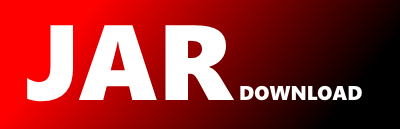
com.example.graphql.client.betterbotz.orders.DeleteOrdersMutation Maven / Gradle / Ivy
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package com.example.graphql.client.betterbotz.orders;
import com.apollographql.apollo.api.Input;
import com.apollographql.apollo.api.Mutation;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.InputFieldMarshaller;
import com.apollographql.apollo.api.internal.InputFieldWriter;
import com.apollographql.apollo.api.internal.Mutator;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.UnmodifiableMapBuilder;
import com.apollographql.apollo.api.internal.Utils;
import com.example.graphql.client.betterbotz.type.CustomType;
import com.example.graphql.client.betterbotz.type.MutationOptions;
import com.example.graphql.client.betterbotz.type.OrdersFilterInput;
import com.example.graphql.client.betterbotz.type.OrdersInput;
import java.io.IOException;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Optional;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class DeleteOrdersMutation implements Mutation, DeleteOrdersMutation.Variables> {
public static final String OPERATION_ID = "614d9233c8360b84d90cc65d46514adf535aedaa9057d74cefa9d4f1b727d559";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"mutation DeleteOrders($value: OrdersInput!, $ifExists: Boolean, $ifCondition: OrdersFilterInput, $options: MutationOptions) {\n"
+ " deleteOrders(value: $value, ifExists: $ifExists, ifCondition: $ifCondition, options: $options) {\n"
+ " __typename\n"
+ " applied\n"
+ " value {\n"
+ " __typename\n"
+ " id\n"
+ " prodId\n"
+ " prodName\n"
+ " customerName\n"
+ " address\n"
+ " description\n"
+ " price\n"
+ " sellPrice\n"
+ " }\n"
+ " }\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "DeleteOrders";
}
};
private final DeleteOrdersMutation.Variables variables;
public DeleteOrdersMutation(@NotNull OrdersInput value, @NotNull Input ifExists,
@NotNull Input ifCondition, @NotNull Input options) {
Utils.checkNotNull(value, "value == null");
Utils.checkNotNull(ifExists, "ifExists == null");
Utils.checkNotNull(ifCondition, "ifCondition == null");
Utils.checkNotNull(options, "options == null");
variables = new DeleteOrdersMutation.Variables(value, ifExists, ifCondition, options);
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public Optional wrapData(DeleteOrdersMutation.Data data) {
return Optional.ofNullable(data);
}
@Override
public DeleteOrdersMutation.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response> parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response> parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response> parse(@NotNull final BufferedSource source)
throws IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response> parse(@NotNull final ByteString byteString)
throws IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
private @NotNull OrdersInput value;
private Input ifExists = Input.absent();
private Input ifCondition = Input.absent();
private Input options = Input.absent();
Builder() {
}
public Builder value(@NotNull OrdersInput value) {
this.value = value;
return this;
}
public Builder ifExists(@Nullable Boolean ifExists) {
this.ifExists = Input.fromNullable(ifExists);
return this;
}
public Builder ifCondition(@Nullable OrdersFilterInput ifCondition) {
this.ifCondition = Input.fromNullable(ifCondition);
return this;
}
public Builder options(@Nullable MutationOptions options) {
this.options = Input.fromNullable(options);
return this;
}
public Builder ifExistsInput(@NotNull Input ifExists) {
this.ifExists = Utils.checkNotNull(ifExists, "ifExists == null");
return this;
}
public Builder ifConditionInput(@NotNull Input ifCondition) {
this.ifCondition = Utils.checkNotNull(ifCondition, "ifCondition == null");
return this;
}
public Builder optionsInput(@NotNull Input options) {
this.options = Utils.checkNotNull(options, "options == null");
return this;
}
public DeleteOrdersMutation build() {
Utils.checkNotNull(value, "value == null");
return new DeleteOrdersMutation(value, ifExists, ifCondition, options);
}
}
public static final class Variables extends Operation.Variables {
private final @NotNull OrdersInput value;
private final Input ifExists;
private final Input ifCondition;
private final Input options;
private final transient Map valueMap = new LinkedHashMap<>();
Variables(@NotNull OrdersInput value, Input ifExists,
Input ifCondition, Input options) {
this.value = value;
this.ifExists = ifExists;
this.ifCondition = ifCondition;
this.options = options;
this.valueMap.put("value", value);
if (ifExists.defined) {
this.valueMap.put("ifExists", ifExists.value);
}
if (ifCondition.defined) {
this.valueMap.put("ifCondition", ifCondition.value);
}
if (options.defined) {
this.valueMap.put("options", options.value);
}
}
public @NotNull OrdersInput value() {
return value;
}
public Input ifExists() {
return ifExists;
}
public Input ifCondition() {
return ifCondition;
}
public Input options() {
return options;
}
@Override
public Map valueMap() {
return Collections.unmodifiableMap(valueMap);
}
@Override
public InputFieldMarshaller marshaller() {
return new InputFieldMarshaller() {
@Override
public void marshal(InputFieldWriter writer) throws IOException {
writer.writeObject("value", value.marshaller());
if (ifExists.defined) {
writer.writeBoolean("ifExists", ifExists.value);
}
if (ifCondition.defined) {
writer.writeObject("ifCondition", ifCondition.value != null ? ifCondition.value.marshaller() : null);
}
if (options.defined) {
writer.writeObject("options", options.value != null ? options.value.marshaller() : null);
}
}
};
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("deleteOrders", "deleteOrders", new UnmodifiableMapBuilder(4)
.put("value", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "value")
.build())
.put("ifExists", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "ifExists")
.build())
.put("ifCondition", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "ifCondition")
.build())
.put("options", new UnmodifiableMapBuilder(2)
.put("kind", "Variable")
.put("variableName", "options")
.build())
.build(), true, Collections.emptyList())
};
final Optional deleteOrders;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@Nullable DeleteOrders deleteOrders) {
this.deleteOrders = Optional.ofNullable(deleteOrders);
}
/**
* Delete mutation for the table 'Orders'.
* Note that 'prodName' and 'customerName' are the fields that correspond to the table primary key.
*/
public Optional getDeleteOrders() {
return this.deleteOrders;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], deleteOrders.isPresent() ? deleteOrders.get().marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "deleteOrders=" + deleteOrders
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return this.deleteOrders.equals(that.deleteOrders);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= deleteOrders.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public Builder toBuilder() {
Builder builder = new Builder();
builder.deleteOrders = deleteOrders.isPresent() ? deleteOrders.get() : null;
return builder;
}
public static Builder builder() {
return new Builder();
}
public static final class Mapper implements ResponseFieldMapper {
final DeleteOrders.Mapper deleteOrdersFieldMapper = new DeleteOrders.Mapper();
@Override
public Data map(ResponseReader reader) {
final DeleteOrders deleteOrders = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public DeleteOrders read(ResponseReader reader) {
return deleteOrdersFieldMapper.map(reader);
}
});
return new Data(deleteOrders);
}
}
public static final class Builder {
private @Nullable DeleteOrders deleteOrders;
Builder() {
}
public Builder deleteOrders(@Nullable DeleteOrders deleteOrders) {
this.deleteOrders = deleteOrders;
return this;
}
public Builder deleteOrders(@NotNull Mutator mutator) {
Utils.checkNotNull(mutator, "mutator == null");
DeleteOrders.Builder builder = this.deleteOrders != null ? this.deleteOrders.toBuilder() : DeleteOrders.builder();
mutator.accept(builder);
this.deleteOrders = builder.build();
return this;
}
public Data build() {
return new Data(deleteOrders);
}
}
}
/**
* The type used to represent results of a mutation for the table 'Orders'.
*/
public static class DeleteOrders {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forBoolean("applied", "applied", null, true, Collections.emptyList()),
ResponseField.forObject("value", "value", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final Optional applied;
final Optional value;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public DeleteOrders(@NotNull String __typename, @Nullable Boolean applied,
@Nullable Value value) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.applied = Optional.ofNullable(applied);
this.value = Optional.ofNullable(value);
}
public @NotNull String get__typename() {
return this.__typename;
}
public Optional getApplied() {
return this.applied;
}
public Optional getValue() {
return this.value;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeBoolean($responseFields[1], applied.isPresent() ? applied.get() : null);
writer.writeObject($responseFields[2], value.isPresent() ? value.get().marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "DeleteOrders{"
+ "__typename=" + __typename + ", "
+ "applied=" + applied + ", "
+ "value=" + value
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof DeleteOrders) {
DeleteOrders that = (DeleteOrders) o;
return this.__typename.equals(that.__typename)
&& this.applied.equals(that.applied)
&& this.value.equals(that.value);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= applied.hashCode();
h *= 1000003;
h ^= value.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public Builder toBuilder() {
Builder builder = new Builder();
builder.__typename = __typename;
builder.applied = applied.isPresent() ? applied.get() : null;
builder.value = value.isPresent() ? value.get() : null;
return builder;
}
public static Builder builder() {
return new Builder();
}
public static final class Mapper implements ResponseFieldMapper {
final Value.Mapper valueFieldMapper = new Value.Mapper();
@Override
public DeleteOrders map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Boolean applied = reader.readBoolean($responseFields[1]);
final Value value = reader.readObject($responseFields[2], new ResponseReader.ObjectReader() {
@Override
public Value read(ResponseReader reader) {
return valueFieldMapper.map(reader);
}
});
return new DeleteOrders(__typename, applied, value);
}
}
public static final class Builder {
private @NotNull String __typename;
private @Nullable Boolean applied;
private @Nullable Value value;
Builder() {
}
public Builder __typename(@NotNull String __typename) {
this.__typename = __typename;
return this;
}
public Builder applied(@Nullable Boolean applied) {
this.applied = applied;
return this;
}
public Builder value(@Nullable Value value) {
this.value = value;
return this;
}
public Builder value(@NotNull Mutator mutator) {
Utils.checkNotNull(mutator, "mutator == null");
Value.Builder builder = this.value != null ? this.value.toBuilder() : Value.builder();
mutator.accept(builder);
this.value = builder.build();
return this;
}
public DeleteOrders build() {
Utils.checkNotNull(__typename, "__typename == null");
return new DeleteOrders(__typename, applied, value);
}
}
}
/**
* The type used to represent results of a query for the table 'Orders'.
*/
public static class Value {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("id", "id", null, true, CustomType.UUID, Collections.emptyList()),
ResponseField.forCustomType("prodId", "prodId", null, true, CustomType.UUID, Collections.emptyList()),
ResponseField.forString("prodName", "prodName", null, true, Collections.emptyList()),
ResponseField.forString("customerName", "customerName", null, true, Collections.emptyList()),
ResponseField.forString("address", "address", null, true, Collections.emptyList()),
ResponseField.forString("description", "description", null, true, Collections.emptyList()),
ResponseField.forCustomType("price", "price", null, true, CustomType.DECIMAL, Collections.emptyList()),
ResponseField.forCustomType("sellPrice", "sellPrice", null, true, CustomType.DECIMAL, Collections.emptyList())
};
final @NotNull String __typename;
final Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy