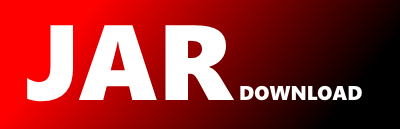
io.stargate.bridge.proto.StargateBridgeGrpc Maven / Gradle / Ivy
package io.stargate.bridge.proto;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* The gPRC API used by Stargate services to interact with the persistence backend.
*
*/
@io.quarkus.grpc.common.Generated(value = "by gRPC proto compiler (version 1.56.0)", comments = "Source: bridge.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class StargateBridgeGrpc {
private StargateBridgeGrpc() {
}
public static final String SERVICE_NAME = "stargate.StargateBridge";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getExecuteQueryMethod;
@io.grpc.stub.annotations.RpcMethod(fullMethodName = SERVICE_NAME + '/' + "ExecuteQuery", requestType = io.stargate.bridge.proto.QueryOuterClass.Query.class, responseType = io.stargate.bridge.proto.QueryOuterClass.Response.class, methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExecuteQueryMethod() {
io.grpc.MethodDescriptor getExecuteQueryMethod;
if ((getExecuteQueryMethod = StargateBridgeGrpc.getExecuteQueryMethod) == null) {
synchronized (StargateBridgeGrpc.class) {
if ((getExecuteQueryMethod = StargateBridgeGrpc.getExecuteQueryMethod) == null) {
StargateBridgeGrpc.getExecuteQueryMethod = getExecuteQueryMethod = io.grpc.MethodDescriptor.newBuilder().setType(io.grpc.MethodDescriptor.MethodType.UNARY).setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteQuery")).setSampledToLocalTracing(true).setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.QueryOuterClass.Query.getDefaultInstance())).setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.QueryOuterClass.Response.getDefaultInstance())).setSchemaDescriptor(new StargateBridgeMethodDescriptorSupplier("ExecuteQuery")).build();
}
}
}
return getExecuteQueryMethod;
}
private static volatile io.grpc.MethodDescriptor getExecuteQueryWithSchemaMethod;
@io.grpc.stub.annotations.RpcMethod(fullMethodName = SERVICE_NAME + '/' + "ExecuteQueryWithSchema", requestType = io.stargate.bridge.proto.Schema.QueryWithSchema.class, responseType = io.stargate.bridge.proto.Schema.QueryWithSchemaResponse.class, methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExecuteQueryWithSchemaMethod() {
io.grpc.MethodDescriptor getExecuteQueryWithSchemaMethod;
if ((getExecuteQueryWithSchemaMethod = StargateBridgeGrpc.getExecuteQueryWithSchemaMethod) == null) {
synchronized (StargateBridgeGrpc.class) {
if ((getExecuteQueryWithSchemaMethod = StargateBridgeGrpc.getExecuteQueryWithSchemaMethod) == null) {
StargateBridgeGrpc.getExecuteQueryWithSchemaMethod = getExecuteQueryWithSchemaMethod = io.grpc.MethodDescriptor.newBuilder().setType(io.grpc.MethodDescriptor.MethodType.UNARY).setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteQueryWithSchema")).setSampledToLocalTracing(true).setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.Schema.QueryWithSchema.getDefaultInstance())).setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.Schema.QueryWithSchemaResponse.getDefaultInstance())).setSchemaDescriptor(new StargateBridgeMethodDescriptorSupplier("ExecuteQueryWithSchema")).build();
}
}
}
return getExecuteQueryWithSchemaMethod;
}
private static volatile io.grpc.MethodDescriptor getExecuteBatchMethod;
@io.grpc.stub.annotations.RpcMethod(fullMethodName = SERVICE_NAME + '/' + "ExecuteBatch", requestType = io.stargate.bridge.proto.QueryOuterClass.Batch.class, responseType = io.stargate.bridge.proto.QueryOuterClass.Response.class, methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExecuteBatchMethod() {
io.grpc.MethodDescriptor getExecuteBatchMethod;
if ((getExecuteBatchMethod = StargateBridgeGrpc.getExecuteBatchMethod) == null) {
synchronized (StargateBridgeGrpc.class) {
if ((getExecuteBatchMethod = StargateBridgeGrpc.getExecuteBatchMethod) == null) {
StargateBridgeGrpc.getExecuteBatchMethod = getExecuteBatchMethod = io.grpc.MethodDescriptor.newBuilder().setType(io.grpc.MethodDescriptor.MethodType.UNARY).setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteBatch")).setSampledToLocalTracing(true).setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.QueryOuterClass.Batch.getDefaultInstance())).setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.QueryOuterClass.Response.getDefaultInstance())).setSchemaDescriptor(new StargateBridgeMethodDescriptorSupplier("ExecuteBatch")).build();
}
}
}
return getExecuteBatchMethod;
}
private static volatile io.grpc.MethodDescriptor getDescribeKeyspaceMethod;
@io.grpc.stub.annotations.RpcMethod(fullMethodName = SERVICE_NAME + '/' + "DescribeKeyspace", requestType = io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery.class, responseType = io.stargate.bridge.proto.Schema.CqlKeyspaceDescribe.class, methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDescribeKeyspaceMethod() {
io.grpc.MethodDescriptor getDescribeKeyspaceMethod;
if ((getDescribeKeyspaceMethod = StargateBridgeGrpc.getDescribeKeyspaceMethod) == null) {
synchronized (StargateBridgeGrpc.class) {
if ((getDescribeKeyspaceMethod = StargateBridgeGrpc.getDescribeKeyspaceMethod) == null) {
StargateBridgeGrpc.getDescribeKeyspaceMethod = getDescribeKeyspaceMethod = io.grpc.MethodDescriptor.newBuilder().setType(io.grpc.MethodDescriptor.MethodType.UNARY).setFullMethodName(generateFullMethodName(SERVICE_NAME, "DescribeKeyspace")).setSampledToLocalTracing(true).setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery.getDefaultInstance())).setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.Schema.CqlKeyspaceDescribe.getDefaultInstance())).setSchemaDescriptor(new StargateBridgeMethodDescriptorSupplier("DescribeKeyspace")).build();
}
}
}
return getDescribeKeyspaceMethod;
}
private static volatile io.grpc.MethodDescriptor getAuthorizeSchemaReadsMethod;
@io.grpc.stub.annotations.RpcMethod(fullMethodName = SERVICE_NAME + '/' + "AuthorizeSchemaReads", requestType = io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest.class, responseType = io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsResponse.class, methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAuthorizeSchemaReadsMethod() {
io.grpc.MethodDescriptor getAuthorizeSchemaReadsMethod;
if ((getAuthorizeSchemaReadsMethod = StargateBridgeGrpc.getAuthorizeSchemaReadsMethod) == null) {
synchronized (StargateBridgeGrpc.class) {
if ((getAuthorizeSchemaReadsMethod = StargateBridgeGrpc.getAuthorizeSchemaReadsMethod) == null) {
StargateBridgeGrpc.getAuthorizeSchemaReadsMethod = getAuthorizeSchemaReadsMethod = io.grpc.MethodDescriptor.newBuilder().setType(io.grpc.MethodDescriptor.MethodType.UNARY).setFullMethodName(generateFullMethodName(SERVICE_NAME, "AuthorizeSchemaReads")).setSampledToLocalTracing(true).setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest.getDefaultInstance())).setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsResponse.getDefaultInstance())).setSchemaDescriptor(new StargateBridgeMethodDescriptorSupplier("AuthorizeSchemaReads")).build();
}
}
}
return getAuthorizeSchemaReadsMethod;
}
private static volatile io.grpc.MethodDescriptor getGetSupportedFeaturesMethod;
@io.grpc.stub.annotations.RpcMethod(fullMethodName = SERVICE_NAME + '/' + "GetSupportedFeatures", requestType = io.stargate.bridge.proto.Schema.SupportedFeaturesRequest.class, responseType = io.stargate.bridge.proto.Schema.SupportedFeaturesResponse.class, methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetSupportedFeaturesMethod() {
io.grpc.MethodDescriptor getGetSupportedFeaturesMethod;
if ((getGetSupportedFeaturesMethod = StargateBridgeGrpc.getGetSupportedFeaturesMethod) == null) {
synchronized (StargateBridgeGrpc.class) {
if ((getGetSupportedFeaturesMethod = StargateBridgeGrpc.getGetSupportedFeaturesMethod) == null) {
StargateBridgeGrpc.getGetSupportedFeaturesMethod = getGetSupportedFeaturesMethod = io.grpc.MethodDescriptor.newBuilder().setType(io.grpc.MethodDescriptor.MethodType.UNARY).setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetSupportedFeatures")).setSampledToLocalTracing(true).setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.Schema.SupportedFeaturesRequest.getDefaultInstance())).setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(io.stargate.bridge.proto.Schema.SupportedFeaturesResponse.getDefaultInstance())).setSchemaDescriptor(new StargateBridgeMethodDescriptorSupplier("GetSupportedFeatures")).build();
}
}
}
return getGetSupportedFeaturesMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static StargateBridgeStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory = new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public StargateBridgeStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateBridgeStub(channel, callOptions);
}
};
return StargateBridgeStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static StargateBridgeBlockingStub newBlockingStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory = new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public StargateBridgeBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateBridgeBlockingStub(channel, callOptions);
}
};
return StargateBridgeBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static StargateBridgeFutureStub newFutureStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory = new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public StargateBridgeFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateBridgeFutureStub(channel, callOptions);
}
};
return StargateBridgeFutureStub.newStub(factory, channel);
}
/**
*
* The gPRC API used by Stargate services to interact with the persistence backend.
*
*/
public interface AsyncService {
/**
*
* Executes a single CQL query.
*
*/
default void executeQuery(io.stargate.bridge.proto.QueryOuterClass.Query request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteQueryMethod(), responseObserver);
}
/**
*
* Executes a single CQL query, assuming that a keyspace with the given version hash exists on the
* bridge side.
* This is an optimization when the client builds a query based on a keyspace's contents: with
* this operation, it can use its local version of the keyspace (therefore avoiding an extra
* network hop to fetch it), and execute the query optimistically. If the keyspace has changed,
* the bridge will reply with the new version, allowing the client to retry.
*
*/
default void executeQueryWithSchema(io.stargate.bridge.proto.Schema.QueryWithSchema request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteQueryWithSchemaMethod(), responseObserver);
}
/**
*
* Executes a batch of CQL queries.
*
*/
default void executeBatch(io.stargate.bridge.proto.QueryOuterClass.Batch request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteBatchMethod(), responseObserver);
}
/**
*
* Similar to CQL "DESCRIBE KEYSPACE".
* Note that this operation does not perform any authorization check. The rationale is that, most
* of the time, client services use schema metadata to build another query that will be
* immediately executed with `ExecuteQuery` (which does check authorization).
* If that is not the case (e.g. you return the metadata directly to the client), you can check
* authorization explicitly with `AuthorizeSchemaReads`.
*
*/
default void describeKeyspace(io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDescribeKeyspaceMethod(), responseObserver);
}
/**
*
* Checks whether the client is authorized to describe one or more schema elements.
*
*/
default void authorizeSchemaReads(io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAuthorizeSchemaReadsMethod(), responseObserver);
}
/**
*
* Checks which features are supported by the persistence backend.
*
*/
default void getSupportedFeatures(io.stargate.bridge.proto.Schema.SupportedFeaturesRequest request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetSupportedFeaturesMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service StargateBridge.
*
* The gPRC API used by Stargate services to interact with the persistence backend.
*
*/
public static abstract class StargateBridgeImplBase implements io.grpc.BindableService, AsyncService {
@java.lang.Override
public io.grpc.ServerServiceDefinition bindService() {
return StargateBridgeGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service StargateBridge.
*
* The gPRC API used by Stargate services to interact with the persistence backend.
*
*/
public static class StargateBridgeStub extends io.grpc.stub.AbstractAsyncStub {
private StargateBridgeStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected StargateBridgeStub build(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateBridgeStub(channel, callOptions);
}
/**
*
* Executes a single CQL query.
*
*/
public void executeQuery(io.stargate.bridge.proto.QueryOuterClass.Query request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(getChannel().newCall(getExecuteQueryMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Executes a single CQL query, assuming that a keyspace with the given version hash exists on the
* bridge side.
* This is an optimization when the client builds a query based on a keyspace's contents: with
* this operation, it can use its local version of the keyspace (therefore avoiding an extra
* network hop to fetch it), and execute the query optimistically. If the keyspace has changed,
* the bridge will reply with the new version, allowing the client to retry.
*
*/
public void executeQueryWithSchema(io.stargate.bridge.proto.Schema.QueryWithSchema request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(getChannel().newCall(getExecuteQueryWithSchemaMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Executes a batch of CQL queries.
*
*/
public void executeBatch(io.stargate.bridge.proto.QueryOuterClass.Batch request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(getChannel().newCall(getExecuteBatchMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Similar to CQL "DESCRIBE KEYSPACE".
* Note that this operation does not perform any authorization check. The rationale is that, most
* of the time, client services use schema metadata to build another query that will be
* immediately executed with `ExecuteQuery` (which does check authorization).
* If that is not the case (e.g. you return the metadata directly to the client), you can check
* authorization explicitly with `AuthorizeSchemaReads`.
*
*/
public void describeKeyspace(io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(getChannel().newCall(getDescribeKeyspaceMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Checks whether the client is authorized to describe one or more schema elements.
*
*/
public void authorizeSchemaReads(io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(getChannel().newCall(getAuthorizeSchemaReadsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Checks which features are supported by the persistence backend.
*
*/
public void getSupportedFeatures(io.stargate.bridge.proto.Schema.SupportedFeaturesRequest request, io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(getChannel().newCall(getGetSupportedFeaturesMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service StargateBridge.
*
* The gPRC API used by Stargate services to interact with the persistence backend.
*
*/
public static class StargateBridgeBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private StargateBridgeBlockingStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected StargateBridgeBlockingStub build(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateBridgeBlockingStub(channel, callOptions);
}
/**
*
* Executes a single CQL query.
*
*/
public io.stargate.bridge.proto.QueryOuterClass.Response executeQuery(io.stargate.bridge.proto.QueryOuterClass.Query request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(getChannel(), getExecuteQueryMethod(), getCallOptions(), request);
}
/**
*
* Executes a single CQL query, assuming that a keyspace with the given version hash exists on the
* bridge side.
* This is an optimization when the client builds a query based on a keyspace's contents: with
* this operation, it can use its local version of the keyspace (therefore avoiding an extra
* network hop to fetch it), and execute the query optimistically. If the keyspace has changed,
* the bridge will reply with the new version, allowing the client to retry.
*
*/
public io.stargate.bridge.proto.Schema.QueryWithSchemaResponse executeQueryWithSchema(io.stargate.bridge.proto.Schema.QueryWithSchema request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(getChannel(), getExecuteQueryWithSchemaMethod(), getCallOptions(), request);
}
/**
*
* Executes a batch of CQL queries.
*
*/
public io.stargate.bridge.proto.QueryOuterClass.Response executeBatch(io.stargate.bridge.proto.QueryOuterClass.Batch request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(getChannel(), getExecuteBatchMethod(), getCallOptions(), request);
}
/**
*
* Similar to CQL "DESCRIBE KEYSPACE".
* Note that this operation does not perform any authorization check. The rationale is that, most
* of the time, client services use schema metadata to build another query that will be
* immediately executed with `ExecuteQuery` (which does check authorization).
* If that is not the case (e.g. you return the metadata directly to the client), you can check
* authorization explicitly with `AuthorizeSchemaReads`.
*
*/
public io.stargate.bridge.proto.Schema.CqlKeyspaceDescribe describeKeyspace(io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(getChannel(), getDescribeKeyspaceMethod(), getCallOptions(), request);
}
/**
*
* Checks whether the client is authorized to describe one or more schema elements.
*
*/
public io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsResponse authorizeSchemaReads(io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(getChannel(), getAuthorizeSchemaReadsMethod(), getCallOptions(), request);
}
/**
*
* Checks which features are supported by the persistence backend.
*
*/
public io.stargate.bridge.proto.Schema.SupportedFeaturesResponse getSupportedFeatures(io.stargate.bridge.proto.Schema.SupportedFeaturesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(getChannel(), getGetSupportedFeaturesMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service StargateBridge.
*
* The gPRC API used by Stargate services to interact with the persistence backend.
*
*/
public static class StargateBridgeFutureStub extends io.grpc.stub.AbstractFutureStub {
private StargateBridgeFutureStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected StargateBridgeFutureStub build(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new StargateBridgeFutureStub(channel, callOptions);
}
/**
*
* Executes a single CQL query.
*
*/
public com.google.common.util.concurrent.ListenableFuture executeQuery(io.stargate.bridge.proto.QueryOuterClass.Query request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(getChannel().newCall(getExecuteQueryMethod(), getCallOptions()), request);
}
/**
*
* Executes a single CQL query, assuming that a keyspace with the given version hash exists on the
* bridge side.
* This is an optimization when the client builds a query based on a keyspace's contents: with
* this operation, it can use its local version of the keyspace (therefore avoiding an extra
* network hop to fetch it), and execute the query optimistically. If the keyspace has changed,
* the bridge will reply with the new version, allowing the client to retry.
*
*/
public com.google.common.util.concurrent.ListenableFuture executeQueryWithSchema(io.stargate.bridge.proto.Schema.QueryWithSchema request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(getChannel().newCall(getExecuteQueryWithSchemaMethod(), getCallOptions()), request);
}
/**
*
* Executes a batch of CQL queries.
*
*/
public com.google.common.util.concurrent.ListenableFuture executeBatch(io.stargate.bridge.proto.QueryOuterClass.Batch request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(getChannel().newCall(getExecuteBatchMethod(), getCallOptions()), request);
}
/**
*
* Similar to CQL "DESCRIBE KEYSPACE".
* Note that this operation does not perform any authorization check. The rationale is that, most
* of the time, client services use schema metadata to build another query that will be
* immediately executed with `ExecuteQuery` (which does check authorization).
* If that is not the case (e.g. you return the metadata directly to the client), you can check
* authorization explicitly with `AuthorizeSchemaReads`.
*
*/
public com.google.common.util.concurrent.ListenableFuture describeKeyspace(io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(getChannel().newCall(getDescribeKeyspaceMethod(), getCallOptions()), request);
}
/**
*
* Checks whether the client is authorized to describe one or more schema elements.
*
*/
public com.google.common.util.concurrent.ListenableFuture authorizeSchemaReads(io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(getChannel().newCall(getAuthorizeSchemaReadsMethod(), getCallOptions()), request);
}
/**
*
* Checks which features are supported by the persistence backend.
*
*/
public com.google.common.util.concurrent.ListenableFuture getSupportedFeatures(io.stargate.bridge.proto.Schema.SupportedFeaturesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(getChannel().newCall(getGetSupportedFeaturesMethod(), getCallOptions()), request);
}
}
private static final int METHODID_EXECUTE_QUERY = 0;
private static final int METHODID_EXECUTE_QUERY_WITH_SCHEMA = 1;
private static final int METHODID_EXECUTE_BATCH = 2;
private static final int METHODID_DESCRIBE_KEYSPACE = 3;
private static final int METHODID_AUTHORIZE_SCHEMA_READS = 4;
private static final int METHODID_GET_SUPPORTED_FEATURES = 5;
private static final class MethodHandlers implements io.grpc.stub.ServerCalls.UnaryMethod, io.grpc.stub.ServerCalls.ServerStreamingMethod, io.grpc.stub.ServerCalls.ClientStreamingMethod, io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch(methodId) {
case METHODID_EXECUTE_QUERY:
serviceImpl.executeQuery((io.stargate.bridge.proto.QueryOuterClass.Query) request, (io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_QUERY_WITH_SCHEMA:
serviceImpl.executeQueryWithSchema((io.stargate.bridge.proto.Schema.QueryWithSchema) request, (io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_BATCH:
serviceImpl.executeBatch((io.stargate.bridge.proto.QueryOuterClass.Batch) request, (io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DESCRIBE_KEYSPACE:
serviceImpl.describeKeyspace((io.stargate.bridge.proto.Schema.DescribeKeyspaceQuery) request, (io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_AUTHORIZE_SCHEMA_READS:
serviceImpl.authorizeSchemaReads((io.stargate.bridge.proto.Schema.AuthorizeSchemaReadsRequest) request, (io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_SUPPORTED_FEATURES:
serviceImpl.getSupportedFeatures((io.stargate.bridge.proto.Schema.SupportedFeaturesRequest) request, (io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(io.grpc.stub.StreamObserver responseObserver) {
switch(methodId) {
default:
throw new AssertionError();
}
}
}
public static io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor()).addMethod(getExecuteQueryMethod(), io.grpc.stub.ServerCalls.asyncUnaryCall(new MethodHandlers(service, METHODID_EXECUTE_QUERY))).addMethod(getExecuteQueryWithSchemaMethod(), io.grpc.stub.ServerCalls.asyncUnaryCall(new MethodHandlers(service, METHODID_EXECUTE_QUERY_WITH_SCHEMA))).addMethod(getExecuteBatchMethod(), io.grpc.stub.ServerCalls.asyncUnaryCall(new MethodHandlers(service, METHODID_EXECUTE_BATCH))).addMethod(getDescribeKeyspaceMethod(), io.grpc.stub.ServerCalls.asyncUnaryCall(new MethodHandlers(service, METHODID_DESCRIBE_KEYSPACE))).addMethod(getAuthorizeSchemaReadsMethod(), io.grpc.stub.ServerCalls.asyncUnaryCall(new MethodHandlers(service, METHODID_AUTHORIZE_SCHEMA_READS))).addMethod(getGetSupportedFeaturesMethod(), io.grpc.stub.ServerCalls.asyncUnaryCall(new MethodHandlers(service, METHODID_GET_SUPPORTED_FEATURES))).build();
}
private static abstract class StargateBridgeBaseDescriptorSupplier implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
StargateBridgeBaseDescriptorSupplier() {
}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return io.stargate.bridge.proto.Bridge.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("StargateBridge");
}
}
private static final class StargateBridgeFileDescriptorSupplier extends StargateBridgeBaseDescriptorSupplier {
StargateBridgeFileDescriptorSupplier() {
}
}
private static final class StargateBridgeMethodDescriptorSupplier extends StargateBridgeBaseDescriptorSupplier implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
StargateBridgeMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (StargateBridgeGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME).setSchemaDescriptor(new StargateBridgeFileDescriptorSupplier()).addMethod(getExecuteQueryMethod()).addMethod(getExecuteQueryWithSchemaMethod()).addMethod(getExecuteBatchMethod()).addMethod(getDescribeKeyspaceMethod()).addMethod(getAuthorizeSchemaReadsMethod()).addMethod(getGetSupportedFeaturesMethod()).build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy