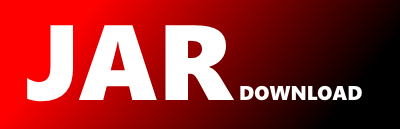
com.example.graphql.client.betterbotz.collections.GetCollectionsNestedQuery Maven / Gradle / Ivy
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package com.example.graphql.client.betterbotz.collections;
import com.apollographql.apollo.api.Input;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Query;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.Mutator;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import com.example.graphql.client.betterbotz.type.CollectionsNestedInput;
import com.example.graphql.client.betterbotz.type.CustomType;
import java.io.IOException;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.ArrayList;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class GetCollectionsNestedQuery implements Query, GetCollectionsNestedQuery.Variables> {
public static final String OPERATION_ID = "8fe0480180bb5f609cb04bf0ca8781c33d96bddb98b7193b9537e365d0a8fc3b";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"query GetCollectionsNested($value: CollectionsNestedInput) {\n"
+ " CollectionsNested(value: $value) {\n"
+ " __typename\n"
+ " pageState\n"
+ " values {\n"
+ " __typename\n"
+ " id\n"
+ " listValue1 {\n"
+ " __typename\n"
+ " key\n"
+ " value\n"
+ " }\n"
+ " setValue1\n"
+ " mapValue1 {\n"
+ " __typename\n"
+ " key\n"
+ " value {\n"
+ " __typename\n"
+ " key\n"
+ " value\n"
+ " }\n"
+ " }\n"
+ " }\n"
+ " }\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "GetCollectionsNested";
}
};
private final GetCollectionsNestedQuery.Variables variables;
public GetCollectionsNestedQuery(@NotNull Input value) {
Utils.checkNotNull(value, "value == null");
variables = new GetCollectionsNestedQuery.Variables(value);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public Optional wrapData(GetCollectionsNestedQuery.Data data) {
return Optional.ofNullable(data);
}
@Override
public GetCollectionsNestedQuery.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response> parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response> parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response> parse(@NotNull final BufferedSource source)
throws IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response> parse(@NotNull final ByteString byteString)
throws IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private Input value = Input.absent();
Builder() {
}
public Builder value(@Nullable CollectionsNestedInput value) {
this.value = Input.fromNullable(value);
return this;
}
public Builder valueInput(@NotNull Input value) {
this.value = Utils.checkNotNull(value, "value == null");
return this;
}
public GetCollectionsNestedQuery build() {
return new GetCollectionsNestedQuery(value);
}
}
public static final class Variables extends Operation.Variables {
private final Input value;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(Input value) {
this.value = value;
if (value.defined) {
this.valueMap.put("value", value.value);
}
}
public Input value() {
return value;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
if (value.defined) {
writer.writeObject("value", value.value != null ? value.value.marshaller() : null);
}
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("CollectionsNested", "CollectionsNested", new UnmodifiableMapBuilder(1)
.put("value", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "value")
.build())
.build(), true, Collections.emptyList())
};
final Optional CollectionsNested;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable CollectionsNested CollectionsNested) {
this.CollectionsNested = Optional.ofNullable(CollectionsNested);
}
/**
* Query for the table 'CollectionsNested'.
* Note that 'id' is the field that corresponds to the table primary key.
*/
public Optional getCollectionsNested() {
return this.CollectionsNested;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], CollectionsNested.isPresent() ? CollectionsNested.get().marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "CollectionsNested=" + CollectionsNested
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return this.CollectionsNested.equals(that.CollectionsNested);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= CollectionsNested.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public Builder toBuilder() {
Builder builder = new Builder();
builder.CollectionsNested = CollectionsNested.isPresent() ? CollectionsNested.get() : null;
return builder;
}
public static Builder builder() {
return new Builder();
}
public static final class Mapper implements ResponseFieldMapper {
final CollectionsNested.Mapper collectionsNestedFieldMapper = new CollectionsNested.Mapper();
@Override
public Data map(ResponseReader reader) {
final CollectionsNested CollectionsNested = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public CollectionsNested read(ResponseReader reader) {
return collectionsNestedFieldMapper.map(reader);
}
});
return new Data(CollectionsNested);
}
}
public static final class Builder {
private @Nullable CollectionsNested CollectionsNested;
Builder() {
}
public Builder CollectionsNested(@Nullable CollectionsNested CollectionsNested) {
this.CollectionsNested = CollectionsNested;
return this;
}
public Builder CollectionsNested(@NotNull Mutator mutator) {
Utils.checkNotNull(mutator, "mutator == null");
CollectionsNested.Builder builder = this.CollectionsNested != null ? this.CollectionsNested.toBuilder() : CollectionsNested.builder();
mutator.accept(builder);
this.CollectionsNested = builder.build();
return this;
}
public Data build() {
return new Data(CollectionsNested);
}
}
}
public static class CollectionsNested {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forString("pageState", "pageState", null, true, Collections.emptyList()),
ResponseField.forList("values", "values", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final Optional pageState;
final Optional> values;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public CollectionsNested(@NotNull String __typename, @Nullable String pageState,
@Nullable List values) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.pageState = Optional.ofNullable(pageState);
this.values = Optional.ofNullable(values);
}
public @NotNull String get__typename() {
return this.__typename;
}
public Optional getPageState() {
return this.pageState;
}
public Optional> getValues() {
return this.values;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeString($responseFields[1], pageState.isPresent() ? pageState.get() : null);
writer.writeList($responseFields[2], values.isPresent() ? values.get() : null, new ResponseWriter.ListWriter() {
@Override
public void write(List items, ResponseWriter.ListItemWriter listItemWriter) {
for (Object item : items) {
listItemWriter.writeObject(((Value) item).marshaller());
}
}
});
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "CollectionsNested{"
+ "__typename=" + __typename + ", "
+ "pageState=" + pageState + ", "
+ "values=" + values
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CollectionsNested) {
CollectionsNested that = (CollectionsNested) o;
return this.__typename.equals(that.__typename)
&& this.pageState.equals(that.pageState)
&& this.values.equals(that.values);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= pageState.hashCode();
h *= 1000003;
h ^= values.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public Builder toBuilder() {
Builder builder = new Builder();
builder.__typename = __typename;
builder.pageState = pageState.isPresent() ? pageState.get() : null;
builder.values = values.isPresent() ? values.get() : null;
return builder;
}
public static Builder builder() {
return new Builder();
}
public static final class Mapper implements ResponseFieldMapper {
final Value.Mapper valueFieldMapper = new Value.Mapper();
@Override
public CollectionsNested map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final String pageState = reader.readString($responseFields[1]);
final List values = reader.readList($responseFields[2], new ResponseReader.ListReader() {
@Override
public Value read(ResponseReader.ListItemReader listItemReader) {
return listItemReader.readObject(new ResponseReader.ObjectReader() {
@Override
public Value read(ResponseReader reader) {
return valueFieldMapper.map(reader);
}
});
}
});
return new CollectionsNested(__typename, pageState, values);
}
}
public static final class Builder {
private @NotNull String __typename;
private @Nullable String pageState;
private @Nullable List values;
Builder() {
}
public Builder __typename(@NotNull String __typename) {
this.__typename = __typename;
return this;
}
public Builder pageState(@Nullable String pageState) {
this.pageState = pageState;
return this;
}
public Builder values(@Nullable List values) {
this.values = values;
return this;
}
public Builder values(@NotNull Mutator> mutator) {
Utils.checkNotNull(mutator, "mutator == null");
List builders = new ArrayList<>();
if (this.values != null) {
for (Value item : this.values) {
builders.add(item != null ? item.toBuilder() : null);
}
}
mutator.accept(builders);
List values = new ArrayList<>();
for (Value.Builder item : builders) {
values.add(item != null ? item.build() : null);
}
this.values = values;
return this;
}
public CollectionsNested build() {
Utils.checkNotNull(__typename, "__typename == null");
return new CollectionsNested(__typename, pageState, values);
}
}
}
/**
* The type used to represent results of a query for the table 'CollectionsNested'.
*/
public static class Value {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, true, CustomType.UUID, Collections.emptyList()),
ResponseField.forList("listValue1", "listValue1", null, true, Collections.emptyList()),
ResponseField.forList("setValue1", "setValue1", null, true, Collections.emptyList()),
ResponseField.forList("mapValue1", "mapValue1", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy