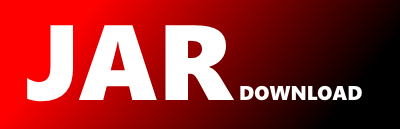
io.stargate.it.http.graphql.GraphqlClient Maven / Gradle / Ivy
/*
* Copyright The Stargate Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.stargate.it.http.graphql;
import static org.assertj.core.api.Assertions.assertThat;
import static org.junit.jupiter.api.Assertions.fail;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.stargate.it.http.RestUtils;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import okhttp3.Headers;
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import org.apache.http.HttpStatus;
/**
* A lightweight client for GraphQL tests.
*
* Queries are passed as plain strings, and results returned as raw JSON structures.
*/
public abstract class GraphqlClient {
private static final ObjectMapper OBJECT_MAPPER = new ObjectMapper();
protected Map getGraphqlData(String authToken, String url, String graphqlQuery) {
Map response =
getGraphqlResponse(
Collections.singletonMap("X-Cassandra-Token", authToken),
url,
graphqlQuery,
HttpStatus.SC_OK);
assertThat(response).isNotNull();
assertThat(response.get("errors")).isNull();
@SuppressWarnings("unchecked")
Map data = (Map) response.get("data");
return data;
}
protected String getGraphqlError(
String authToken, String url, String graphqlQuery, int expectedStatus) {
List