID_EXECUTE_BATCH_STREAM)))
.build();
}
private static abstract class StargateBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
StargateBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return io.stargate.proto.StargateOuterClass.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Stargate");
}
}
private static final class StargateFileDescriptorSupplier
extends StargateBaseDescriptorSupplier {
StargateFileDescriptorSupplier() {}
}
private static final class StargateMethodDescriptorSupplier
extends StargateBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
StargateMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (StargateGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new StargateFileDescriptorSupplier())
.addMethod(getExecuteQueryMethod())
.addMethod(getExecuteQueryStreamMethod())
.addMethod(getExecuteBatchMethod())
.addMethod(getExecuteBatchStreamMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy