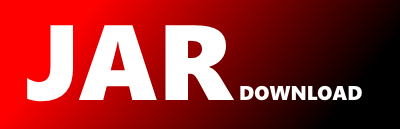
com.example.graphql.client.betterbotz.udts.InsertUdtsMutation Maven / Gradle / Ivy
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package com.example.graphql.client.betterbotz.udts;
import com.apollographql.apollo.api.Input;
import com.apollographql.apollo.api.Mutation;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.Mutator;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import com.example.graphql.client.betterbotz.type.MutationOptions;
import com.example.graphql.client.betterbotz.type.UdtsInput;
import java.io.IOException;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Optional;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class InsertUdtsMutation implements Mutation, InsertUdtsMutation.Variables> {
public static final String OPERATION_ID = "7f96f1bff5b63addedf9ab31fdc1f66836675ccecdcbdaf39f48ca15fbe87cec";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"mutation InsertUdts($value: UdtsInput!, $ifNotExists: Boolean, $options: MutationOptions) {\n"
+ " insertUdts(value: $value, ifNotExists: $ifNotExists, options: $options) {\n"
+ " __typename\n"
+ " applied\n"
+ " }\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "InsertUdts";
}
};
private final InsertUdtsMutation.Variables variables;
public InsertUdtsMutation(@NotNull UdtsInput value, @NotNull Input ifNotExists,
@NotNull Input options) {
Utils.checkNotNull(value, "value == null");
Utils.checkNotNull(ifNotExists, "ifNotExists == null");
Utils.checkNotNull(options, "options == null");
variables = new InsertUdtsMutation.Variables(value, ifNotExists, options);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public Optional wrapData(InsertUdtsMutation.Data data) {
return Optional.ofNullable(data);
}
@Override
public InsertUdtsMutation.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response> parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response> parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response> parse(@NotNull final BufferedSource source)
throws IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response> parse(@NotNull final ByteString byteString)
throws IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private @NotNull UdtsInput value;
private Input ifNotExists = Input.absent();
private Input options = Input.absent();
Builder() {
}
public Builder value(@NotNull UdtsInput value) {
this.value = value;
return this;
}
public Builder ifNotExists(@Nullable Boolean ifNotExists) {
this.ifNotExists = Input.fromNullable(ifNotExists);
return this;
}
public Builder options(@Nullable MutationOptions options) {
this.options = Input.fromNullable(options);
return this;
}
public Builder ifNotExistsInput(@NotNull Input ifNotExists) {
this.ifNotExists = Utils.checkNotNull(ifNotExists, "ifNotExists == null");
return this;
}
public Builder optionsInput(@NotNull Input options) {
this.options = Utils.checkNotNull(options, "options == null");
return this;
}
public InsertUdtsMutation build() {
Utils.checkNotNull(value, "value == null");
return new InsertUdtsMutation(value, ifNotExists, options);
}
}
public static final class Variables extends Operation.Variables {
private final @NotNull UdtsInput value;
private final Input ifNotExists;
private final Input options;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(@NotNull UdtsInput value, Input ifNotExists,
Input options) {
this.value = value;
this.ifNotExists = ifNotExists;
this.options = options;
this.valueMap.put("value", value);
if (ifNotExists.defined) {
this.valueMap.put("ifNotExists", ifNotExists.value);
}
if (options.defined) {
this.valueMap.put("options", options.value);
}
}
public @NotNull UdtsInput value() {
return value;
}
public Input ifNotExists() {
return ifNotExists;
}
public Input options() {
return options;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
writer.writeObject("value", value.marshaller());
if (ifNotExists.defined) {
writer.writeBoolean("ifNotExists", ifNotExists.value);
}
if (options.defined) {
writer.writeObject("options", options.value != null ? options.value.marshaller() : null);
}
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("insertUdts", "insertUdts", new UnmodifiableMapBuilder(3)
.put("value", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "value")
.build())
.put("ifNotExists", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "ifNotExists")
.build())
.put("options", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "options")
.build())
.build(), true, Collections.emptyList())
};
final Optional insertUdts;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable InsertUdts insertUdts) {
this.insertUdts = Optional.ofNullable(insertUdts);
}
/**
* Insert mutation for the table 'Udts'.
* Note that 'a' is the field that corresponds to the table primary key.
*/
public Optional getInsertUdts() {
return this.insertUdts;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], insertUdts.isPresent() ? insertUdts.get().marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "insertUdts=" + insertUdts
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return this.insertUdts.equals(that.insertUdts);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= insertUdts.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public Builder toBuilder() {
Builder builder = new Builder();
builder.insertUdts = insertUdts.isPresent() ? insertUdts.get() : null;
return builder;
}
public static Builder builder() {
return new Builder();
}
public static final class Mapper implements ResponseFieldMapper {
final InsertUdts.Mapper insertUdtsFieldMapper = new InsertUdts.Mapper();
@Override
public Data map(ResponseReader reader) {
final InsertUdts insertUdts = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public InsertUdts read(ResponseReader reader) {
return insertUdtsFieldMapper.map(reader);
}
});
return new Data(insertUdts);
}
}
public static final class Builder {
private @Nullable InsertUdts insertUdts;
Builder() {
}
public Builder insertUdts(@Nullable InsertUdts insertUdts) {
this.insertUdts = insertUdts;
return this;
}
public Builder insertUdts(@NotNull Mutator mutator) {
Utils.checkNotNull(mutator, "mutator == null");
InsertUdts.Builder builder = this.insertUdts != null ? this.insertUdts.toBuilder() : InsertUdts.builder();
mutator.accept(builder);
this.insertUdts = builder.build();
return this;
}
public Data build() {
return new Data(insertUdts);
}
}
}
/**
* The type used to represent results of a mutation for the table 'Udts'.
*/
public static class InsertUdts {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forBoolean("applied", "applied", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final Optional applied;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public InsertUdts(@NotNull String __typename, @Nullable Boolean applied) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.applied = Optional.ofNullable(applied);
}
public @NotNull String get__typename() {
return this.__typename;
}
public Optional getApplied() {
return this.applied;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeBoolean($responseFields[1], applied.isPresent() ? applied.get() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "InsertUdts{"
+ "__typename=" + __typename + ", "
+ "applied=" + applied
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof InsertUdts) {
InsertUdts that = (InsertUdts) o;
return this.__typename.equals(that.__typename)
&& this.applied.equals(that.applied);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= applied.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public Builder toBuilder() {
Builder builder = new Builder();
builder.__typename = __typename;
builder.applied = applied.isPresent() ? applied.get() : null;
return builder;
}
public static Builder builder() {
return new Builder();
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public InsertUdts map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Boolean applied = reader.readBoolean($responseFields[1]);
return new InsertUdts(__typename, applied);
}
}
public static final class Builder {
private @NotNull String __typename;
private @Nullable Boolean applied;
Builder() {
}
public Builder __typename(@NotNull String __typename) {
this.__typename = __typename;
return this;
}
public Builder applied(@Nullable Boolean applied) {
this.applied = applied;
return this;
}
public InsertUdts build() {
Utils.checkNotNull(__typename, "__typename == null");
return new InsertUdts(__typename, applied);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy