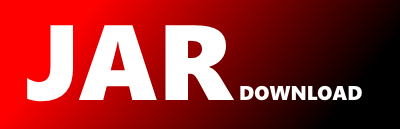
io.stargate.it.http.restapi.RestApiv2MVTest Maven / Gradle / Ivy
package io.stargate.it.http.restapi;
import static org.assertj.core.api.Assertions.assertThat;
import static org.assertj.core.api.Assumptions.assumeThat;
import com.datastax.oss.driver.api.core.CqlSession;
import com.datastax.oss.driver.api.core.cql.ResultSet;
import io.stargate.it.driver.CqlSessionExtension;
import io.stargate.it.driver.CqlSessionSpec;
import io.stargate.it.http.RestUtils;
import java.io.IOException;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import net.jcip.annotations.NotThreadSafe;
import org.apache.http.HttpStatus;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
/**
* Integration tests for REST API v2 that test Materialized View functionality. Separate from other
* tests to allow exclusion when testing backends that do not support MVs.
*/
@NotThreadSafe
@ExtendWith(CqlSessionExtension.class)
@CqlSessionSpec()
public class RestApiv2MVTest extends RestApiTestBase {
@Test
public void getAllRowsFromMaterializedView(CqlSession session) throws IOException {
assumeThat(isCassandra4())
.as("Disabled because MVs are not enabled by default on a Cassandra 4 backend")
.isFalse();
createTestKeyspace(keyspaceName);
tableName = "tbl_mvread_" + System.currentTimeMillis();
createTestTable(
tableName,
Arrays.asList("id text", "firstName text", "lastName text"),
Collections.singletonList("id"),
null);
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy