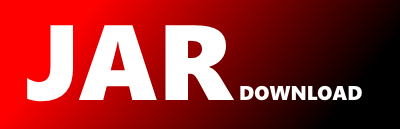
io.stargate.bridge.proto.MutinyStargateBridgeGrpc Maven / Gradle / Ivy
package io.stargate.bridge.proto;
import static io.stargate.bridge.proto.StargateBridgeGrpc.getServiceDescriptor;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
@jakarta.annotation.Generated(value = "by Mutiny Grpc generator", comments = "Source: bridge.proto")
public final class MutinyStargateBridgeGrpc implements io.quarkus.grpc.MutinyGrpc {
private MutinyStargateBridgeGrpc() {
}
public static MutinyStargateBridgeStub newMutinyStub(io.grpc.Channel channel) {
return new MutinyStargateBridgeStub(channel);
}
/**
*
* The gPRC API used by Stargate services to interact with the persistence backend.
*
*/
public static class MutinyStargateBridgeStub extends io.grpc.stub.AbstractStub implements io.quarkus.grpc.MutinyStub {
private StargateBridgeGrpc.StargateBridgeStub delegateStub;
private MutinyStargateBridgeStub(io.grpc.Channel channel) {
super(channel);
delegateStub = StargateBridgeGrpc.newStub(channel);
}
private MutinyStargateBridgeStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
delegateStub = StargateBridgeGrpc.newStub(channel).build(channel, callOptions);
}
@Override
protected MutinyStargateBridgeStub build(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new MutinyStargateBridgeStub(channel, callOptions);
}
/**
*
* Executes a single CQL query.
*
*/
public io.smallrye.mutiny.Uni executeQuery(io.stargate.bridge.proto.QueryOuterClass.Query request) {
return io.quarkus.grpc.stubs.ClientCalls.oneToOne(request, delegateStub::executeQuery);
}
/**
*
* Executes a single CQL query, assuming that a keyspace with the given version hash exists on the
* bridge side.
* This is an optimization when the client builds a query based on a keyspace's contents: with
* this operation, it can use its local version of the keyspace (therefore avoiding an extra
* network hop to fetch it), and execute the query optimistically. If the keyspace has changed,
* the bridge will reply with the new version, allowing the client to retry.
*
*/
public io.smallrye.mutiny.Uni executeQueryWithSchema(io.stargate.bridge.proto.Schema.QueryWithSchema request) {
return io.quarkus.grpc.stubs.ClientCalls.oneToOne(request, delegateStub::executeQueryWithSchema);
}
/**
*
* Executes a batch of CQL queries.
*
*/
public io.smallrye.mutiny.Uni executeBatch(io.stargate.bridge.proto.QueryOuterClass.Batch request) {
return io.quarkus.grpc.stubs.ClientCalls.oneToOne(request, delegateStub::executeBatch);
}
/**
*
* Similar to CQL "DESCRIBE KEYSPACE".
* Note that this operation does not perform any authorization check. The rationale is that, most
* of the time, client services use schema metadata to build another query that will be
* immediately executed with `ExecuteQuery` (which does check authorization).
* If that is not the case (e.g. you return the metadata directly to the client), you can check
* authorization explicitly with `AuthorizeSchemaReads`.
*
*/
public io.smallrye.mutiny.Uni describeKeyspace(io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery request) {
return io.quarkus.grpc.stubs.ClientCalls.oneToOne(request, delegateStub::describeKeyspace);
}
/**
*
* Checks whether the client is authorized to describe one or more schema elements.
*
*/
public io.smallrye.mutiny.Uni authorizeSchemaReads(io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest request) {
return io.quarkus.grpc.stubs.ClientCalls.oneToOne(request, delegateStub::authorizeSchemaReads);
}
/**
*
* Checks which features are supported by the persistence backend.
*
*/
public io.smallrye.mutiny.Uni getSupportedFeatures(io.stargate.bridge.proto.Schema.SupportedFeaturesRequest request) {
return io.quarkus.grpc.stubs.ClientCalls.oneToOne(request, delegateStub::getSupportedFeatures);
}
}
/**
*
* The gPRC API used by Stargate services to interact with the persistence backend.
*
*/
public static abstract class StargateBridgeImplBase implements io.grpc.BindableService {
private String compression;
/**
* Set whether the server will try to use a compressed response.
*
* @param compression the compression, e.g {@code gzip}
*/
public StargateBridgeImplBase withCompression(String compression) {
this.compression = compression;
return this;
}
/**
*
* Executes a single CQL query.
*
*/
public io.smallrye.mutiny.Uni executeQuery(io.stargate.bridge.proto.QueryOuterClass.Query request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
/**
*
* Executes a single CQL query, assuming that a keyspace with the given version hash exists on the
* bridge side.
* This is an optimization when the client builds a query based on a keyspace's contents: with
* this operation, it can use its local version of the keyspace (therefore avoiding an extra
* network hop to fetch it), and execute the query optimistically. If the keyspace has changed,
* the bridge will reply with the new version, allowing the client to retry.
*
*/
public io.smallrye.mutiny.Uni executeQueryWithSchema(io.stargate.bridge.proto.Schema.QueryWithSchema request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
/**
*
* Executes a batch of CQL queries.
*
*/
public io.smallrye.mutiny.Uni executeBatch(io.stargate.bridge.proto.QueryOuterClass.Batch request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
/**
*
* Similar to CQL "DESCRIBE KEYSPACE".
* Note that this operation does not perform any authorization check. The rationale is that, most
* of the time, client services use schema metadata to build another query that will be
* immediately executed with `ExecuteQuery` (which does check authorization).
* If that is not the case (e.g. you return the metadata directly to the client), you can check
* authorization explicitly with `AuthorizeSchemaReads`.
*
*/
public io.smallrye.mutiny.Uni describeKeyspace(io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
/**
*
* Checks whether the client is authorized to describe one or more schema elements.
*
*/
public io.smallrye.mutiny.Uni authorizeSchemaReads(io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
/**
*
* Checks which features are supported by the persistence backend.
*
*/
public io.smallrye.mutiny.Uni getSupportedFeatures(io.stargate.bridge.proto.Schema.SupportedFeaturesRequest request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
@java.lang.Override
public io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor()).addMethod(io.stargate.bridge.proto.StargateBridgeGrpc.getExecuteQueryMethod(), asyncUnaryCall(new MethodHandlers(this, METHODID_EXECUTE_QUERY, compression))).addMethod(io.stargate.bridge.proto.StargateBridgeGrpc.getExecuteQueryWithSchemaMethod(), asyncUnaryCall(new MethodHandlers(this, METHODID_EXECUTE_QUERY_WITH_SCHEMA, compression))).addMethod(io.stargate.bridge.proto.StargateBridgeGrpc.getExecuteBatchMethod(), asyncUnaryCall(new MethodHandlers(this, METHODID_EXECUTE_BATCH, compression))).addMethod(io.stargate.bridge.proto.StargateBridgeGrpc.getDescribeKeyspaceMethod(), asyncUnaryCall(new MethodHandlers(this, METHODID_DESCRIBE_KEYSPACE, compression))).addMethod(io.stargate.bridge.proto.StargateBridgeGrpc.getAuthorizeSchemaReadsMethod(), asyncUnaryCall(new MethodHandlers(this, METHODID_AUTHORIZE_SCHEMA_READS, compression))).addMethod(io.stargate.bridge.proto.StargateBridgeGrpc.getGetSupportedFeaturesMethod(), asyncUnaryCall(new MethodHandlers(this, METHODID_GET_SUPPORTED_FEATURES, compression))).build();
}
}
private static final int METHODID_EXECUTE_QUERY = 0;
private static final int METHODID_EXECUTE_QUERY_WITH_SCHEMA = 1;
private static final int METHODID_EXECUTE_BATCH = 2;
private static final int METHODID_DESCRIBE_KEYSPACE = 3;
private static final int METHODID_AUTHORIZE_SCHEMA_READS = 4;
private static final int METHODID_GET_SUPPORTED_FEATURES = 5;
private static final class MethodHandlers implements io.grpc.stub.ServerCalls.UnaryMethod, io.grpc.stub.ServerCalls.ServerStreamingMethod, io.grpc.stub.ServerCalls.ClientStreamingMethod, io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final StargateBridgeImplBase serviceImpl;
private final int methodId;
private final String compression;
MethodHandlers(StargateBridgeImplBase serviceImpl, int methodId, String compression) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
this.compression = compression;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch(methodId) {
case METHODID_EXECUTE_QUERY:
io.quarkus.grpc.stubs.ServerCalls.oneToOne((io.stargate.bridge.proto.QueryOuterClass.Query) request, (io.grpc.stub.StreamObserver) responseObserver, compression, serviceImpl::executeQuery);
break;
case METHODID_EXECUTE_QUERY_WITH_SCHEMA:
io.quarkus.grpc.stubs.ServerCalls.oneToOne((io.stargate.bridge.proto.Schema.QueryWithSchema) request, (io.grpc.stub.StreamObserver) responseObserver, compression, serviceImpl::executeQueryWithSchema);
break;
case METHODID_EXECUTE_BATCH:
io.quarkus.grpc.stubs.ServerCalls.oneToOne((io.stargate.bridge.proto.QueryOuterClass.Batch) request, (io.grpc.stub.StreamObserver) responseObserver, compression, serviceImpl::executeBatch);
break;
case METHODID_DESCRIBE_KEYSPACE:
io.quarkus.grpc.stubs.ServerCalls.oneToOne((io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery) request, (io.grpc.stub.StreamObserver) responseObserver, compression, serviceImpl::describeKeyspace);
break;
case METHODID_AUTHORIZE_SCHEMA_READS:
io.quarkus.grpc.stubs.ServerCalls.oneToOne((io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest) request, (io.grpc.stub.StreamObserver) responseObserver, compression, serviceImpl::authorizeSchemaReads);
break;
case METHODID_GET_SUPPORTED_FEATURES:
io.quarkus.grpc.stubs.ServerCalls.oneToOne((io.stargate.bridge.proto.Schema.SupportedFeaturesRequest) request, (io.grpc.stub.StreamObserver) responseObserver, compression, serviceImpl::getSupportedFeatures);
break;
default:
throw new java.lang.AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(io.grpc.stub.StreamObserver responseObserver) {
switch(methodId) {
default:
throw new java.lang.AssertionError();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy