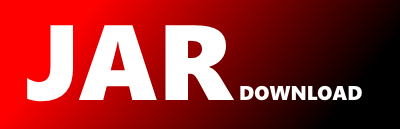
io.stargate.sgv2.api.common.grpc.StargateBridgeClientImpl Maven / Gradle / Ivy
/*
* Copyright The Stargate Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package io.stargate.sgv2.api.common.grpc;
import io.smallrye.mutiny.Uni;
import io.stargate.bridge.proto.QueryOuterClass;
import io.stargate.bridge.proto.Schema;
import io.stargate.sgv2.api.common.StargateRequestInfo;
import io.stargate.sgv2.api.common.schema.SchemaManager;
import jakarta.enterprise.context.ApplicationScoped;
import jakarta.inject.Inject;
import java.nio.charset.StandardCharsets;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletionStage;
import java.util.function.Function;
import org.apache.commons.codec.binary.Hex;
@ApplicationScoped
public class StargateBridgeClientImpl implements StargateBridgeClient {
private static final Function>
MISSING_KEYSPACE = ks -> Uni.createFrom().nullItem();
@Inject SchemaManager schemaManager;
@Inject StargateRequestInfo requestInfo;
@Override
public CompletionStage executeQueryAsync(QueryOuterClass.Query query) {
return requestInfo.getStargateBridge().executeQuery(query).subscribeAsCompletionStage();
}
@Override
public CompletionStage executeQueryAsync(
String keyspaceName,
String tableName,
Function, QueryOuterClass.Query> queryProducer) {
// TODO implement optimistic queries (probably requires changes directly in SchemaManager)
return getTableAsync(keyspaceName, tableName, true)
.thenCompose(table -> executeQueryAsync(queryProducer.apply(table)));
}
@Override
public CompletionStage executeBatchAsync(QueryOuterClass.Batch batch) {
return requestInfo.getStargateBridge().executeBatch(batch).subscribeAsCompletionStage();
}
@Override
public CompletionStage> getKeyspaceAsync(
String keyspaceName, boolean checkIfAuthorized) {
Uni keyspace =
checkIfAuthorized
? schemaManager.getKeyspaceAuthorized(keyspaceName)
: schemaManager.getKeyspace(keyspaceName);
return keyspace.map(Optional::ofNullable).subscribeAsCompletionStage();
}
@Override
public CompletionStage> getAllKeyspacesAsync() {
return schemaManager.getKeyspaces().collect().asList().subscribeAsCompletionStage();
}
@Override
public String decorateKeyspaceName(String keyspaceName) {
return requestInfo
.getTenantId()
.map(
tenantId ->
Hex.encodeHexString(tenantId.getBytes(StandardCharsets.UTF_8)) + "_" + keyspaceName)
.orElse(keyspaceName);
}
@Override
public CompletionStage> getTableAsync(
String keyspaceName, String tableName, boolean checkIfAuthorized) {
Uni table =
checkIfAuthorized
? schemaManager.getTableAuthorized(keyspaceName, tableName, MISSING_KEYSPACE)
: schemaManager.getTable(keyspaceName, tableName, MISSING_KEYSPACE);
return table.map(Optional::ofNullable).subscribeAsCompletionStage();
}
@Override
public CompletionStage> getTablesAsync(String keyspaceName) {
return schemaManager
.getTables(keyspaceName, MISSING_KEYSPACE)
.collect()
.asList()
.subscribeAsCompletionStage();
}
@Override
public CompletionStage> authorizeSchemaReadsAsync(
List schemaReads) {
return requestInfo
.getStargateBridge()
.authorizeSchemaReads(
Schema.AuthorizeSchemaReadsRequest.newBuilder().addAllSchemaReads(schemaReads).build())
.map(Schema.AuthorizeSchemaReadsResponse::getAuthorizedList)
.subscribeAsCompletionStage();
}
@Override
public CompletionStage getSupportedFeaturesAsync() {
return requestInfo
.getStargateBridge()
.getSupportedFeatures(Schema.SupportedFeaturesRequest.getDefaultInstance())
.subscribeAsCompletionStage();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy